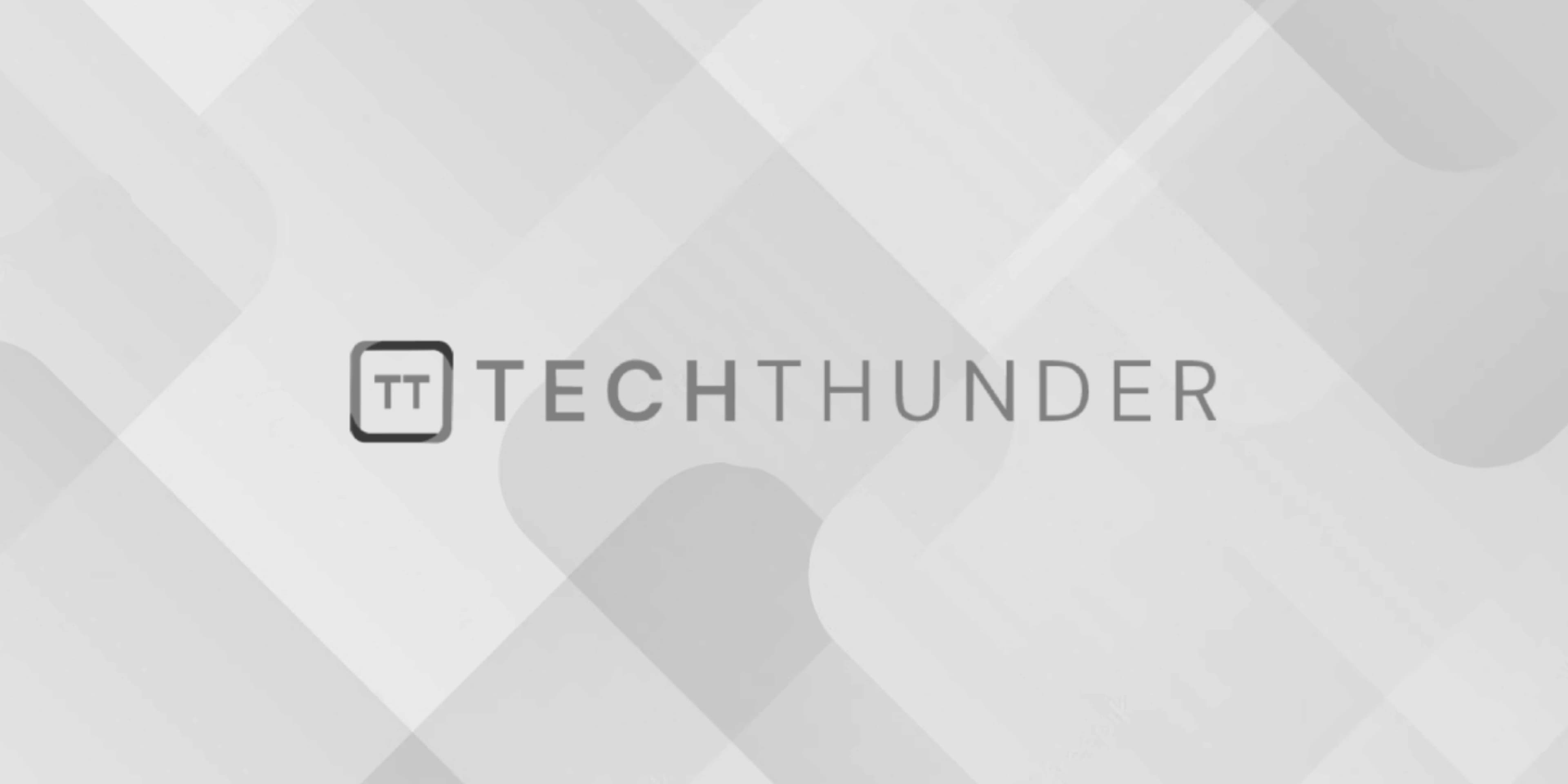
221 views
String Comparison
The Java can compare strings in several ways, depending on your specific needs. String comparison is a common task in programming, and Java provides multiple methods for comparing strings. Here are some common techniques:
- Using
equals()
Method: Theequals()
method is used to compare the content of two strings for equality. It returnstrue
if the strings have the same content andfalse
otherwise. This method performs a case-sensitive comparison.
String str1 = "Hello";
String str2 = "hello";
boolean areEqual = str1.equals(str2); // Returns false
- Using
equalsIgnoreCase()
Method: TheequalsIgnoreCase()
method is similar toequals()
, but it performs a case-insensitive comparison. It returnstrue
if the strings have the same content, regardless of case.
String str1 = "Hello";
String str2 = "hello";
boolean areEqual = str1.equalsIgnoreCase(str2); // Returns true
- Using
compareTo()
Method: ThecompareTo()
method is used for lexicographical comparison of strings. It returns an integer value:
- If the strings are equal, it returns
0
. - If the calling string is lexicographically less than the provided string, it returns a negative value.
- If the calling string is lexicographically greater than the provided string, it returns a positive value.
String str1 = "apple";
String str2 = "banana";
int result = str1.compareTo(str2); // Returns a negative value
- Using
compareToIgnoreCase()
Method: ThecompareToIgnoreCase()
method is similar tocompareTo()
, but it performs a case-insensitive lexicographical comparison.
String str1 = "apple";
String str2 = "Banana";
int result = str1.compareToIgnoreCase(str2); // Returns a positive value
- Using
==
Operator: The==
operator compares string references. It checks whether two string variables reference the same string object. It is not recommended for content comparison, as it checks object identity, not equality.
String str1 = "Hello";
String str2 = "Hello";
boolean areSame = (str1 == str2); // Returns true
- Using
equals()
andequalsIgnoreCase()
withnull
Checks: When comparing strings, it’s essential to handlenull
values gracefully. You should check fornull
before using theequals()
orequalsIgnoreCase()
methods to avoidNullPointerException
.
String str1 = "Hello";
String str2 = null;
boolean areEqual = str1 != null && str1.equals(str2); // Safe comparison
- Using
Objects.equals()
(Java 7 and later): TheObjects.equals()
method allows you to safely compare strings, handlingnull
values without causing exceptions.
String str1 = "Hello";
String str2 = null;
boolean areEqual = Objects.equals(str1, str2); // Safe comparison
The choice of string comparison method depends on your specific requirements. If you need to perform case-insensitive comparisons, use equalsIgnoreCase()
or compareToIgnoreCase()
. If you need lexicographical ordering, use compareTo()
or compareToIgnoreCase()
. Be cautious when using the ==
operator for string comparison, as it may not produce the expected results for content comparison.