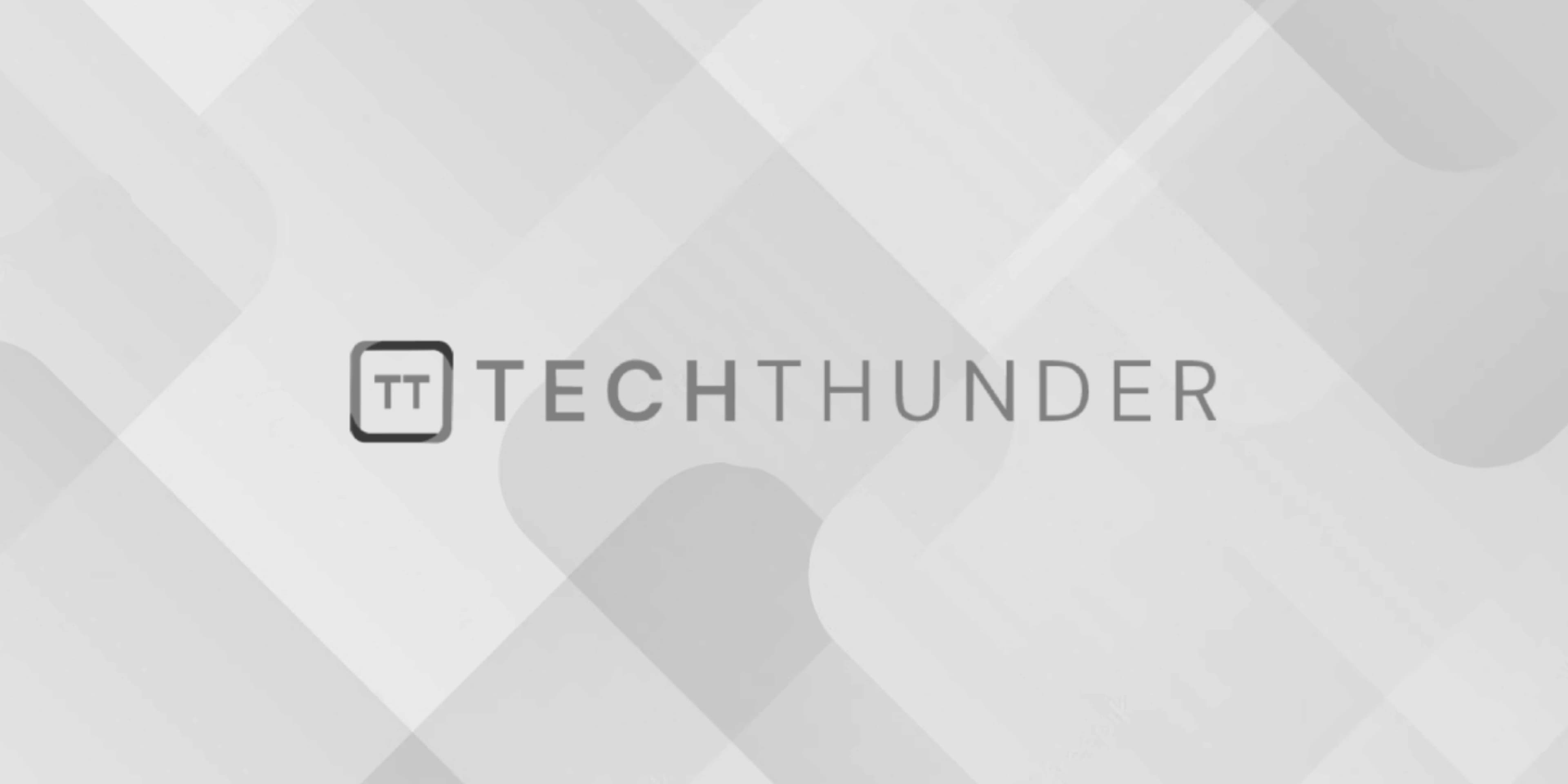
String split()
The Java split()
method is used to split a string into an array of substrings based on a specified delimiter or regular expression. It takes the delimiter or regular expression pattern as an argument and returns an array of strings obtained by splitting the original string at each occurrence of the delimiter or matching the regular expression pattern.
The basic syntax of the split()
method is as follows:
String[] substrings = originalString.split(delimiter);
originalString
: The original string that you want to split.delimiter
: A string or regular expression pattern that defines where to split the original string.
Here’s a simple example demonstrating the use of split()
with a delimiter:
public class StringSplitExample {
public static void main(String[] args) {
String input = "apple,banana,orange,grape";
String[] fruits = input.split(",");
for (String fruit : fruits) {
System.out.println(fruit);
}
}
}
In this example, the split()
method is used to split the input
string into an array of substrings, using a comma ,
as the delimiter. The resulting fruits
array contains the individual fruit names, and we iterate through the array to print them.
You can also use regular expressions as delimiters. For instance, if you want to split a string based on one or more spaces or tabs, you can use the regular expression pattern “\s+” to represent one or more whitespace characters:
String input = "This is a sample sentence.";
String[] words = input.split("\\s+");
for (String word : words) {
System.out.println(word);
}
The split("\\s+")
call splits the input
string into an array of words separated by one or more whitespace characters.
Keep in mind that the split()
method returns an array of substrings, and you can access these substrings using array indexing, as shown in the examples.