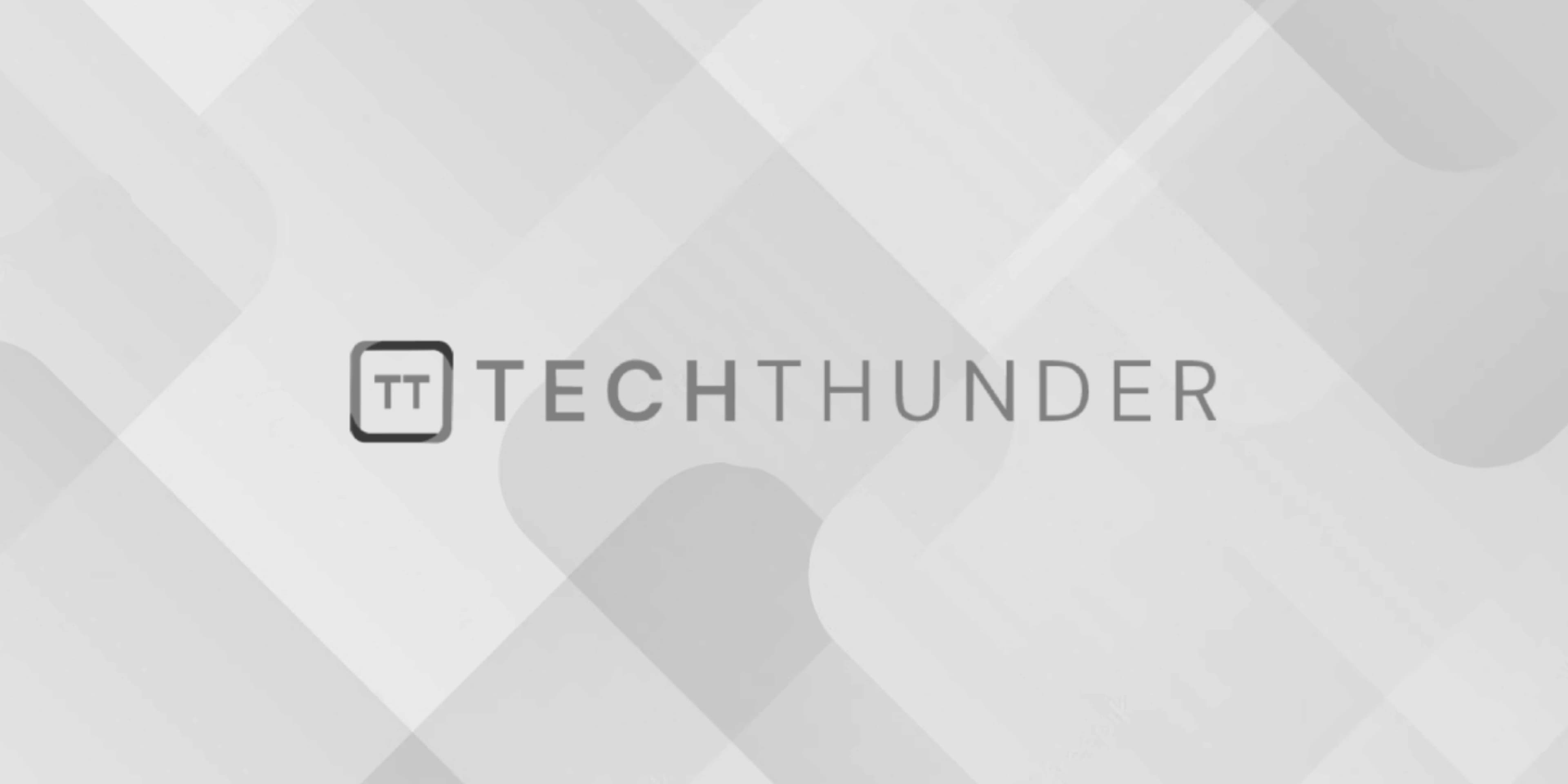
String substring()
The substring()
method is a part of the String
class and is used to extract a portion of a string. It returns a new string that represents the specified substring.
The basic syntax of the substring()
method is as follows:
String substring(int beginIndex)
String substring(int beginIndex, int endIndex)
Here’s how you can use it:
- Using a single argument (beginIndex):
String original = "Hello, world!";
String substring = original.substring(7); // "world!"
In this example, calling substring(7)
extracts the portion of the original string starting from index 7 (inclusive) to the end of the string.
- Using two arguments (beginIndex and endIndex):
String original = "Hello, world!";
String substring = original.substring(0, 5); // "Hello"
In this example, calling substring(0, 5)
extracts the portion of the original string starting from index 0 (inclusive) up to index 5 (exclusive), which results in the substring “Hello”.
It’s important to note the following about the substring()
method:
- The
beginIndex
is inclusive, meaning the character at that index is included in the substring. - The
endIndex
is exclusive, meaning the character at that index is not included in the substring. - If you provide only one argument (
beginIndex
), the substring will be extracted from that index to the end of the string. - If you provide two arguments (
beginIndex
andendIndex
), the substring will be extracted frombeginIndex
up to, but not including,endIndex
. - If the
beginIndex
is greater than or equal to the length of the string, an empty string is returned. - If the
endIndex
is greater than the length of the string, it is automatically adjusted to the length of the string.
Here are a few more examples:
String original = "abcdef";
String sub1 = original.substring(2, 4); // "cd"
String sub2 = original.substring(1, 3); // "bc"
String sub3 = original.substring(4); // "ef"
Keep in mind that the substring()
method returns a new string that represents the extracted substring. The original string remains unchanged.