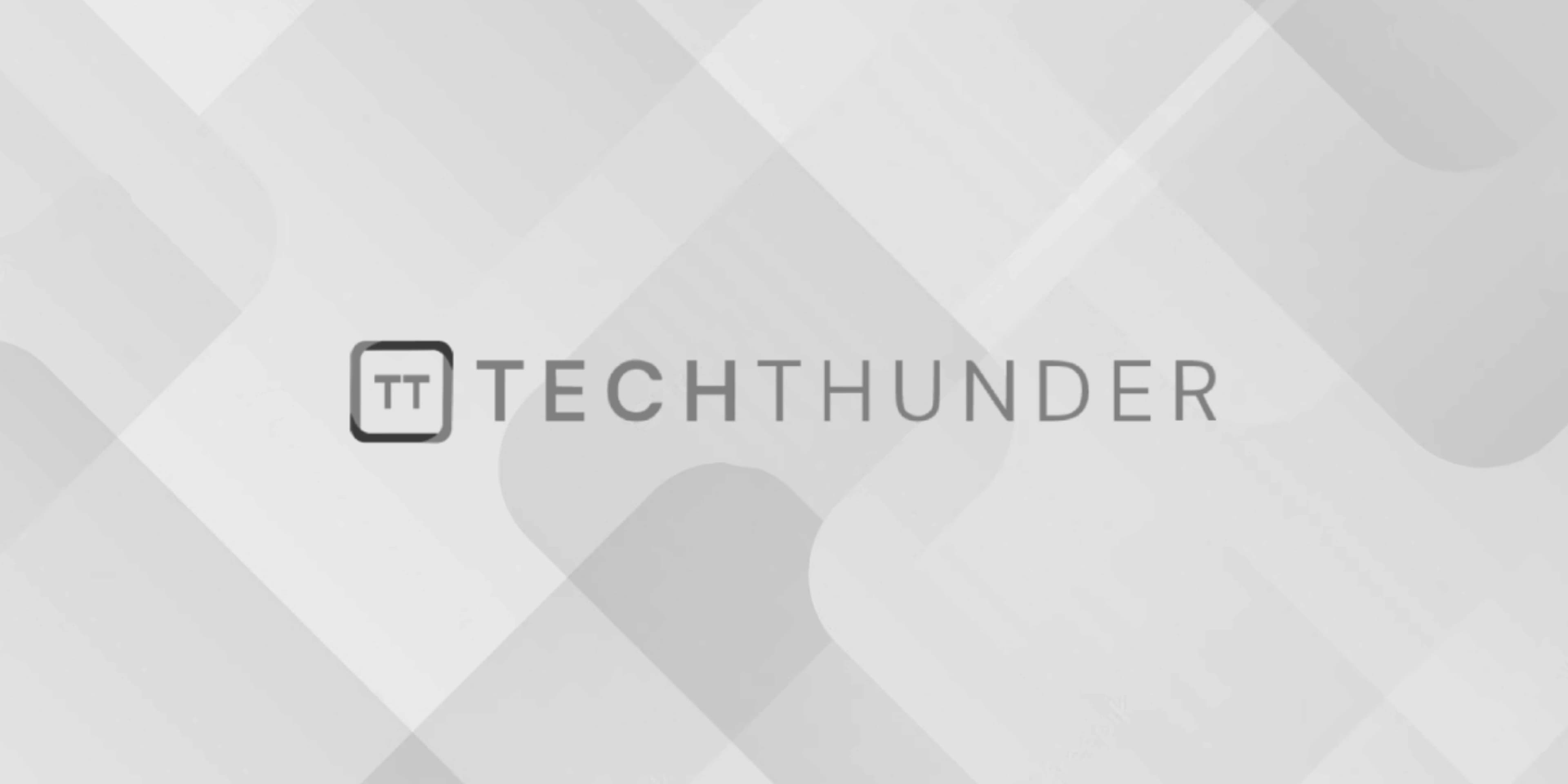
165 views
StringTokenizer class
The StringTokenizer
class in Java is used for breaking a string into smaller tokens, which are typically separated by specific delimiter characters or by whitespace. It’s a legacy class, and modern Java applications often use other techniques, such as String.split()
or regular expressions, for string parsing. However, StringTokenizer
can still be useful for some specific use cases.
Here’s an overview of the StringTokenizer
class:
- Initialization:
- You create a
StringTokenizer
object by specifying the input string and optionally specifying the delimiter characters that determine how the string is split into tokens. - The basic constructor is
StringTokenizer(String str)
, which uses the default delimiter characters (whitespace) to split the string. - You can also provide a custom set of delimiter characters by using the constructor
StringTokenizer(String str, String delim)
.
- Tokenization:
- The
StringTokenizer
object provides methods for retrieving tokens from the input string. - The main methods for tokenization are:
boolean hasMoreTokens()
: Returnstrue
if there are more tokens in the input string.String nextToken()
: Returns the next token from the input string.String nextToken(String delim)
: Allows changing the delimiter on the fly for the next token.
- Count Tokens:
- The
countTokens()
method returns the total number of tokens remaining in the input string.
- Examples: Here’s a simple example of using
StringTokenizer
to split a string using whitespace as the delimiter:
String input = "This is a simple example";
StringTokenizer tokenizer = new StringTokenizer(input);
while (tokenizer.hasMoreTokens()) {
String token = tokenizer.nextToken();
System.out.println(token);
}
You can also specify custom delimiter characters:
String input = "apple,banana,cherry,date";
StringTokenizer tokenizer = new StringTokenizer(input, ",");
while (tokenizer.hasMoreTokens()) {
String token = tokenizer.nextToken();
System.out.println(token);
}
- Limitations:
StringTokenizer
is considered a legacy class and is not the most efficient or flexible option for string parsing in modern Java. For more complex splitting patterns, regular expressions orString.split()
are often more suitable.
- Alternatives:
- The
split()
method of theString
class can be more flexible and is often preferred for simple delimiter-based splitting. For more complex splitting patterns, Java regular expressions (Pattern
andMatcher
) are a powerful choice. - If you need more advanced parsing and tokenization, consider using libraries like Apache Commons Lang or the Java Scanner class.
StringTokenizer
is a simple class for splitting strings into tokens based on specified delimiters. It can be useful for straightforward tokenization tasks, but for more complex requirements, consider using regular expressions or other parsing techniques.