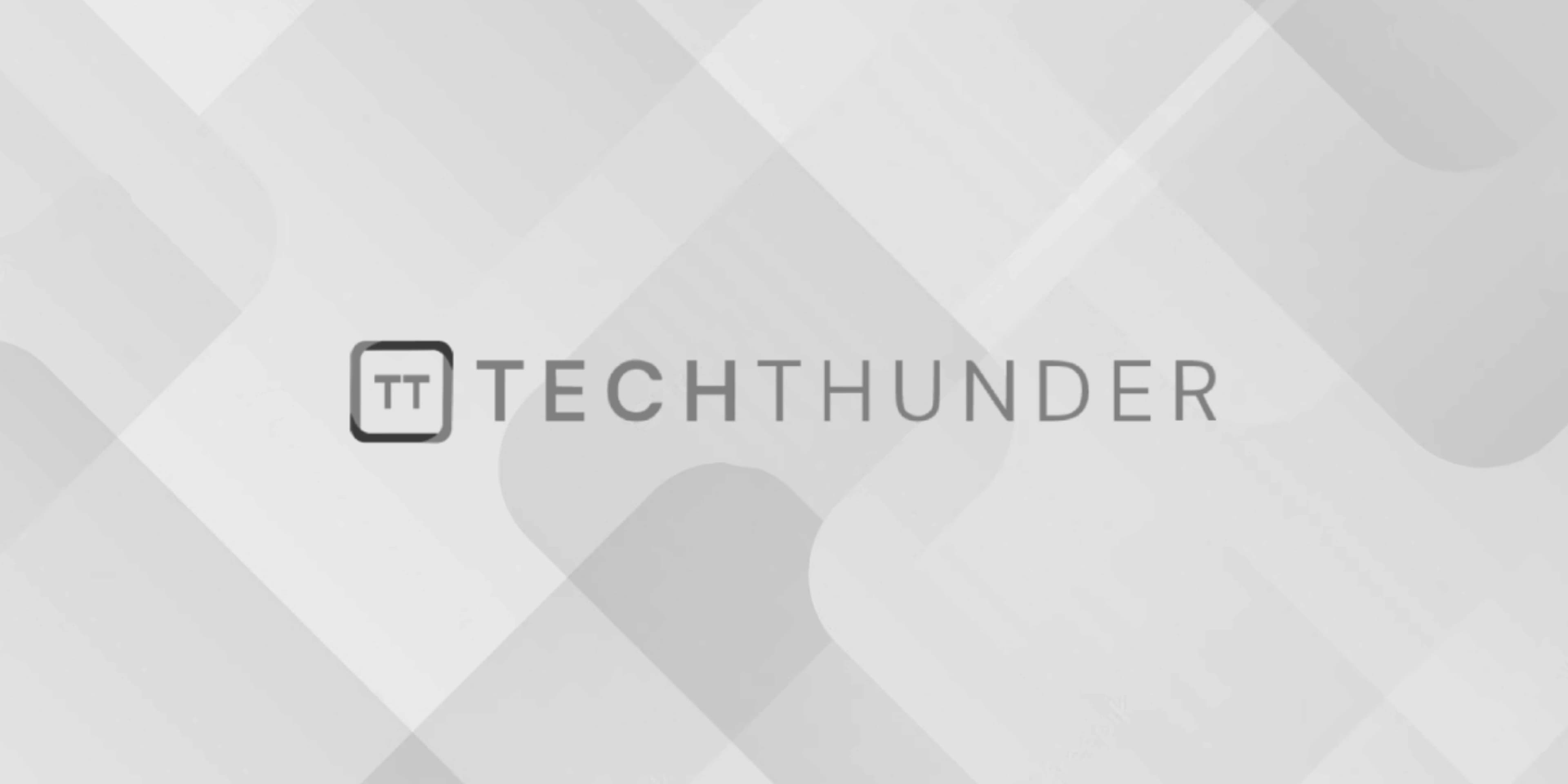
188 views
New Notification System using Laravel 5.7
Laravel provides a powerful notification system that allows you to send notifications across various channels like email, SMS, Slack, etc. Here’s a step-by-step guide to setting up a basic notification system using Laravel 5.7:
- Create a Notification Class: Start by creating a notification class using the
php artisan
command-line tool. This class will define how your notifications should be sent.
Bash
php artisan make:notification NewNotification
This will generate a NewNotification
class under the app/Notifications
directory.
- Configure the Notification: Open the
NewNotification
class that was generated. Here you can customize the notification’s behavior, including the channels it should be sent through and the content of the notification.
PHP
// app/Notifications/NewNotification.php
use Illuminate\Notifications\Notification;
use Illuminate\Notifications\Messages\MailMessage;
class NewNotification extends Notification
{
public function toMail($notifiable)
{
return (new MailMessage)
->line('The introduction to the notification.')
->action('Notification Action', url('/'))
->line('Thank you for using our application!');
}
}
- Sending Notifications: To send a notification, you’ll typically use the
notify
method on a notifiable entity (like a user).
PHP
use App\Notifications\NewNotification;
$user->notify(new NewNotification());
- Notification Channels: Laravel supports multiple notification channels like email, SMS, Slack, etc. By default, the notification will use the email channel. You can customize the channels by defining methods for each channel in your notification class (e.g.,
toMail
,toDatabase
,toSlack
, etc.). - Notification Views: If you’re using email notifications, Laravel will generate view files for your notifications. You can customize these views to style your emails. Views can be found in the
resources/views/vendor/notifications
directory. - Database Notifications: Laravel’s notification system can also store notifications in the database, allowing users to view their notifications when logged in. To use database notifications, you need to add the
Illuminate\Notifications\Notifiable
trait to your user model and configure the notification driver inconfig/notification.php
.
Remember that Laravel’s documentation is always the most accurate and up-to-date resource for learning about its features and implementation details. If you’re working with a version as old as Laravel 5.7, I strongly recommend checking the documentation specific to that version. For more recent versions, you should refer to the latest Laravel documentation.