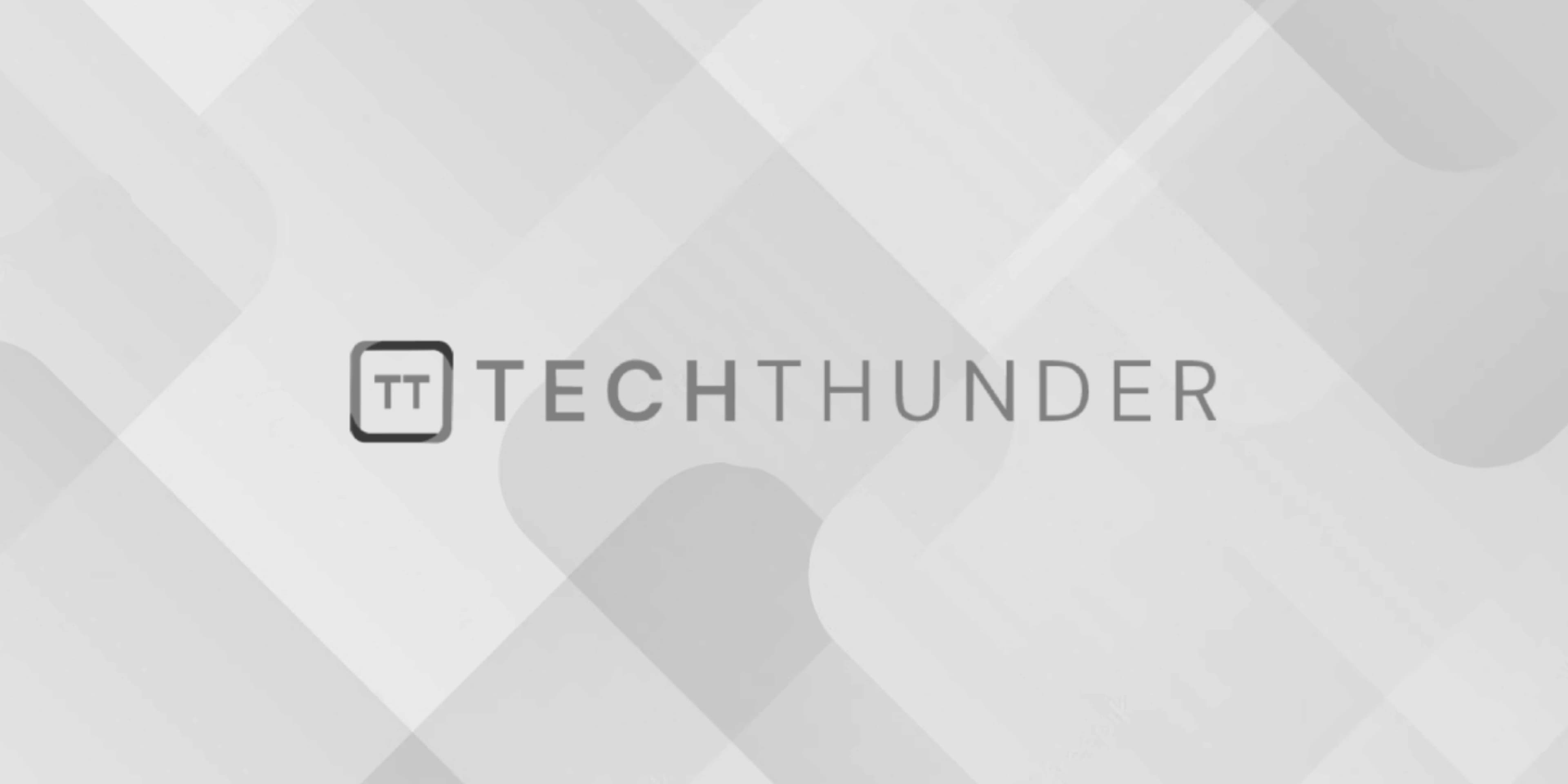
Laravel Sessions
The Laravel, sessions allow you to store data on the server and associate it with a specific user’s browsing session. This data can be used to persist information across multiple HTTP requests and is essential for building features like user authentication, shopping carts, and more. Here’s how to work with sessions in Laravel:
1. Starting a Session:
By default, Laravel starts a session for each incoming HTTP request. There’s no need to manually start a session. Laravel handles this for you.
2. Storing Data in the Session:
You can store data in the session using the session
global helper or the Session
facade. For example:
// Using the session helper
session(['key' => 'value']);
// Using the Session facade
\Session::put('key', 'value');
3. Retrieving Data from the Session:
To retrieve data from the session, use the session
helper or the Session
facade:
// Using the session helper
$value = session('key');
// Using the Session facade
$value = \Session::get('key');
4. Flash Data:
Flash data is a type of session data that is only available for the next request. You can flash data using the session
helper or the Session
facade:
// Flash data using the session helper
session()->flash('message', 'This is a flashed message.');
// Flash data using the Session facade
\Session::flash('message', 'This is a flashed message.');
5. Retrieving and Keeping Flash Data:
To retrieve flashed data and keep it for the subsequent request, you can use the session
helper or the Session
facade:
// Retrieve flashed data
$message = session('message');
// Retrieve and keep flashed data
$message = session('message', 'default-value');
6. Deleting Data from the Session:
To remove data from the session, use the forget
method:
// Remove a specific item from the session
session()->forget('key');
// Remove all items from the session
session()->flush();
7. Checking for Data in the Session:
You can check if a specific item exists in the session using the has
method:
if (session()->has('key')) {
// The item exists in the session
}
8. Retrieving All Session Data:
To retrieve all data stored in the session, you can use the all
method:
$data = session()->all();
9. Using the with
Method in Redirects:
You can use the with
method when redirecting to pass data to the next request:
return redirect('/')->with('key', 'value');
You can then retrieve this data in the redirected route.
10. Customizing the Session Configuration:
Laravel’s session configuration is stored in the config/session.php
file. You can customize session settings, such as the session driver, lifetime, and more, by editing this file.
11. Session Drivers:
Laravel supports various session drivers, including the default file-based driver, database driver, and more. You can configure the driver in the config/session.php
file.
12. Using Database Sessions:
If you choose the database session driver, you need to run the php artisan session:table
and php artisan migrate
commands to create the necessary database table for sessions.
13. Protecting Session Data:
Laravel automatically encrypts and signs session data to prevent tampering. You don’t need to worry about data security in the session.
Laravel’s session management system is flexible and powerful, making it easy to work with user data across HTTP requests in your applications.