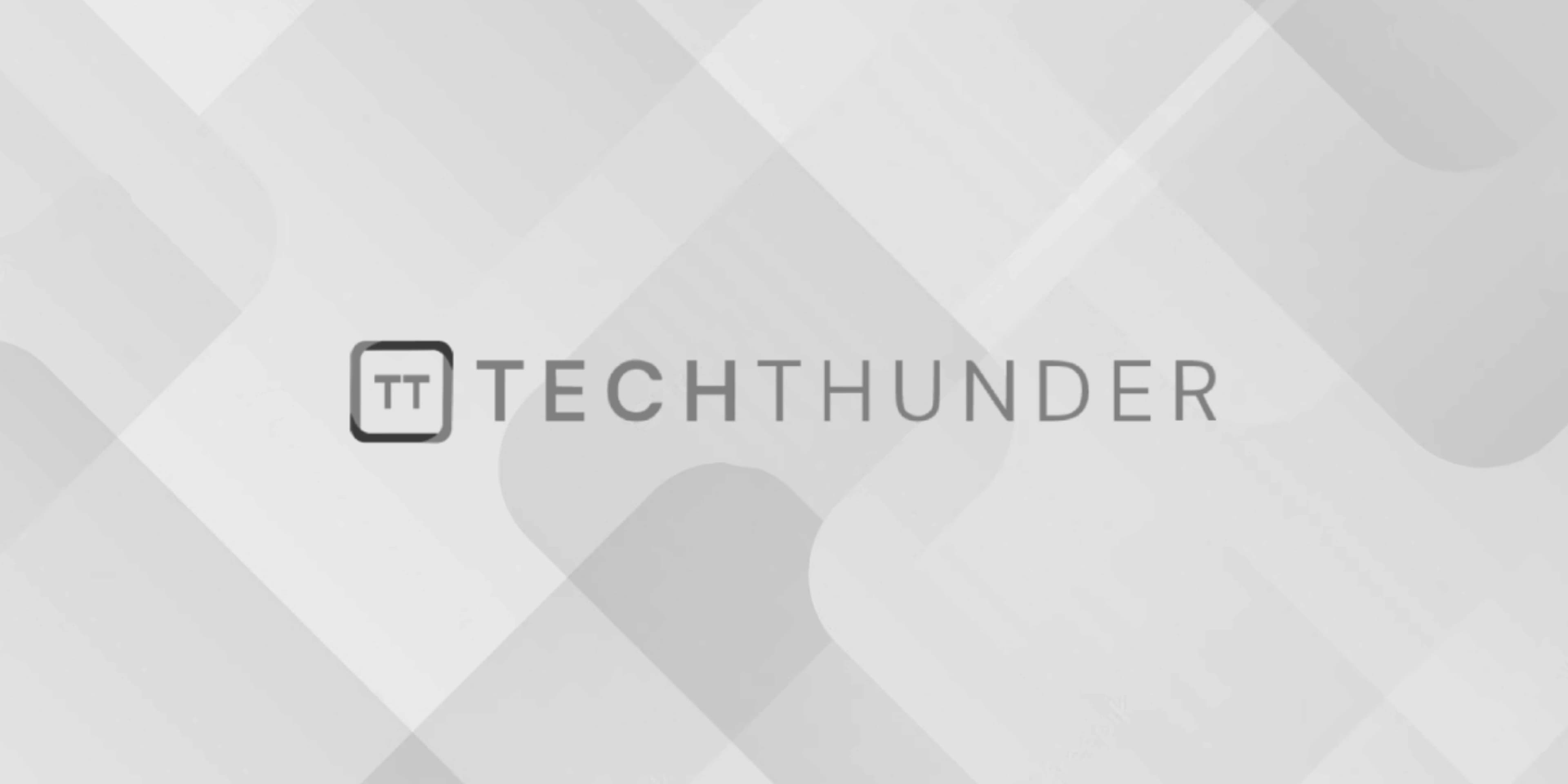
String replace()
The replace()
method in java is a part of the String
class and is used to create a new string by replacing occurrences of a specified character or substring with another character or substring. The method returns a new string with the replacements made.
The basic syntax of the replace()
method is as follows:
String replace(char oldChar, char newChar)
String replace(CharSequence target, CharSequence replacement)
Here’s how you can use it:
- Replacing a character:
String original = "Hello, world!";
String replaced = original.replace('o', '0'); // "Hell0, w0rld!"
In this example, the replace('o', '0')
call replaces all occurrences of the character ‘o’ with the character ‘0’, resulting in the string “Hell0, w0rld!”.
- Replacing a substring:
String original = "Hello, world!";
String replaced = original.replace("world", "Java"); // "Hello, Java!"
In this example, the replace("world", "Java")
call replaces the substring “world” with the substring “Java”, resulting in the string “Hello, Java!”.
Keep in mind:
- The
replace()
method creates a new string with the replacements made. The original string remains unchanged. - The replacements are case-sensitive. If you need to perform case-insensitive replacements, you’ll need to use additional logic or regular expressions.
- For both
replace()
methods, you can replace occurrences of characters or substrings. - The first
replace()
method replaces individual characters, while the second method replaces entire substrings.
Here are a few more examples:
String text = "aabbcc";
String replaced1 = text.replace('a', 'x'); // "xxbbcc"
String replaced2 = text.replace("bb", "yy"); // "aayycc"
In these examples, replaced1
replaces occurrences of ‘a’ with ‘x’, resulting in “xxbbcc”. replaced2
replaces occurrences of “bb” with “yy”, resulting in “aayycc”.