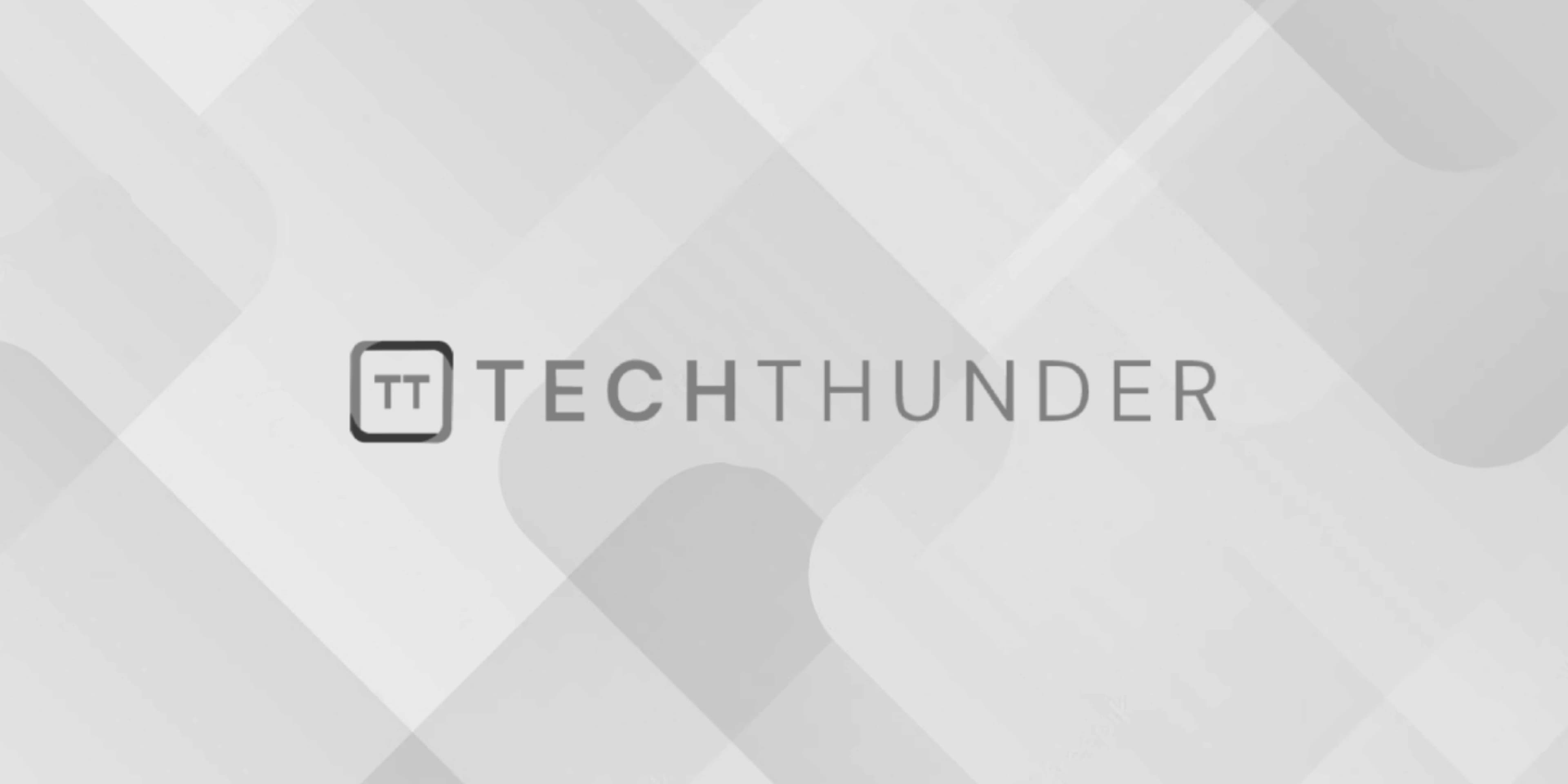
String contains()
The Java contains()
method is used to check whether a particular sequence of characters (substring) is present within a given string. It returns a boolean value, true
if the substring is found in the string, and false
otherwise. The basic syntax of the contains()
method is as follows:
boolean result = originalString.contains(substring);
originalString
: The string in which you want to check for the presence of a substring.substring
: The substring you want to check for within the original string.
Here’s an example of using the contains()
method:
public class ContainsExample {
public static void main(String[] args) {
String text = "The quick brown fox jumps over the lazy dog.";
String target = "fox";
boolean containsFox = text.contains(target);
if (containsFox) {
System.out.println("The string contains the word 'fox'.");
} else {
System.out.println("The string does not contain the word 'fox'.");
}
}
}
In this example, the contains()
method is used to check if the string text
contains the substring “fox.” Since “fox” is present in the original string, the result will be true
, and the program will print “The string contains the word ‘fox’.”
It’s important to note that the contains()
method performs a case-sensitive search. If you need to perform a case-insensitive search, you can convert both the original string and the substring to lowercase (or uppercase) using the toLowerCase()
method and then use contains()
:
String text = "The quick brown fox jumps over the lazy dog.";
String target = "FOX";
boolean containsFox = text.toLowerCase().contains(target.toLowerCase());
This modification makes the search case-insensitive, allowing “FOX” to be found in the original string, regardless of the case.