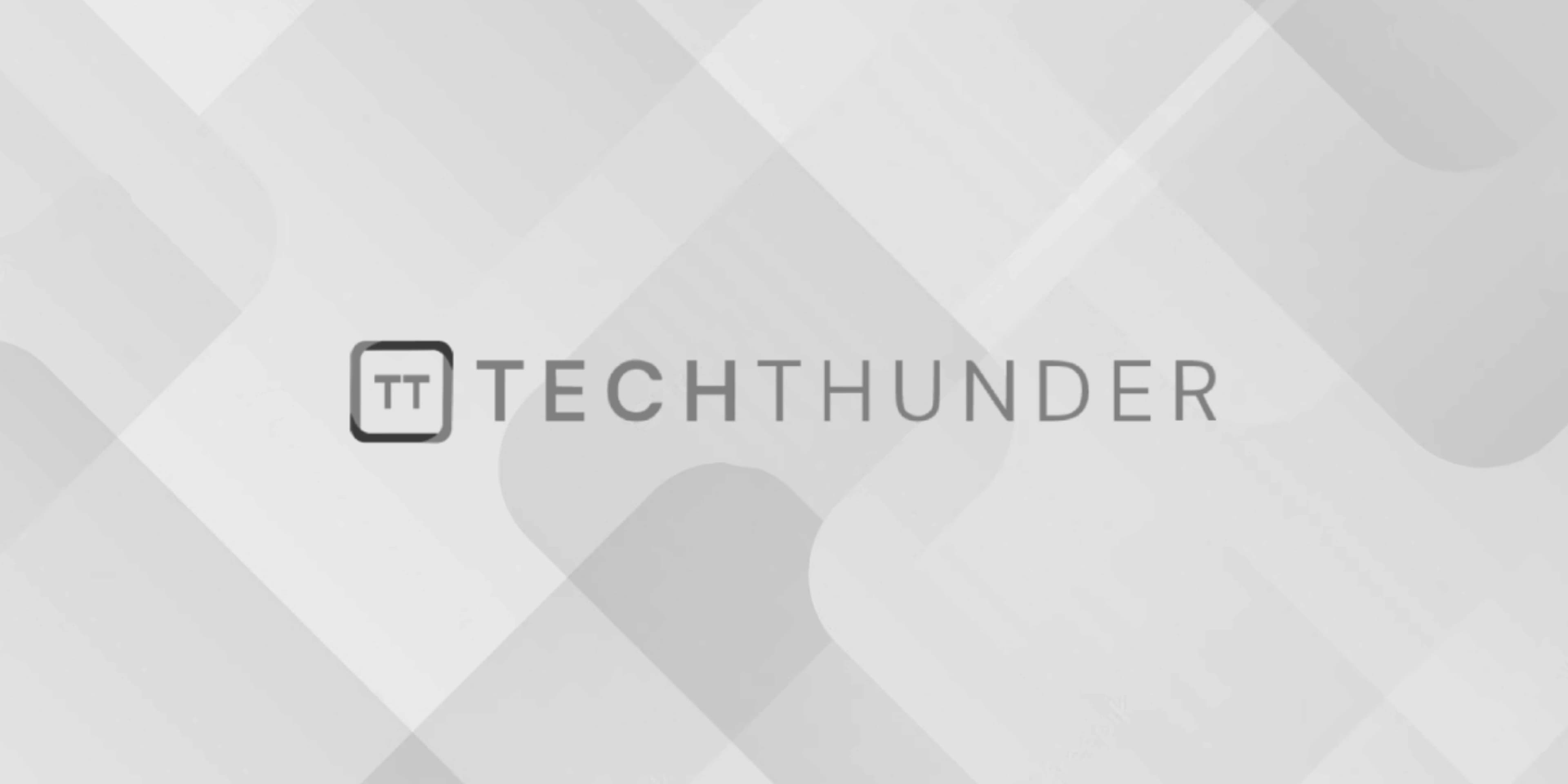
MongoDB CRUD in Laravel
Performing CRUD operations (Create, Read, Update, Delete) with MongoDB in Laravel involves using the MongoDB Eloquent driver, which provides an abstraction layer to work with MongoDB collections as if they were regular Eloquent models. Here’s a guide on how to perform CRUD operations with MongoDB in Laravel:
- Install MongoDB and Configure Laravel: Make sure you have MongoDB installed and running. Additionally, install the
jenssegers/mongodb
package, which provides the MongoDB Eloquent driver, using Composer:
composer require jenssegers/mongodb
Then, configure your MongoDB connection in config/database.php
:
'connections' => [
'mongodb' => [
'driver' => 'mongodb',
'host' => env('DB_HOST', '127.0.0.1'),
'port' => env('DB_PORT', 27017),
'database' => env('DB_DATABASE'),
'username' => env('DB_USERNAME'),
'password' => env('DB_PASSWORD'),
// ...
],
],
- Create a MongoDB Model: Create a MongoDB model using the
make:model
Artisan command:
php artisan make:model Book --all
This will generate a model, migration, factory, and controller for the “Book” entity.
- Define the Model and Schema: In your generated
app/Models/Book.php
model file, specify the MongoDB connection and schema:
namespace App\Models;
use Jenssegers\Mongodb\Eloquent\Model;
class Book extends Model
{
protected $connection = 'mongodb';
protected $fillable = ['title', 'author', 'price'];
}
- Create CRUD Methods in Controller: In your generated
app/Http/Controllers/BookController.php
controller, define the CRUD methods for your MongoDB model:
namespace App\Http\Controllers;
use App\Models\Book;
use Illuminate\Http\Request;
class BookController extends Controller
{
public function index()
{
$books = Book::all();
return view('books.index', compact('books'));
}
public function create()
{
return view('books.create');
}
public function store(Request $request)
{
Book::create($request->all());
return redirect()->route('books.index');
}
public function edit($id)
{
$book = Book::find($id);
return view('books.edit', compact('book'));
}
public function update(Request $request, $id)
{
$book = Book::find($id);
$book->update($request->all());
return redirect()->route('books.index');
}
public function destroy($id)
{
$book = Book::find($id);
$book->delete();
return redirect()->route('books.index');
}
}
- Create Views: Create Blade views for listing, creating, editing, and viewing details of books in the
resources/views/books
directory. - Define Routes: Define routes in
routes/web.php
for your CRUD operations:
use App\Http\Controllers\BookController;
Route::resource('books', BookController::class);
- Run Migrations and Start the Server: Run migrations to create the
books
collection in your MongoDB database:
php artisan migrate
Start the development server:
php artisan serve
You now have a basic setup for performing CRUD operations with MongoDB using Laravel’s Eloquent driver for MongoDB. You can customize and expand on this setup according to your application’s requirements. Remember to check the official documentation for the Jenssegers MongoDB package and Laravel Eloquent for more advanced features and options.