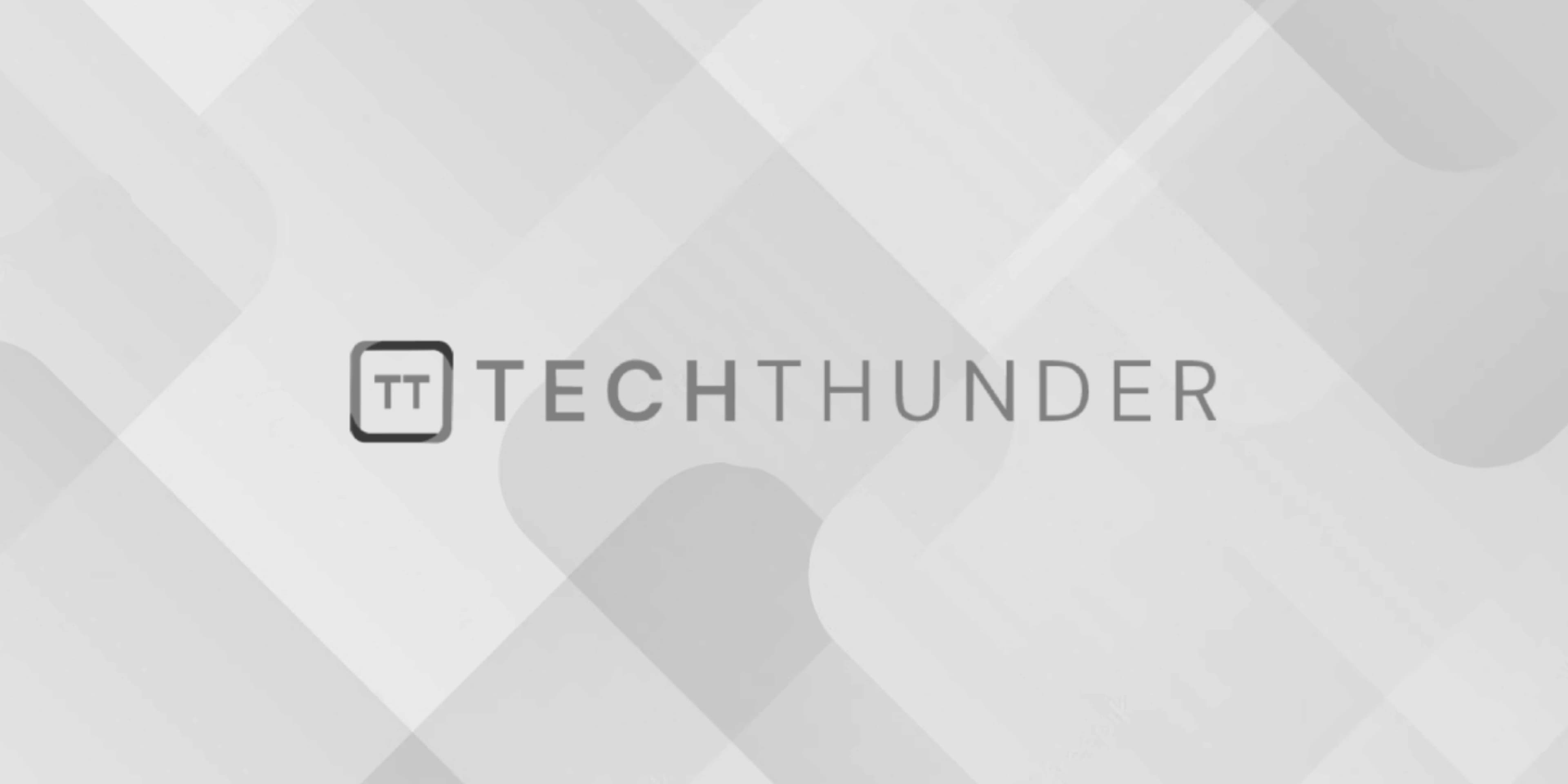
Laravel Validation
Laravel provides a powerful validation system that allows you to easily validate user input, ensuring that data is valid before it’s processed or stored in your application. Here’s a step-by-step guide on how to use Laravel’s validation:
Step 1: Define Validation Rules
In your controller or wherever you handle the request, define the validation rules for your incoming data using the validate
method or the Validator
facade. For example:
use Illuminate\Http\Request;
public function store(Request $request)
{
$validatedData = $request->validate([
'name' => 'required|string|max:255',
'email' => 'required|email|unique:users|max:255',
'password' => 'required|min:6',
]);
// Continue with your logic if validation passes
}
In this example, we’ve defined rules for the name
, email
, and password
fields. The validate
method will automatically redirect back with validation errors if any rules are violated.
Step 2: Display Validation Errors in Views
In your views, you can display validation errors using the @error
directive. For example:
<input type="text" name="name" value="{{ old('name') }}">
@error('name')
<div class="alert alert-danger">{{ $message }}</div>
@enderror
Here, we use old('name')
to repopulate the field with the user’s input if validation fails.
Step 3: Customize Error Messages
You can customize error messages by specifying them in the resources/lang/en/validation.php
language file. Laravel provides default error messages, which you can override with your own messages.
Step 4: Conditional Validation Rules
You can apply conditional validation rules based on certain conditions. For example, you can use the required_if
rule:
$request->validate([
'credit_card' => 'required_if:payment_method,credit_card',
]);
In this example, the ‘credit_card’ field is required only if the ‘payment_method’ field is ‘credit_card’.
Step 5: Form Request Validation (Optional)
For more complex validation or when dealing with multiple forms, you can create custom form request classes using Artisan:
php artisan make:request StorePostRequest
In the generated StorePostRequest.php
file, define your validation rules in the rules
method:
public function rules()
{
return [
'title' => 'required|string|max:255',
'content' => 'required|string',
];
}
Then, type-hint your custom request class in your controller method:
use App\Http\Requests\StorePostRequest;
public function store(StorePostRequest $request)
{
// Validation rules are defined in the form request class
// Continue with your logic if validation passes
}
Using form request validation keeps your controllers clean and organized.
Step 6: Additional Validation Techniques
Laravel offers a wide range of validation rules and techniques, including:
- Numeric validation:
integer
,numeric
,between
, etc. - String validation:
string
,max
,min
,regex
, etc. - Date and time validation:
date
,date_format
,before
,after
, etc. - File validation:
file
,image
,mimes
,size
, etc. - Custom validation rules: Define custom rules and validators.
You can explore these options in the Laravel documentation: https://laravel.com/docs/validation
Step 7: Testing Validation
Always thoroughly test your validation rules to ensure that they work as expected. Laravel provides testing tools like PHPUnit and Laravel Dusk for this purpose.
With Laravel’s validation system, you can easily ensure that your application’s input data is secure and valid, providing a better user experience and preventing potential security issues.