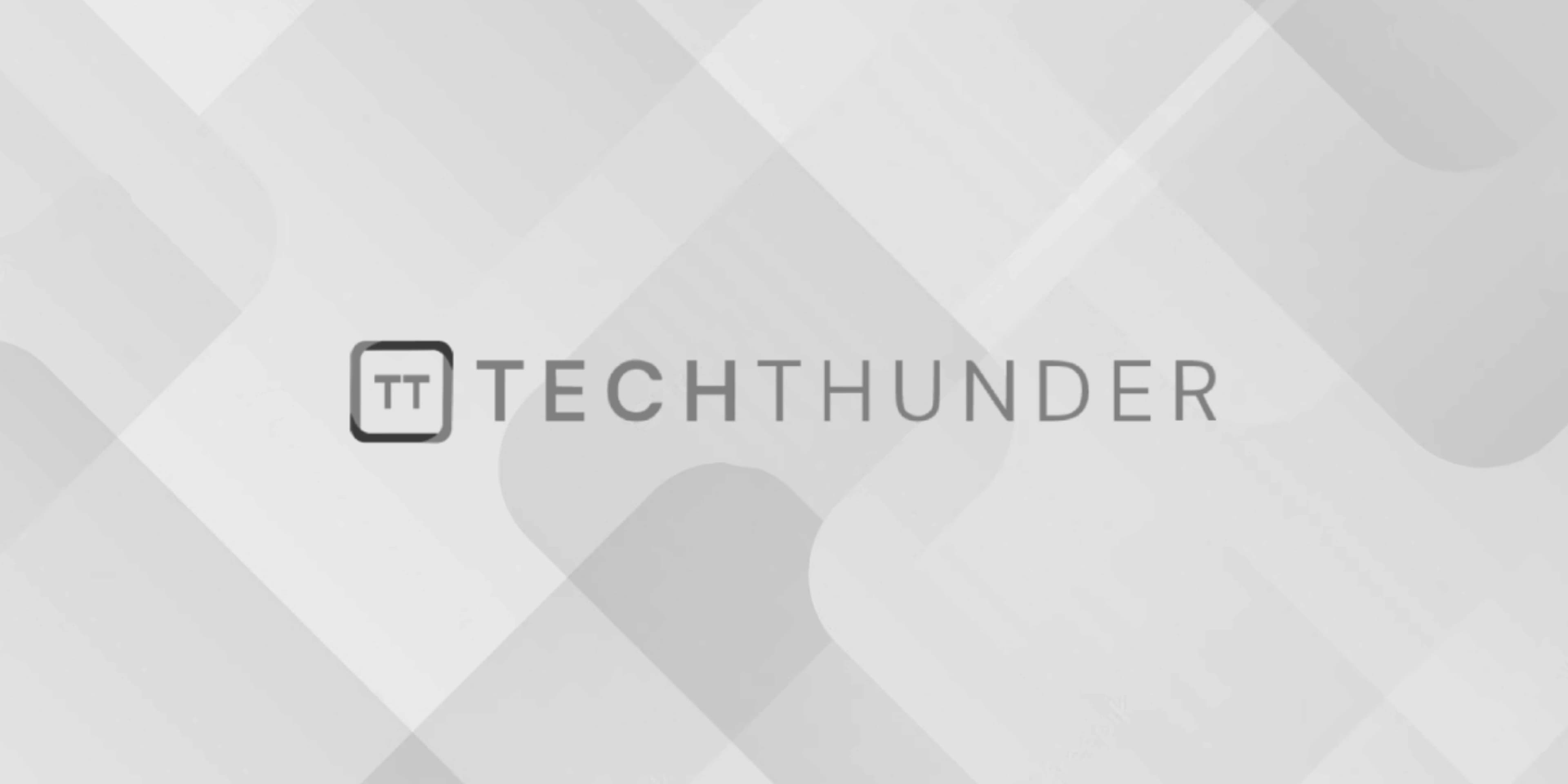
Laravel Basic Routing
The laravel basic routing is a fundamental concept that defines how your application responds to HTTP requests. Routing maps HTTP request URIs (Uniform Resource Identifiers) to specific controller actions or closures, allowing you to determine what code should be executed for a given request.
Here’s how to work with basic routing in Laravel:
1. Route Definitions:
Route definitions are typically located in the routes/web.php
or routes/api.php
file. These files contain a collection of route definitions using the Route
facade or helper functions.
use Illuminate\Support\Facades\Route;
Route::get('/example', 'ExampleController@index');
Route::post('/submit', 'FormController@submit');
Route::put('/update/{id}', 'DataController@update');
In the example above, we have defined three routes:
- A
GET
route that maps the/example
URI to theindex
method of theExampleController
. - A
POST
route that maps the/submit
URI to thesubmit
method of theFormController
. - A
PUT
route with a route parameter{id}
that maps to theupdate
method of theDataController
.
2. Route Parameters:
You can define route parameters by enclosing them in curly braces {}
in your route URI. These parameters capture values from the URI and pass them as arguments to the controller method or closure.
Route::get('/user/{id}', 'UserController@show');
In this example, {id}
is a route parameter that can capture any value from the URI. You can access this parameter in the show
method of the UserController
.
3. Route Names:
Assigning names to routes allows you to reference them by name rather than their URLs. This can make your code more readable and maintainable.
Route::get('/dashboard', 'DashboardController@index')->name('dashboard');
With the route name set as “dashboard,” you can generate URLs or perform redirects using the route name:
$url = route('dashboard'); // Generates the URL for the 'dashboard' route.
4. HTTP Verbs and Actions:
Laravel supports various HTTP verbs (GET, POST, PUT, PATCH, DELETE, etc.) to define routes for different types of actions. You can use the following methods to define routes:
Route::get()
: For handling HTTP GET requests.Route::post()
: For handling HTTP POST requests.Route::put()
: For handling HTTP PUT requests.Route::patch()
: For handling HTTP PATCH requests.Route::delete()
: For handling HTTP DELETE requests.Route::any()
: For handling any HTTP method.
5. Route Groups:
You can group related routes together and apply common middleware, route attributes, and other settings using the Route::group
method. This helps organize routes and keep your code clean.
Route::middleware(['auth'])->group(function () {
Route::get('/profile', 'ProfileController@index');
Route::get('/settings', 'ProfileController@settings');
});
In this example, both /profile
and /settings
routes are grouped and share the “auth” middleware.
These are the basic concepts of routing in Laravel. By defining routes, you can map URLs to specific controller actions or closures, making it easy to create web applications with structured and organized code.