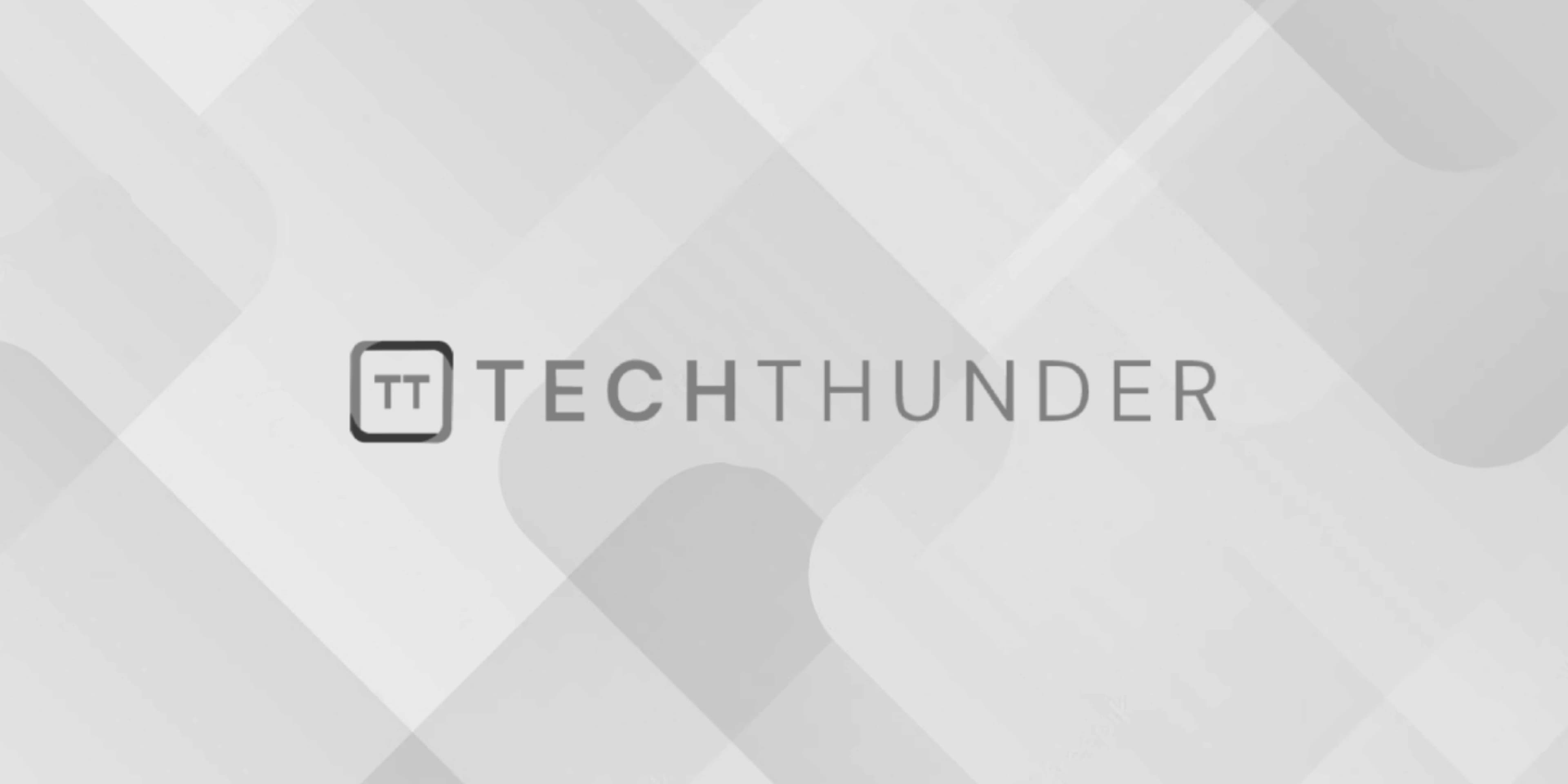
String valueOf()
The valueOf()
method in java is a static method that belongs to various classes and is used to convert different types of values into a string representation. It’s commonly used to convert primitive data types or objects into their string equivalents. This method is typically used when you want to concatenate a non-string value with a string, or when you need to convert a value to a string explicitly.
The valueOf()
method is available in several classes, such as:
- java.lang.String: This class provides the
valueOf()
method to convert various types to a string.
int number = 42;
String str = String.valueOf(number); // Converts int to "42"
- java.lang.Boolean: Used to convert boolean values to strings.
boolean boolValue = true;
String boolStr = Boolean.valueOf(boolValue); // Converts boolean to "true"
- java.lang.Double and java.lang.Float: Used to convert double and float values to strings.
double doubleValue = 3.14159;
String doubleStr = Double.valueOf(doubleValue); // Converts double to "3.14159"
float floatValue = 2.71828f;
String floatStr = Float.valueOf(floatValue); // Converts float to "2.71828"
- Other Wrapper Classes (Integer, Long, Short, Byte, Character): All these classes provide a
valueOf()
method to convert their respective primitive types to strings.
char charValue = 'A';
String charStr = Character.valueOf(charValue); // Converts char to "A"
The valueOf()
method is quite useful when you need to concatenate a non-string value with a string or when you need to pass a string representation of an object to other methods that accept strings. Keep in mind that Java’s automatic type conversion might also handle such conversions implicitly in many situations.