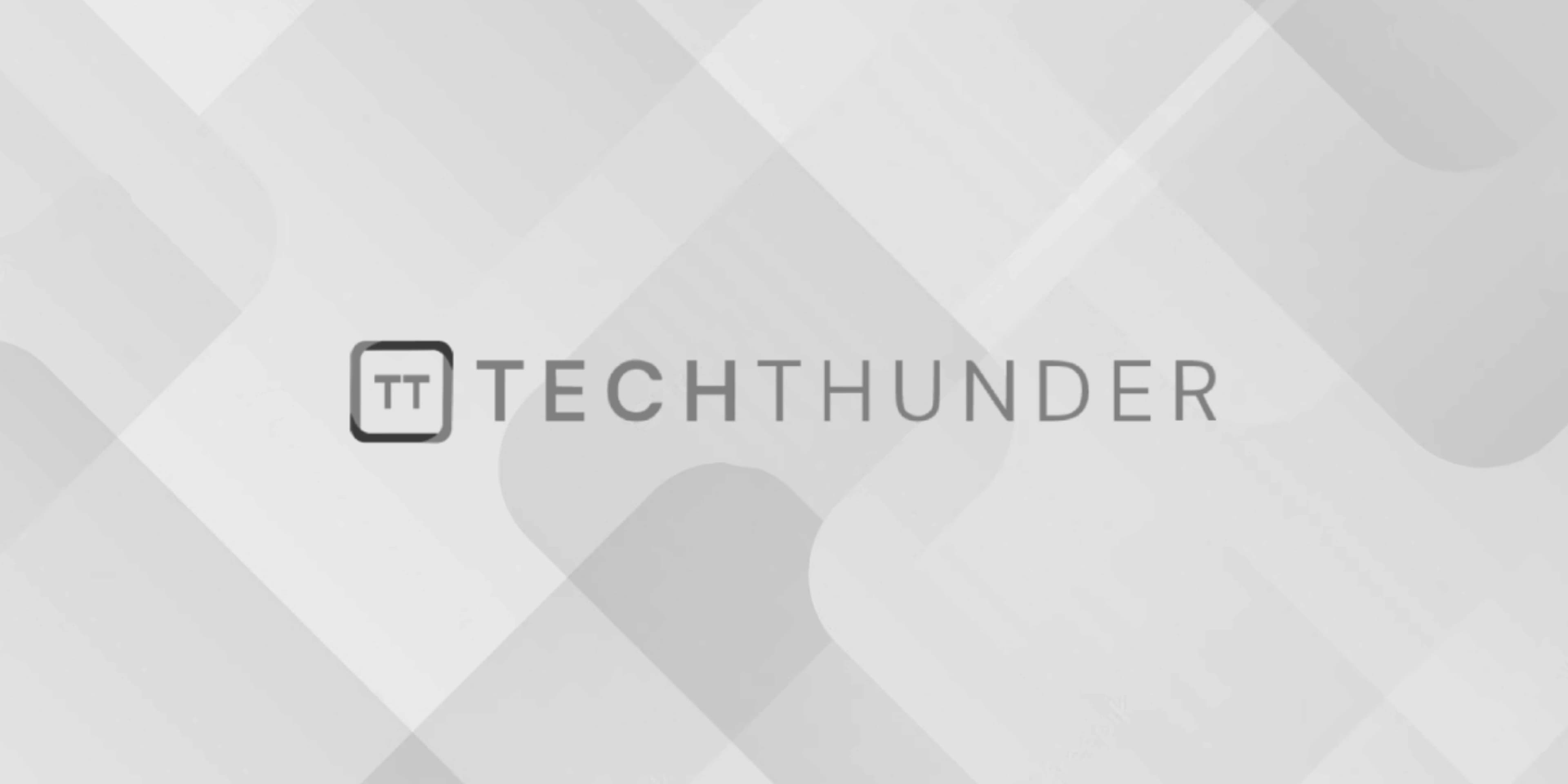
Immutable String
The Java string is immutable, which means that its content cannot be changed once it is created. When you perform operations on a string, such as concatenation or substring extraction, it does not modify the original string but instead creates a new string object with the modified content. This immutability is a fundamental property of the String
class in Java.
Here are some key characteristics of immutable strings:
- Creation: When you create a
String
object, it remains the same throughout its lifetime. Any operation that appears to modify the string actually creates a new string object. - Thread-Safe: Immutable strings are inherently thread-safe. Multiple threads can safely read and share
String
objects without synchronization concerns because there are no changes to the content. - Efficiency: While it may seem that creating new string objects for every operation is inefficient, modern JVMs and compilers are optimized to minimize the performance impact of string manipulation. In fact, immutability can lead to certain optimizations, such as string interning, which allows multiple references to the same string literal to share the same memory.
- Value Comparison: When comparing string values using methods like
equals()
, you are comparing the content of the strings, not their references. This allows you to check whether two strings have the same value without worrying about their memory addresses.
Here’s an example demonstrating string immutability:
String original = "Hello";
String modified = original.concat(", World!"); // A new string is created
System.out.println(original); // Output: Hello
System.out.println(modified); // Output: Hello, World!
In this example, the concat()
method does not modify the original
string; instead, it creates a new string modified
.
Using immutable strings ensures data integrity and simplifies string handling in your code. If you need to perform extensive string manipulations, consider using the StringBuilder
or StringBuffer
classes, which are designed for mutable string operations.