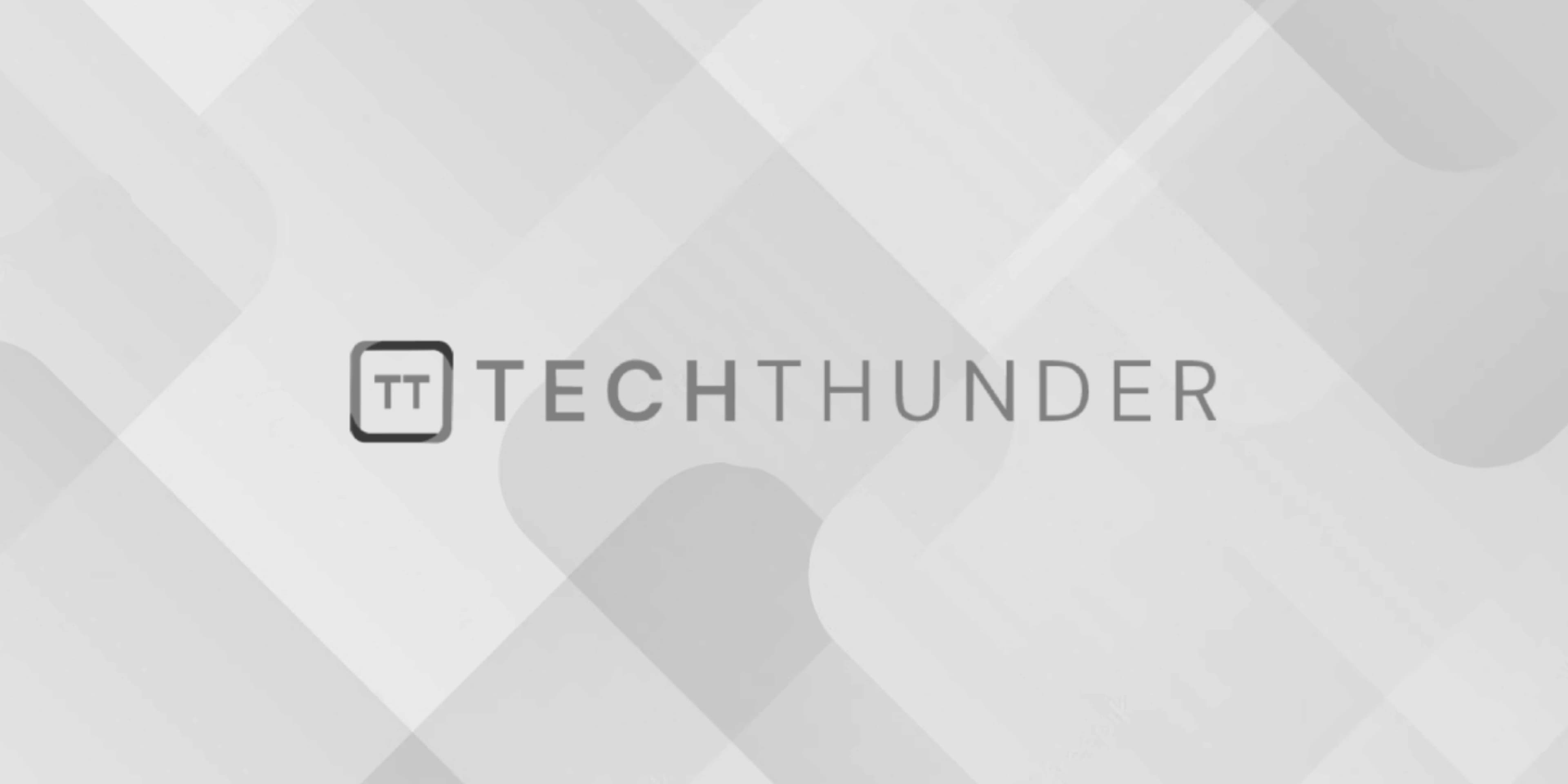
String intern()
The intern()
method in java is a part of the String
class and is used to optimize memory usage by adding the string to the pool of interned strings. This method returns a canonical representation of the string.
The basic syntax of the intern()
method is as follows:
String intern()
Here’s how you can use it:
String str1 = new String("hello");
String str2 = str1.intern();
In this example, the intern()
method is called on the string str1
, which creates a new string that is a canonical representation of the original string. If a string with the same content is already in the string pool, the intern()
method returns the reference to that existing string. If not, the method adds the string to the pool and returns the reference to the newly added string.
The main use case for intern()
is to save memory when working with many strings that have the same content. By interning strings, you ensure that there’s only one copy of each distinct string content in the string pool, which can significantly reduce memory consumption, especially when dealing with large amounts of data.
It’s important to note the following:
- The string pool is an area of memory managed by the Java Virtual Machine (JVM) where strings with the same content are stored as a single instance.
- The string pool is not a part of the regular heap memory used for object allocation, but rather a separate area for string interning.
- Calling
intern()
on a string can be useful when you have strings that are likely to be compared frequently (e.g., in hash maps or equality checks), as using interned strings can speed up these operations. - However, excessive use of
intern()
can also lead to increased memory usage and potential performance issues, so it should be used judiciously.
Here’s an example that illustrates how intern()
works:
String str1 = new String("hello");
String str2 = "hello";
String str3 = str1.intern();
System.out.println(str1 == str2); // false
System.out.println(str2 == str3); // true
In this example, str1
and str2
are different instances with the same content. After calling intern()
on str1
to intern it, str3
refers to the same interned string as str2
, so the comparison between str2
and str3
returns true
.