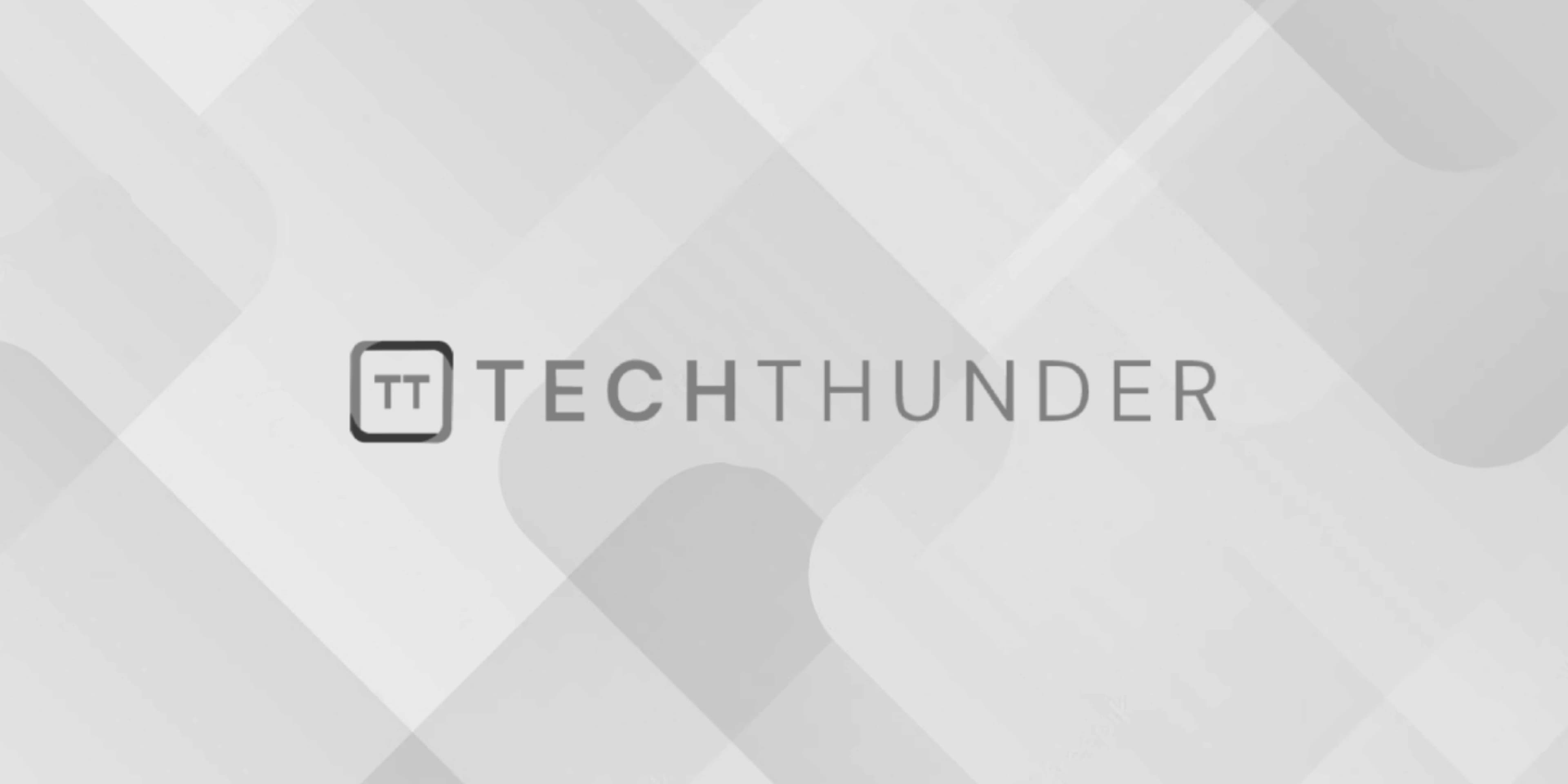
String lastIndexOf()
The lastIndexOf()
method in java is a part of the String
class and is used to find the index of the last occurrence of a specified substring or character within the string. It searches the string from the end to the beginning and returns the index of the last occurrence, or -1 if the substring or character is not found.
The basic syntax of the lastIndexOf()
method is as follows:
int lastIndexOf(String str)
int lastIndexOf(String str, int fromIndex)
int lastIndexOf(char ch)
int lastIndexOf(char ch, int fromIndex)
Here’s how you can use it:
- Using a substring:
String text = "Hello, world! Hello!";
int lastIndex = text.lastIndexOf("Hello"); // 13
In this example, lastIndexOf("Hello")
returns 13 because the last occurrence of “Hello” in the string starts at index 13.
- Using a character:
String text = "Hello, world!";
int lastIndex = text.lastIndexOf('o'); // 8
In this example, lastIndexOf('o')
returns 8 because the last occurrence of the character ‘o’ in the string is at index 8.
- Specifying a fromIndex:
String text = "Hello, world! Hello!";
int lastIndex = text.lastIndexOf("Hello", 12); // 0
In this example, lastIndexOf("Hello", 12)
starts searching for the last occurrence of “Hello” from index 12 backwards and finds it at index 0.
Keep in mind:
- The
lastIndexOf()
methods return -1 if the substring or character is not found. - If you provide the optional
fromIndex
argument, the search starts from that index and goes backward. IffromIndex
is greater than or equal to the length of the string, the entire string is searched. - The
lastIndexOf()
methods are case-sensitive. If you need case-insensitive searching, you can convert the string to lowercase or uppercase before performing the search.
Here’s an example with an optional case-insensitive search:
String text = "HeLlO, WoRlD!";
int lastIndexIgnoreCase = text.toLowerCase().lastIndexOf("hello"); // 0
In this example, lastIndexOf("hello")
is case-insensitive due to the conversion of the string to lowercase using toLowerCase()
.