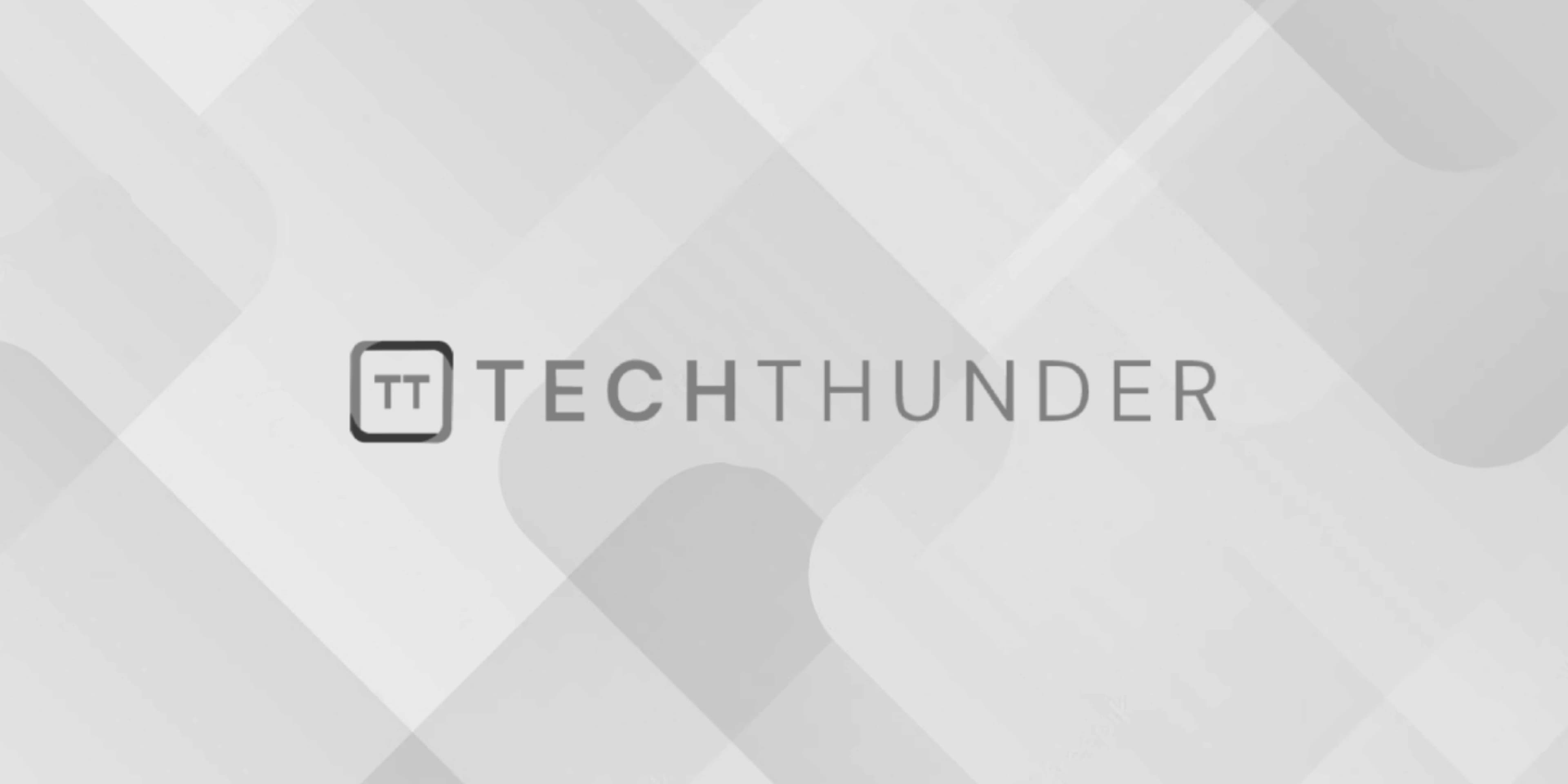
Laravel Relationship
The laravel relationships allow you to define how different database tables or models are related to each other. Laravel provides an elegant and expressive way to define and work with relationships between models. There are several types of relationships supported by Laravel Eloquent:
1. One-to-One Relationship:
In a one-to-one relationship, one record in a table is associated with one record in another table. For example, a “User” has one “Profile” record:
// User Model
public function profile()
{
return $this->hasOne(Profile::class);
}
// Profile Model
public function user()
{
return $this->belongsTo(User::class);
}
2. One-to-Many Relationship:
In a one-to-many relationship, one record in a table is associated with multiple records in another table. For example, a “User” can have many “Posts”:
// User Model
public function posts()
{
return $this->hasMany(Post::class);
}
// Post Model
public function user()
{
return $this->belongsTo(User::class);
}
3. Many-to-One Relationship:
A many-to-one relationship is essentially the reverse of a one-to-many relationship. Many records in one table are associated with one record in another table. For example, many “Comments” belong to one “Post”:
// Comment Model
public function post()
{
return $this->belongsTo(Post::class);
}
// Post Model
public function comments()
{
return $this->hasMany(Comment::class);
}
4. Many-to-Many Relationship:
In a many-to-many relationship, multiple records in one table are associated with multiple records in another table. For example, “User” can have many “Roles,” and a “Role” can belong to many “Users.” You’ll need an intermediate pivot table to define this relationship:
// User Model
public function roles()
{
return $this->belongsToMany(Role::class);
}
// Role Model
public function users()
{
return $this->belongsToMany(User::class);
}
5. Polymorphic Relationship:
A polymorphic relationship allows a model to belong to multiple other types of models on a single association. For example, a “Comment” can belong to both a “Post” and a “Video”:
// Comment Model
public function commentable()
{
return $this->morphTo();
}
// Post Model
public function comments()
{
return $this->morphMany(Comment::class, 'commentable');
}
// Video Model
public function comments()
{
return $this->morphMany(Comment::class, 'commentable');
}
These are some of the most common types of relationships in Laravel. Defining and working with relationships in Eloquent is an essential aspect of building robust and maintainable Laravel applications. It allows you to retrieve related data easily and efficiently, making it a key feature of the Laravel framework for database interactions.