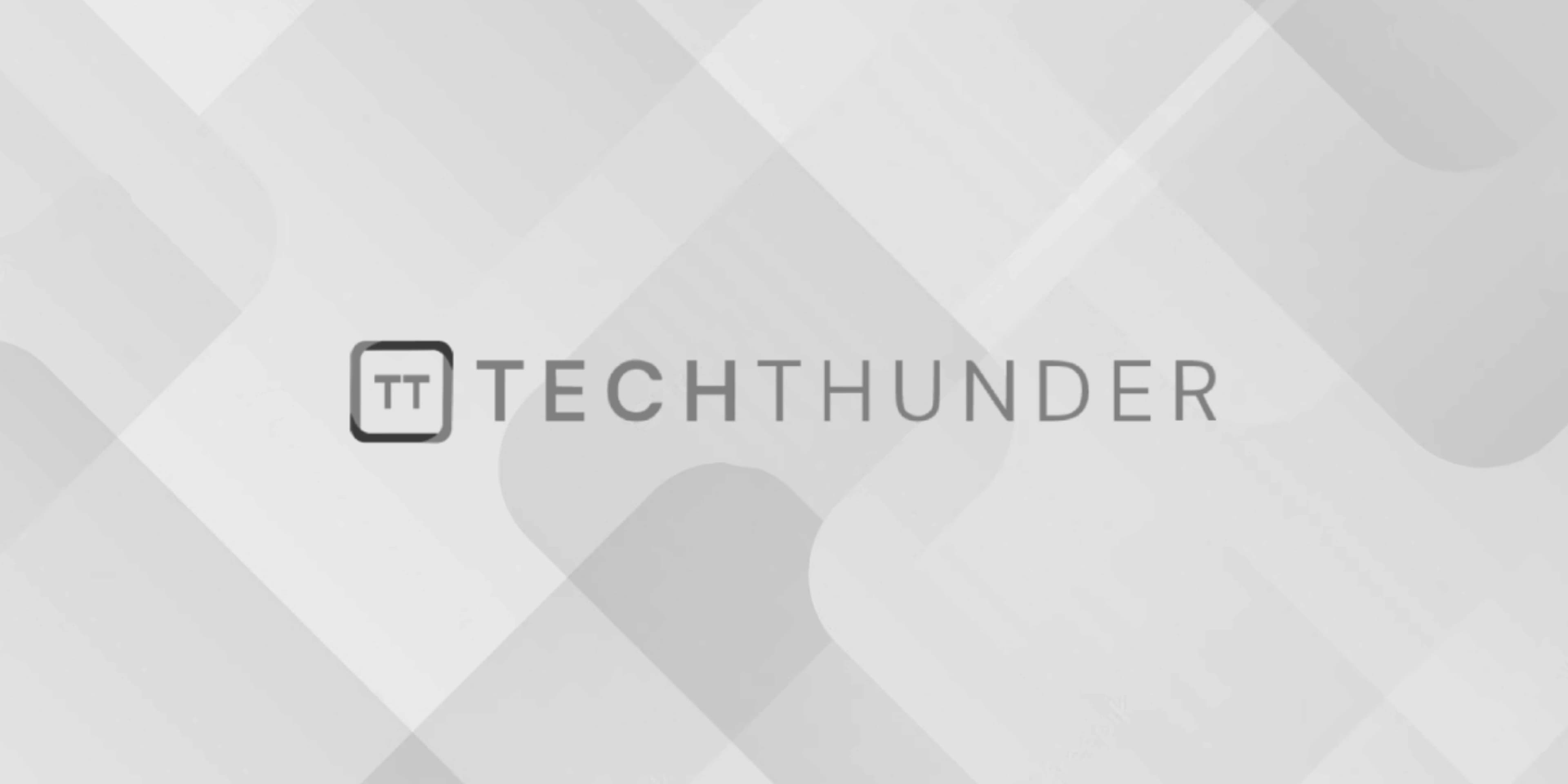
223 views
Implement Flash Message with Laravel 5.7
To implement flash messages in Laravel 5.7, you can follow these steps:
- Create a Flash Messages Blade Partial: First, create a Blade partial that will render the flash messages. Create a new file, let’s say
flash-messages.blade.php
, in theresources/views
directory if it doesn’t already exist. This file will contain the HTML structure for displaying flash messages.
PHP
@if(session('success'))
<div class="alert alert-success">
{{ session('success') }}
</div>
@endif
@if(session('error'))
<div class="alert alert-danger">
{{ session('error') }}
</div>
@endif
- Display the Flash Messages in Your Layout File: In your layout Blade file (e.g.,
resources/views/layouts/app.blade.php
), include the flash messages partial where you want to display the messages, typically near the top of the layout.
PHP
@include('flash-messages')
- Set Flash Messages in Your Controller: To set flash messages in your controller, you can use the
session
method, which is available out of the box in Laravel. Here’s an example:
PHP
public function store(Request $request)
{
// Your logic to store data
// Success flash message
session()->flash('success', 'Data has been successfully saved.');
// Redirect back or to another page
return redirect()->route('your.route.name');
}
public function delete(Request $request)
{
// Your logic to delete data
// Error flash message
session()->flash('error', 'Data could not be deleted.');
// Redirect back or to another page
return redirect()->route('your.route.name');
}
Replace 'success'
and 'error'
with your own keys for different types of flash messages, and customize the message content accordingly.
- Styling (Optional): You can add CSS styles to your flash messages to make them visually appealing. Laravel provides a default Bootstrap-based styling, but you can customize it to match your application’s design.
That’s it! You’ve now implemented flash messages in your Laravel 5.7 application. Flash messages are a convenient way to provide feedback to users about the success or failure of certain actions in your application.