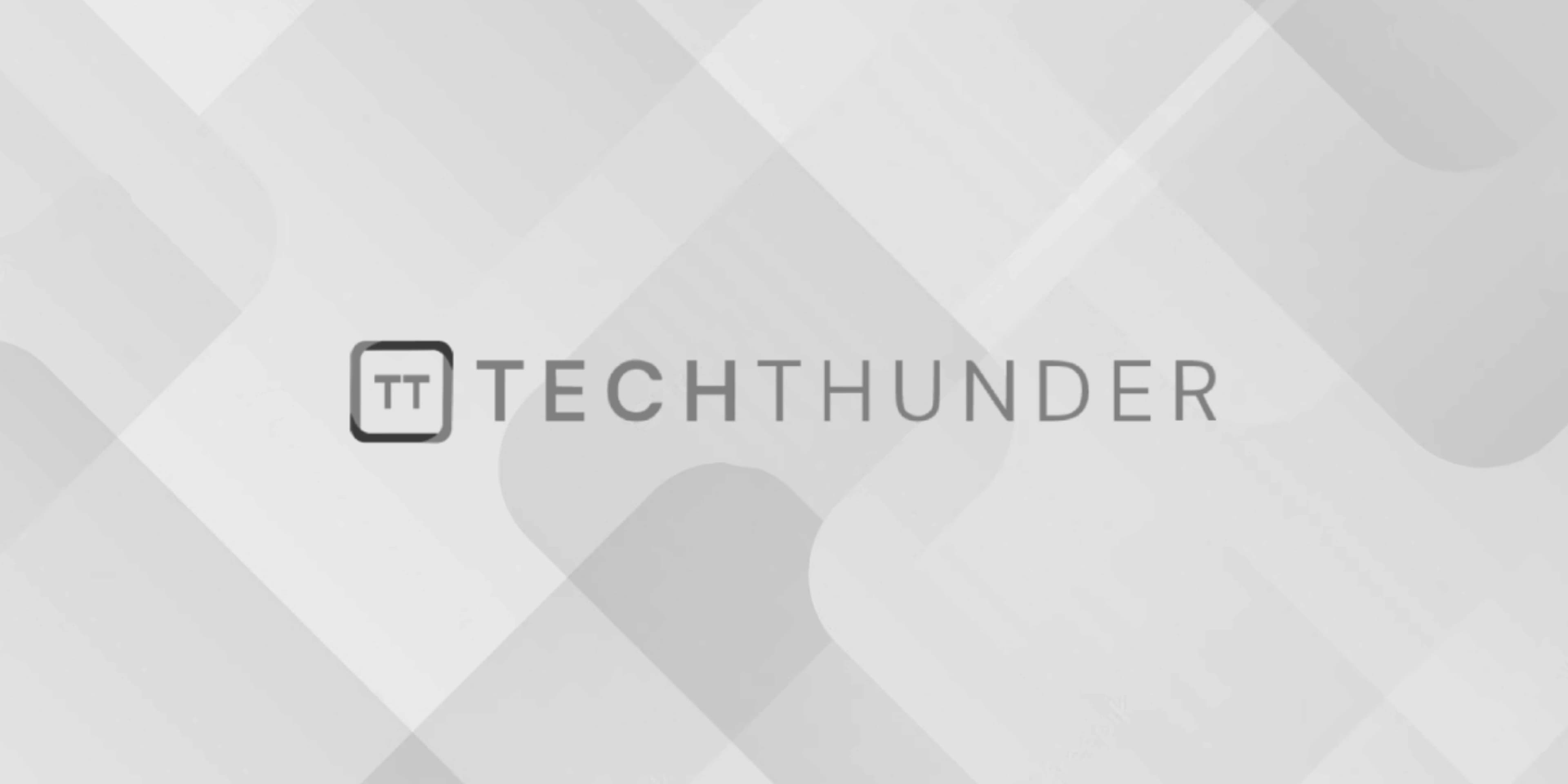
225 views
StringBuffer vs Builder
The Java has both StringBuffer
and StringBuilder
are used to create and manipulate mutable sequences of characters. They are similar in functionality and are both mutable, but the key difference between them is their thread safety:
- StringBuffer:
- Thread-Safe:
StringBuffer
is thread-safe, meaning it is designed to be used in multi-threaded environments without external synchronization. It achieves thread safety by adding synchronization to its methods. This ensures that multiple threads can safely access and modify aStringBuffer
object without data corruption. - Synchronization Overhead: While thread-safety is a valuable feature in multi-threaded applications, it comes at a cost of performance due to the synchronization overhead. In single-threaded applications, this synchronization is unnecessary and can lead to a slight performance penalty.
- Use Cases: Use
StringBuffer
when you need to perform string manipulations in a multi-threaded environment, and you want to ensure that multiple threads can safely work with the same object.
- StringBuilder:
- Not Thread-Safe:
StringBuilder
is not thread-safe, which means it does not provide built-in synchronization. This class is designed for single-threaded use. - No Synchronization Overhead: Because it lacks synchronization,
StringBuilder
is generally faster thanStringBuffer
. In single-threaded applications, it is the preferred choice for efficient string manipulations. - Use Cases: Use
StringBuilder
when you need to perform string manipulations in a single-threaded environment. If you are confident that your code will not be used in a multi-threaded context,StringBuilder
is typically the better choice due to its better performance.
In summary:
- Use
StringBuffer
when you need thread safety, and your application may be accessed by multiple threads concurrently. This ensures that the data is protected from concurrent modifications. - Use
StringBuilder
when thread safety is not a concern, and you want better performance, especially in single-threaded applications. It’s the preferred choice for most string manipulations in non-concurrent scenarios.
Both StringBuffer
and StringBuilder
offer similar methods for appending, inserting, deleting, and modifying characters in the sequence, making them versatile tools for working with mutable strings. Your choice between them should be based on your application’s threading requirements and performance considerations.