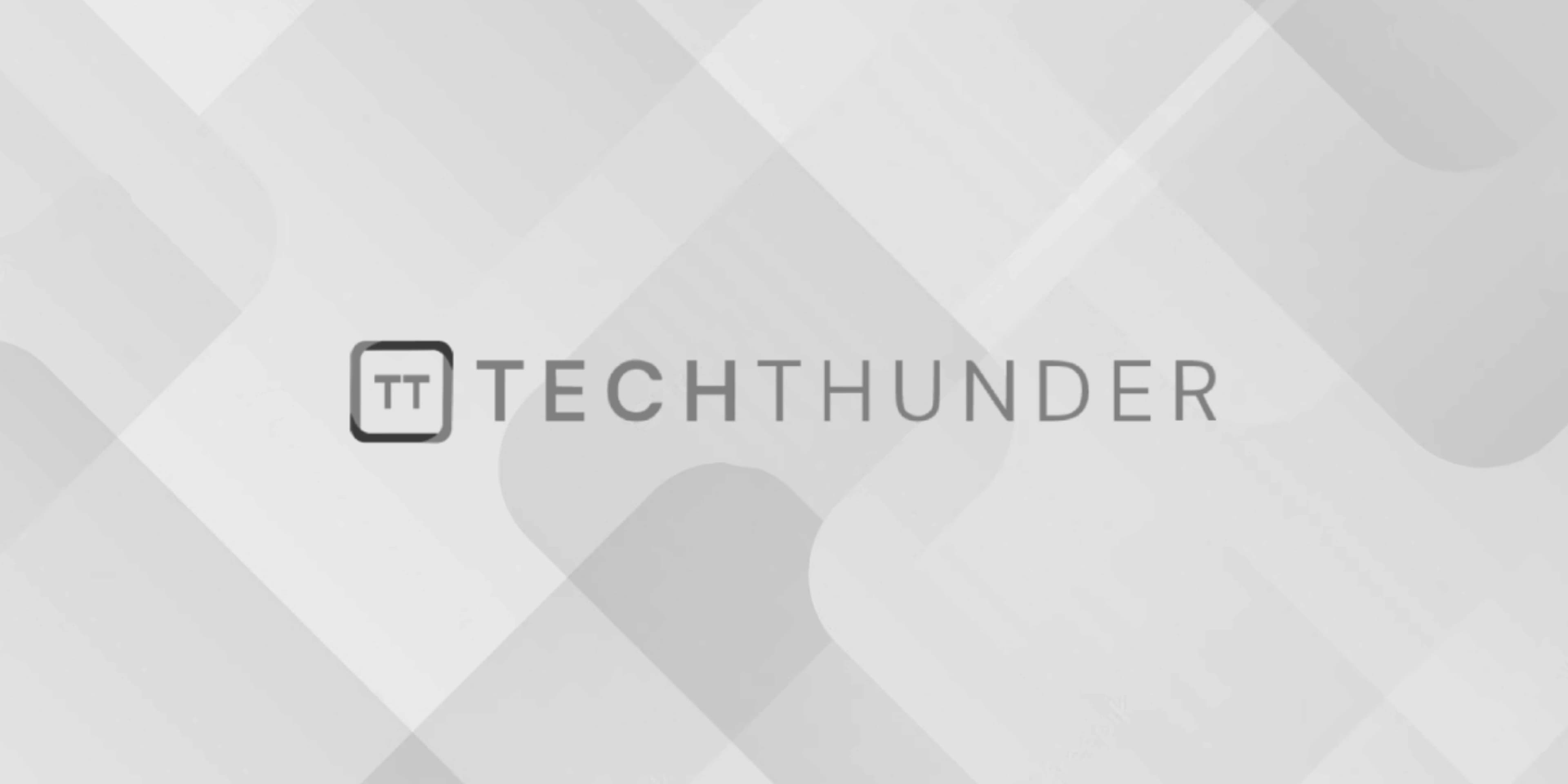
String startsWith()
The startsWith()
method in java is a part of the String
class and is used to check whether a given string starts with a specified prefix. It returns a boolean value indicating whether the string begins with the specified prefix.
The basic syntax of the startsWith()
method is as follows:
boolean startsWith(String prefix)
Here’s how you can use it:
String str = "Hello, world!";
boolean startsWithHello = str.startsWith("Hello"); // true
boolean startsWithHi = str.startsWith("Hi"); // false
In this example, the startsWith()
method is called on the string str
to check whether it starts with the prefixes “Hello” and “Hi”. The method returns true
for the “Hello” prefix and false
for the “Hi” prefix.
Additionally, the startsWith()
method has an overloaded version that allows you to specify an offset index from which to start checking the prefix:
boolean startsWith(String prefix, int offset)
Here’s how the offset version can be used:
String str = "This is a test";
boolean startsWithIs = str.startsWith("is", 2); // true
boolean startsWithTest = str.startsWith("test", 10); // true
In this example, the startsWith()
method is used with an offset of 2 and 10, respectively, to check for prefixes “is” and “test” in the given string, starting from the specified indices.
Keep in mind that the startsWith()
method performs a case-sensitive comparison. If you need a case-insensitive comparison, you can first convert the string and the prefix to lowercase or uppercase using methods like toLowerCase()
or toUpperCase()
before calling startsWith()
.