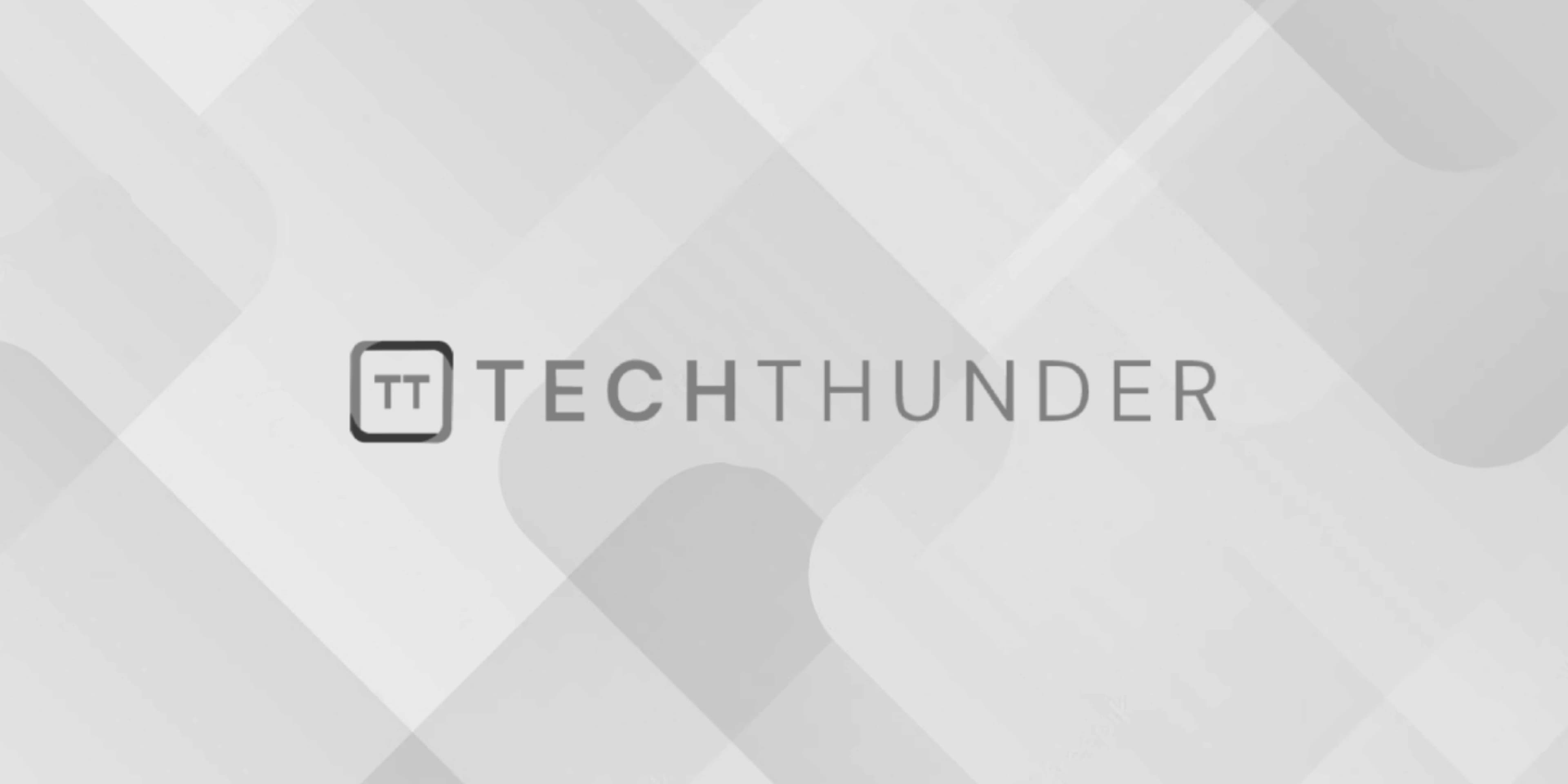
String indexOf()
The Java indexOf()
method is used to find the first occurrence of a specified substring within a string. It returns the index of the first occurrence of the substring in the original string, or -1 if the substring is not found. The method has multiple overloaded forms, allowing you to search for a substring starting from a specific index or using various search options.
The basic syntax of the indexOf()
method is as follows:
int index = originalString.indexOf(substring);
originalString
: The string in which you want to search for the substring.substring
: The substring you want to find within the original string.
Here’s an example of using the basic indexOf()
method:
public class IndexOfExample {
public static void main(String[] args) {
String text = "The quick brown fox jumps over the lazy dog.";
int index = text.indexOf("fox");
if (index != -1) {
System.out.println("The substring 'fox' is found at index " + index);
} else {
System.out.println("The substring 'fox' is not found in the text.");
}
}
}
In this example, the indexOf()
method is used to find the index of the first occurrence of the substring “fox” in the text
string. Since “fox” is present, the result will be the index at which “fox” starts in the string.
You can also use the overloaded versions of the indexOf()
method, which allow you to specify the starting index for the search, perform a backward search, or search for a single character, among other options.
Here are a few examples:
- Using
indexOf()
with a specified starting index:
int index = text.indexOf("fox", 10); // Start searching from index 10
- Using
lastIndexOf()
for a backward search:
int lastIndex = text.lastIndexOf("fox");
- Using
indexOf()
for searching a single character:
int charIndex = text.indexOf('j'); // Search for the character 'j'
The indexOf()
method is a versatile tool for finding substrings or characters within strings, and it’s commonly used for tasks like text processing, parsing, and searching.