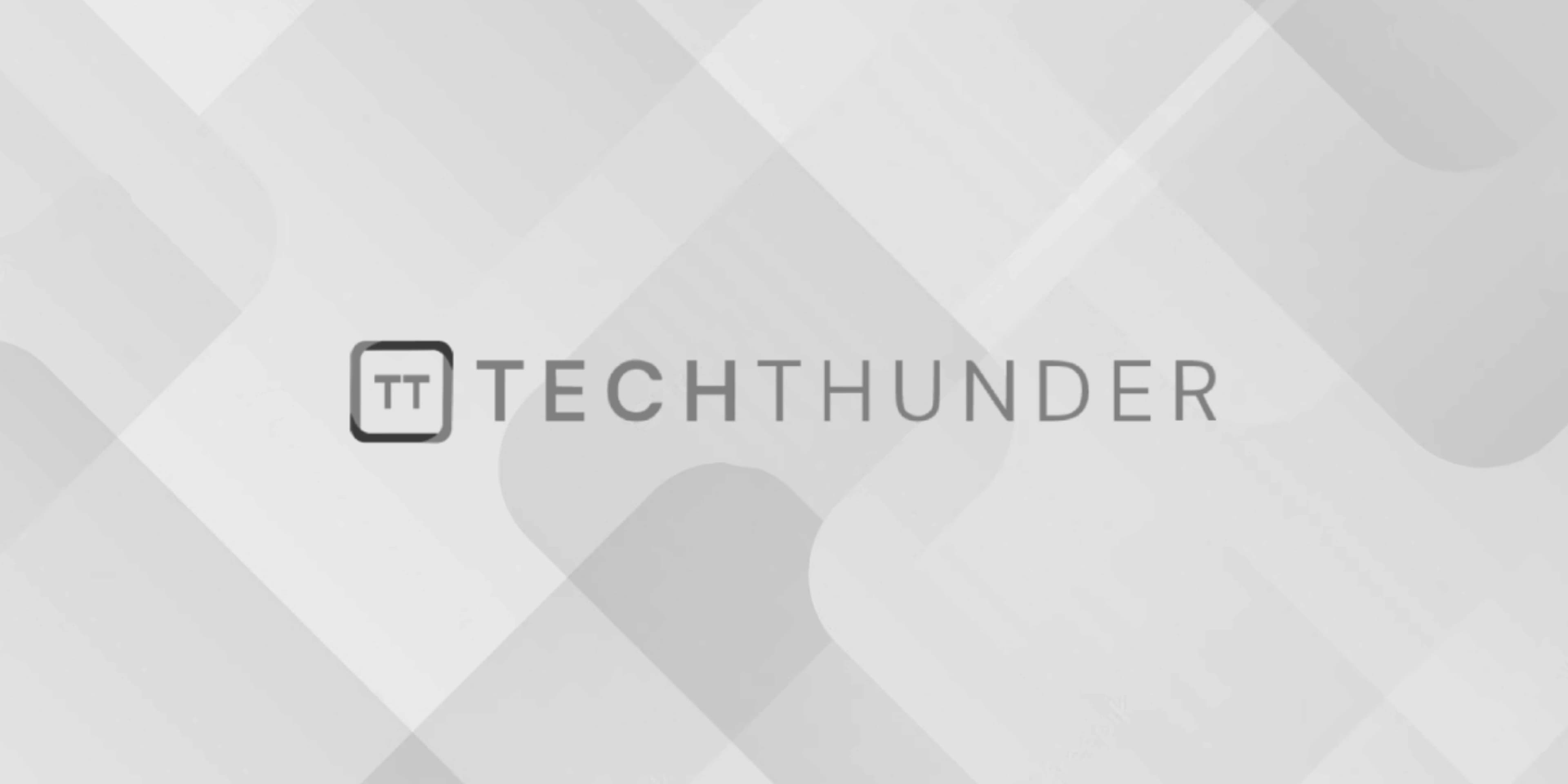
Laravel notification alert using Bootstrap notify plugin
Integrating Laravel notifications with the Bootstrap Notify plugin can provide a visually appealing way to display notifications to users in your web application. The Bootstrap Notify plugin allows you to create customizable and stylish notification alerts.
Here’s a general guide on how you might integrate Laravel notifications with the Bootstrap Notify plugin:
- Include Bootstrap and Bootstrap Notify: Make sure you have included the Bootstrap CSS and JavaScript files in your layout. Also, include the Bootstrap Notify JavaScript file.
<!-- In your HTML layout -->
<link rel="stylesheet" href="path/to/bootstrap.css">
<script src="path/to/bootstrap.js"></script>
<script src="path/to/bootstrap-notify.js"></script>
- Create a Notification Class: Create a Laravel notification class as explained in the previous response.
- Customize the Notification Behavior: Customize the notification to include the JavaScript code needed to trigger the Bootstrap Notify alert.
// app/Notifications/BootstrapNotifyNotification.php
use Illuminate\Notifications\Notification;
use Illuminate\Notifications\Messages\MailMessage;
class BootstrapNotifyNotification extends Notification
{
public function toBootstrapNotify($notifiable)
{
return [
'message' => 'This is your notification message.',
'type' => 'success', // success, info, warning, danger
];
}
}
- Trigger the Bootstrap Notify Alert: In your notification class, use the
toBootstrapNotify
method to customize the notification content and type. Then, in your view or controller, you can use the JavaScript code to trigger the Bootstrap Notify alert.
// In your controller or view
$user->notify(new BootstrapNotifyNotification());
<!-- In your JavaScript code -->
<script>
$(document).ready(function() {
@foreach (Auth::user()->notifications as $notification)
$.notify({
message: '{{ $notification->data['message'] }}'
},{
type: '{{ $notification->data['type'] }}'
});
@endforeach
});
</script>
In this example, the JavaScript code loops through the notifications for the authenticated user and triggers Bootstrap Notify alerts with the message and type specified in the notification.
Please note that this example assumes you are using jQuery alongside Bootstrap Notify. Additionally, you might need to adjust the code to fit your specific application structure and requirements.
Remember that the availability and compatibility of certain plugins, libraries, or code structures might have changed since my last update in September 2021. Always refer to the latest documentation for Laravel, Bootstrap, and any related plugins you are using.