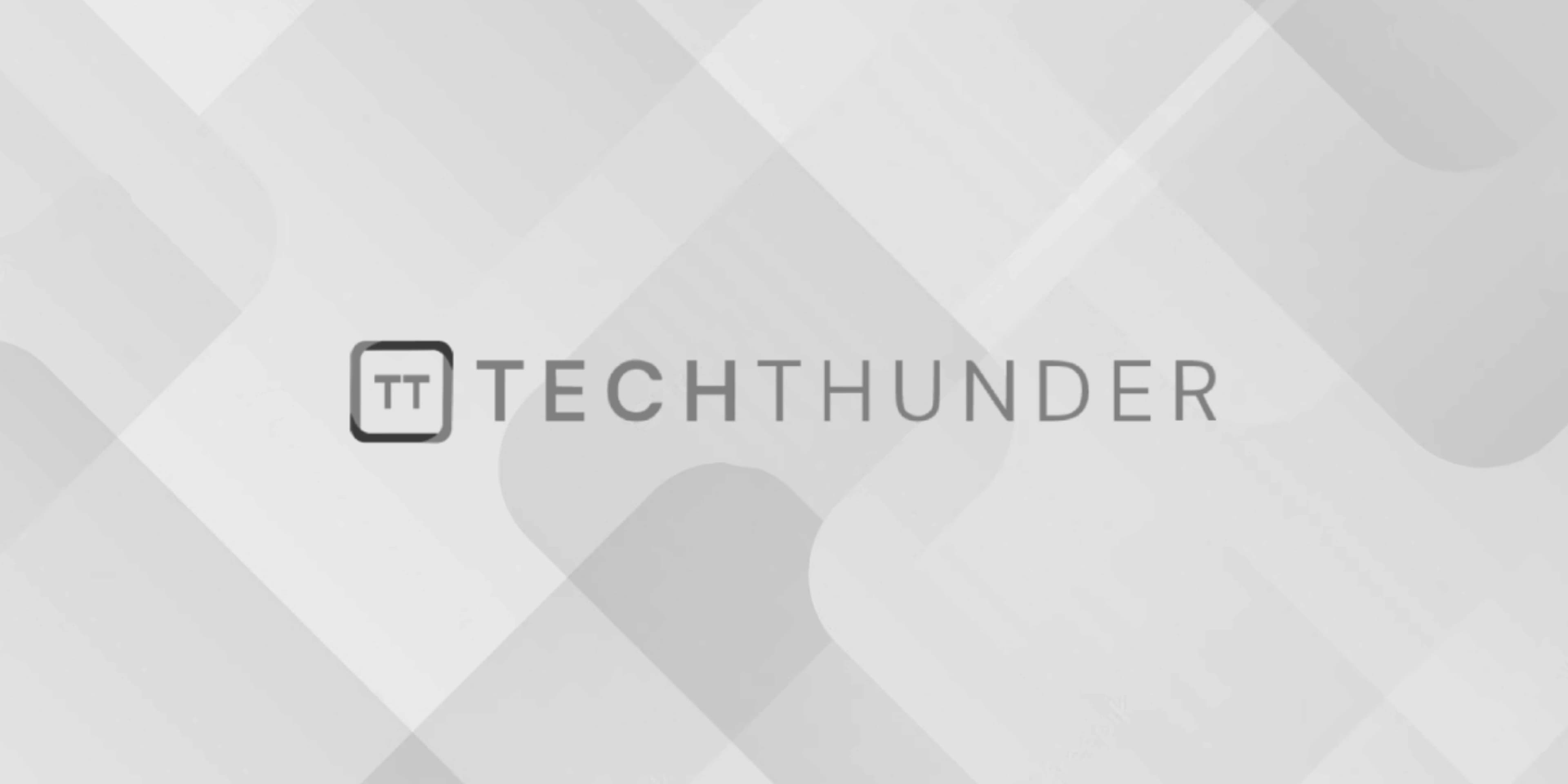
String format()
The Java String.format()
method is used to create a formatted string by applying a specified format to a set of given arguments. It allows you to construct strings with placeholders, where the placeholders are replaced by the values of the provided arguments according to the specified format.
The basic syntax of the String.format()
method is as follows:
String formattedString = String.format(format, args);
format
: A format string that specifies how the resulting string should be formatted. The format string contains placeholders, such as%s
for strings,%d
for integers,%f
for floating-point numbers, and so on.args
: A variable number of arguments that correspond to the placeholders in the format string.
Here’s a simple example of using String.format()
to create a formatted string:
public class StringFormatExample {
public static void main(String[] args) {
String name = "Alice";
int age = 30;
double height = 1.75;
String formattedString = String.format("Name: %s, Age: %d, Height: %.2f meters", name, age, height);
System.out.println(formattedString);
}
}
In this example, the String.format()
method is used to create a formatted string with placeholders for the name, age, and height. The placeholders %s
, %d
, and %.2f
are replaced by the values of the name
, age
, and height
variables, respectively.
The resulting formattedString
will be: “Name: Alice, Age: 30, Height: 1.75 meters” (with the height rounded to two decimal places).
You can use various format specifiers to control how the data is displayed in the formatted string. Some common format specifiers include:
%s
: Format as a string.%d
: Format as an integer.%f
: Format as a floating-point number.%.2f
: Format as a floating-point number with two decimal places.%t
: Format as a date and time.
The format string can also include additional formatting options, such as width, precision, and alignment. For more complex formatting needs, you can refer to the documentation on Java’s String.format()
for a comprehensive list of format specifiers and options.