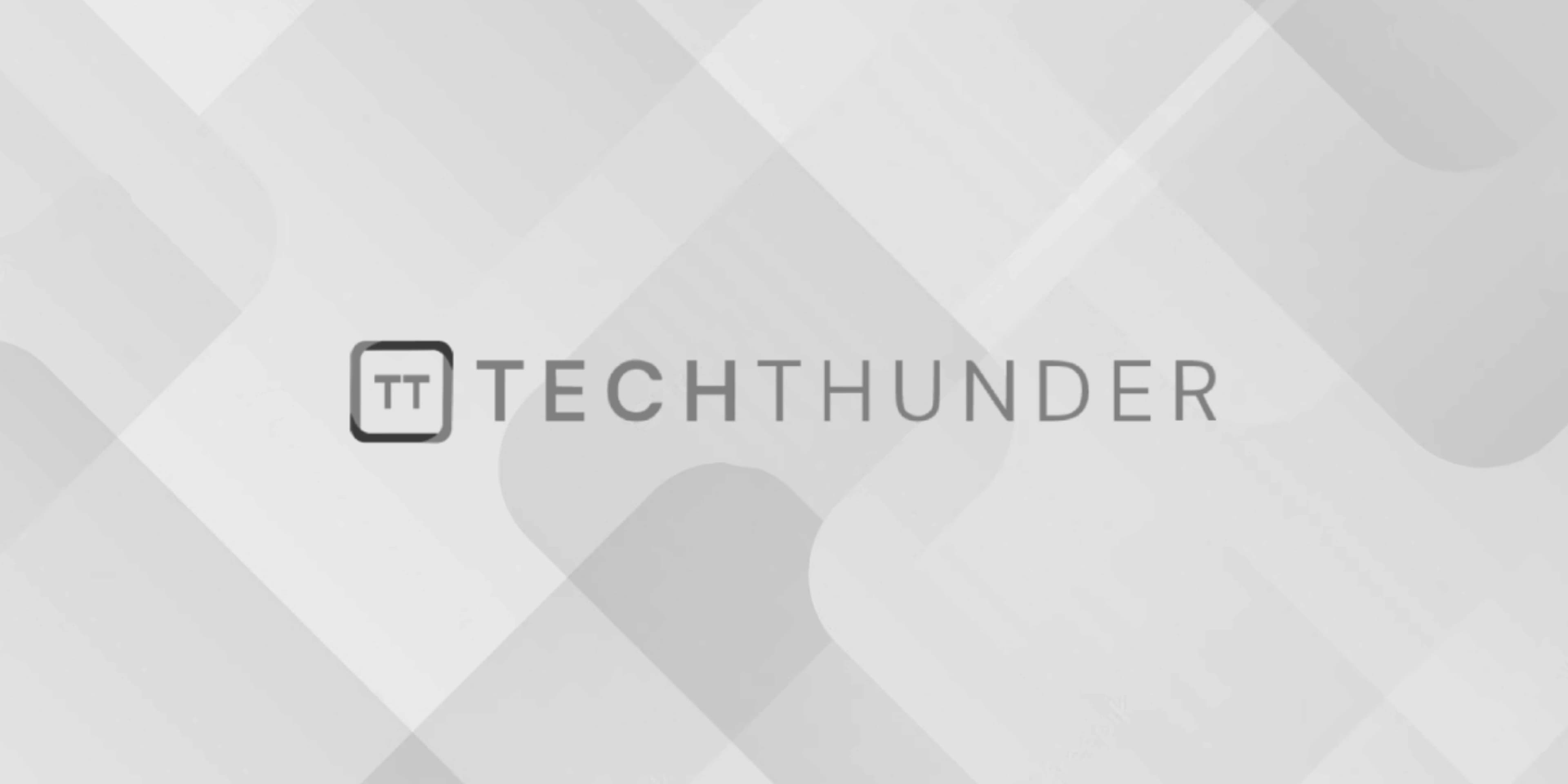
Laravel Controller Middleware
The laravel middleware provides a convenient way to filter HTTP requests entering your application. Middleware can be applied to routes and controllers to perform tasks like authentication, authorization, logging, and more before or after the request reaches your controller. Middleware allows you to separate concerns and keep your code clean and organized.
Here’s how you can use middleware with Laravel controllers:
1. Creating Middleware:
You can create custom middleware using the make:middleware
Artisan command. For example, to create a middleware named “CheckAge,” run:
php artisan make:middleware CheckAge
This will generate a new middleware file in the app/Http/Middleware
directory.
2. Middleware Logic:
Open the generated middleware file (e.g., CheckAge.php
) and define your custom logic in the handle
method. This method is executed for each incoming request. You can modify the request, perform checks, or take any actions you need.
public function handle($request, Closure $next)
{
if ($request->age < 18) {
return redirect('home');
}
return $next($request);
}
In this example, the middleware checks if the user’s age is less than 18 and redirects them to the “home” route if it is.
3. Registering Middleware:
To make your custom middleware available to your application, you need to register it in the app/Http/Kernel.php
file. The $middleware
property in the Kernel
class contains an array of global middleware that runs on every request, and the $routeMiddleware
property contains named middleware that you can apply to specific routes or controllers.
// Add your middleware to the $routeMiddleware property
protected $routeMiddleware = [
'check.age' => \App\Http\Middleware\CheckAge::class,
];
4. Applying Middleware to Routes:
You can apply middleware to routes in the routes/web.php
or routes/api.php
file. Use the middleware
method to specify which middleware should run for a particular route:
Route::get('profile', 'ProfileController@index')->middleware('check.age');
In this example, the “check.age” middleware will run before the “index” method of the “ProfileController” is executed.
5. Applying Middleware to Controllers:
You can also apply middleware to an entire controller by defining it in the controller’s constructor method:
public function __construct()
{
$this->middleware('check.age');
}
This will apply the “check.age” middleware to all methods within the controller.
6. Middleware Parameters:
You can pass parameters to middleware by specifying them after the middleware name. For example, if your middleware needs a parameter, you can define it like this:
Route::get('profile', 'ProfileController@index')->middleware('check.age:18');
Then, in your middleware’s handle
method, you can access this parameter:
public function handle($request, Closure $next, $age)
{
// Use the $age parameter
}
These are the basics of using middleware with Laravel controllers. Middleware allows you to filter and process HTTP requests before they reach your controllers, making it a powerful tool for tasks like authentication, authorization, logging, and more.