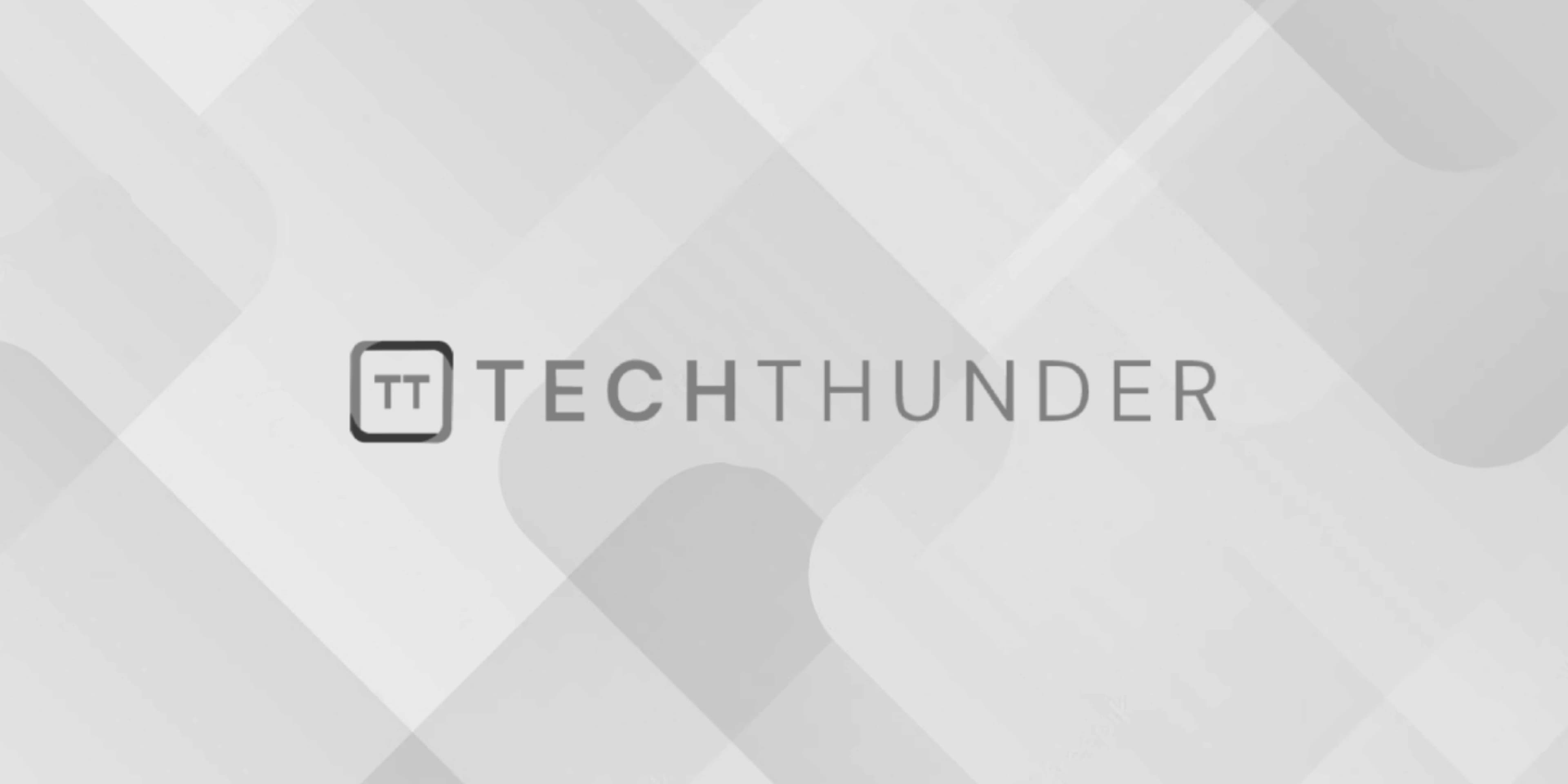
204 views
Delete All Records from Table in Laravel Eloquent
To delete all records from a table using Laravel Eloquent, you can use the delete()
method on the model associated with that table. Here are the steps to do this:
- Open the model file for the table you want to delete records from. This file is usually located in the
app
directory under theModels
folder. - In the model file, you can create a method to delete all records. For example, if you have a model named
YourModel
, you can add a static method like this:
use Illuminate\Database\Eloquent\Model;
class YourModel extends Model
{
// ...
public static function deleteAllRecords()
{
// Use the delete() method to delete all records in the table
self::query()->delete();
}
}
- Now, you can call this method from your controller or wherever you need to delete all records from the table:
YourModel::deleteAllRecords();
This will execute a DELETE query on the table associated with the YourModel
model, effectively deleting all records in that table.
Make sure you have proper access control and validation in place before performing mass deletions like this, as it will delete all records from the table without any conditions.