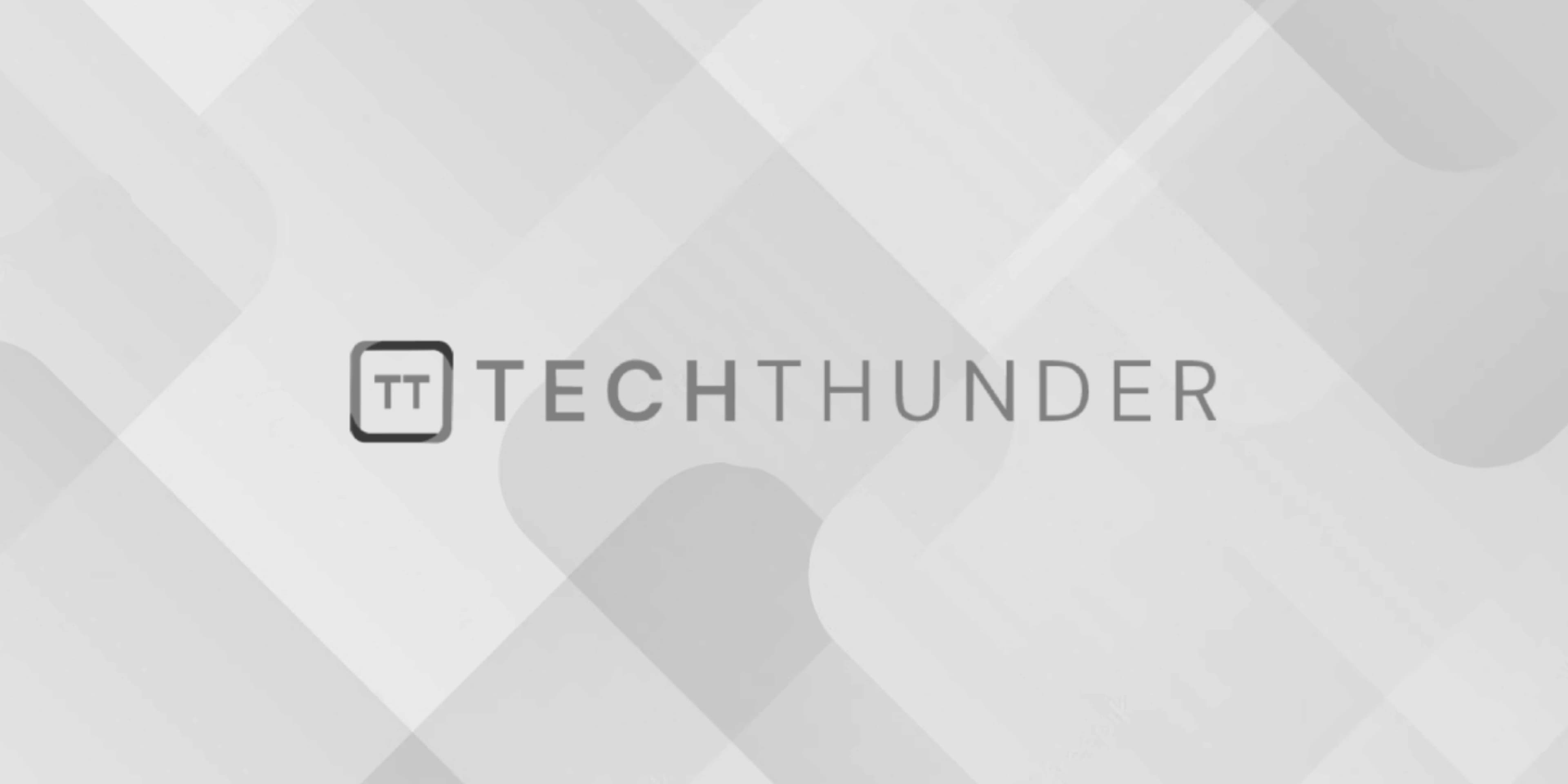
Laravel Eloquent
Laravel Eloquent is an Object-Relational Mapping (ORM) system included with the Laravel framework. Eloquent provides an elegant and expressive way to interact with your database tables and manage database records using PHP objects and methods, rather than writing raw SQL queries. It simplifies database operations and allows you to work with your database in a more object-oriented and intuitive manner.
Here are some key concepts and features of Laravel Eloquent:
1. Models:
In Eloquent, each database table is represented by a corresponding model class. Model classes extend Laravel’s Illuminate\Database\Eloquent\Model
class. A model defines the structure and behavior of the associated database table. For example, if you have a “users” table, you would create a “User” model.
namespace App\Models;
use Illuminate\Database\Eloquent\Model;
class User extends Model
{
// Model configuration goes here
}
2. Relationships:
Eloquent allows you to define relationships between models, such as one-to-one, one-to-many, and many-to-many relationships. You can define these relationships using methods in your model classes. For example, a “User” model might have a relationship with a “Post” model:
class User extends Model
{
public function posts()
{
return $this->hasMany(Post::class);
}
}
3. Query Builder:
Eloquent provides a query builder that allows you to build complex database queries using a fluent, method chaining syntax. You can use methods like where
, orderBy
, select
, and more to create queries.
$users = User::where('status', 'active')
->orderBy('created_at', 'desc')
->get();
4. CRUD Operations:
Eloquent makes it easy to perform Create, Read, Update, and Delete (CRUD) operations on database records. For example, to create a new user:
$user = new User;
$user->name = 'John Doe';
$user->email = '[email protected]';
$user->save();
To update a user’s email:
$user = User::find(1);
$user->email = '[email protected]';
$user->save();
5. Mass Assignment:
Eloquent supports mass assignment, where you can set multiple attributes at once. To enable mass assignment for specific attributes, you can define a fillable
property in your model:
class User extends Model
{
protected $fillable = ['name', 'email'];
}
6. Eloquent ORM Events:
Eloquent allows you to define events that trigger when certain actions are performed on a model, such as creating, updating, or deleting records. You can use these events to perform additional actions or modifications when necessary.
7. Eloquent Collections:
Eloquent returns query results as collections, which are powerful arrays with a wide range of helpful methods for manipulating and working with data.
8. Eager Loading:
Eloquent supports eager loading, which helps you reduce the number of database queries when retrieving related data. This is especially useful for avoiding the N+1 query problem.
These are some of the core concepts and features of Laravel Eloquent. It provides a convenient and intuitive way to work with databases in Laravel applications, making it easier to manage and manipulate database records. Eloquent is a significant factor in Laravel’s popularity and productivity.