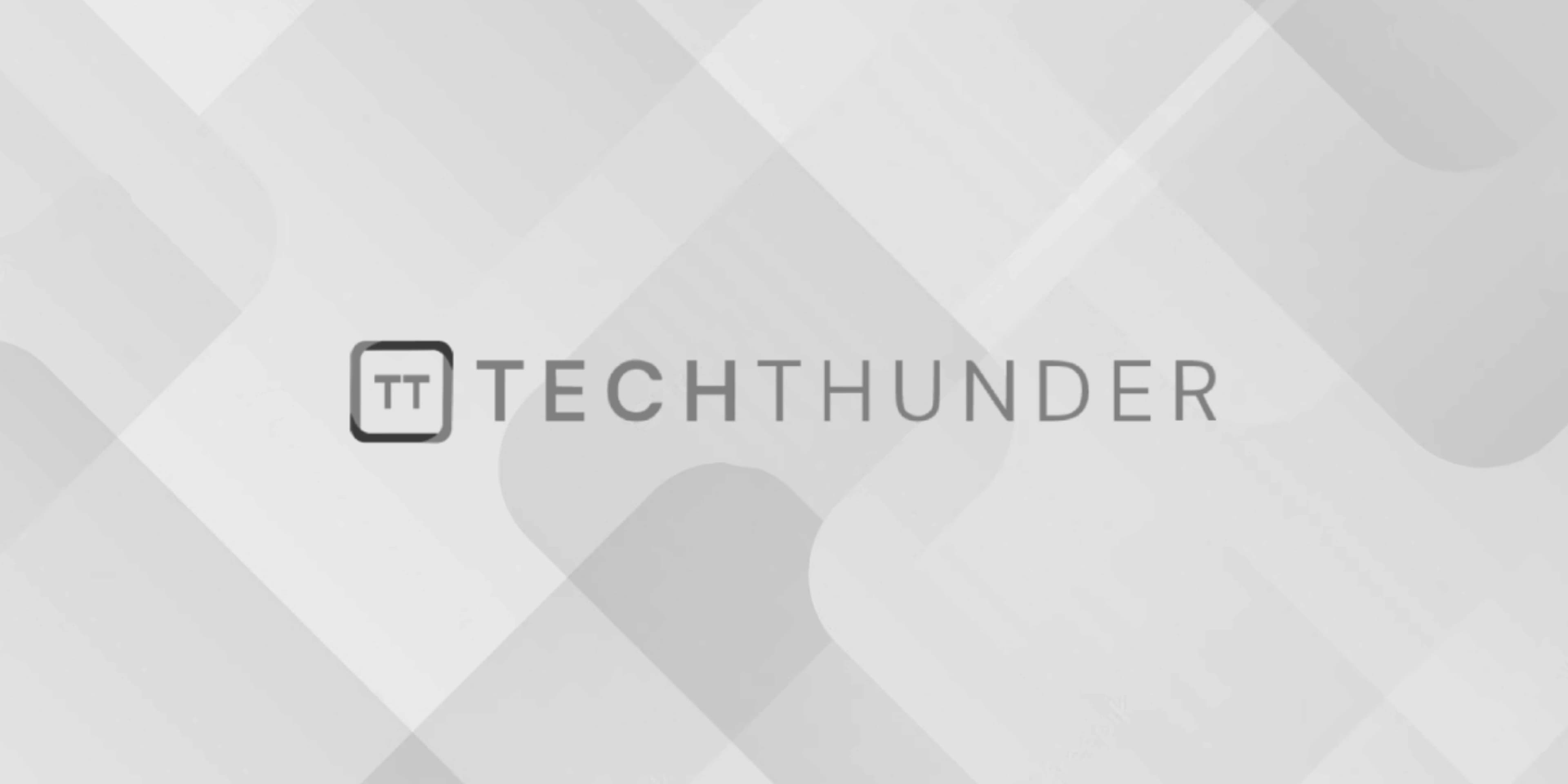
239 views
StringBuffer class
The Java StringBuffer
class is a part of the Java Standard Library and is used to create and manipulate mutable sequences of characters. Unlike the String
class, which is immutable (meaning its content cannot be changed after creation), the StringBuffer
class allows you to modify the characters in the sequence without creating a new object each time.
Here are some key features and methods of the StringBuffer
class:
- Mutable Character Sequence: A
StringBuffer
represents a mutable sequence of characters. You can add, modify, or remove characters from the sequence. - Thread-Safe Operations:
StringBuffer
is designed to be thread-safe, meaning it can be safely used in a multi-threaded environment. It achieves this thread safety through synchronization. However, this synchronization comes at a cost in terms of performance. - Performance Considerations: While
StringBuffer
is thread-safe, it’s not always the most efficient choice for single-threaded applications. If you don’t need thread safety, you can use theStringBuilder
class, which is similar toStringBuffer
but lacks synchronization, making it faster. - Constructors:
StringBuffer()
: Creates an emptyStringBuffer
with a default capacity.StringBuffer(int capacity)
: Creates an emptyStringBuffer
with the specified initial capacity.StringBuffer(String str)
: Creates aStringBuffer
initialized with the contents of the specified string.
- Modifying Operations:
append(data)
: Appends various data types to the end of the sequence.insert(index, data)
: Inserts data at the specified position.setCharAt(index, ch)
: Sets the character at the specified index.delete(start, end)
: Deletes characters from the start index (inclusive) to the end index (exclusive).deleteCharAt(index)
: Deletes the character at the specified index.replace(start, end, str)
: Replaces the characters in the specified range with the contents of the provided string.
- Capacity Management:
capacity()
: Returns the current capacity of theStringBuffer
.ensureCapacity(minimumCapacity)
: Ensures that theStringBuffer
has at least the specified capacity.trimToSize()
: Reduces the capacity of theStringBuffer
to its current length.
- String Conversion:
toString()
: Converts theStringBuffer
to aString
.
- Other Operations:
length()
: Returns the current length (number of characters) in theStringBuffer
.charAt(index)
: Returns the character at the specified index.subSequence(start, end)
: Returns a subsequence from the start index (inclusive) to the end index (exclusive).
Here’s an example of using StringBuffer
:
public class StringBufferExample {
public static void main(String[] args) {
StringBuffer sb = new StringBuffer("Hello, ");
sb.append("World!");
System.out.println(sb); // Output: Hello, World!
sb.insert(7, "Java ");
System.out.println(sb); // Output: Hello, Java World!
sb.replace(7, 11, "C++");
System.out.println(sb); // Output: Hello, C++ World!
sb.delete(7, 11);
System.out.println(sb); // Output: Hello, World!
}
}
The StringBuffer
is used to build and modify a mutable sequence of characters. You can see how the append
, insert
, replace
, and delete
methods are used to manipulate the content.