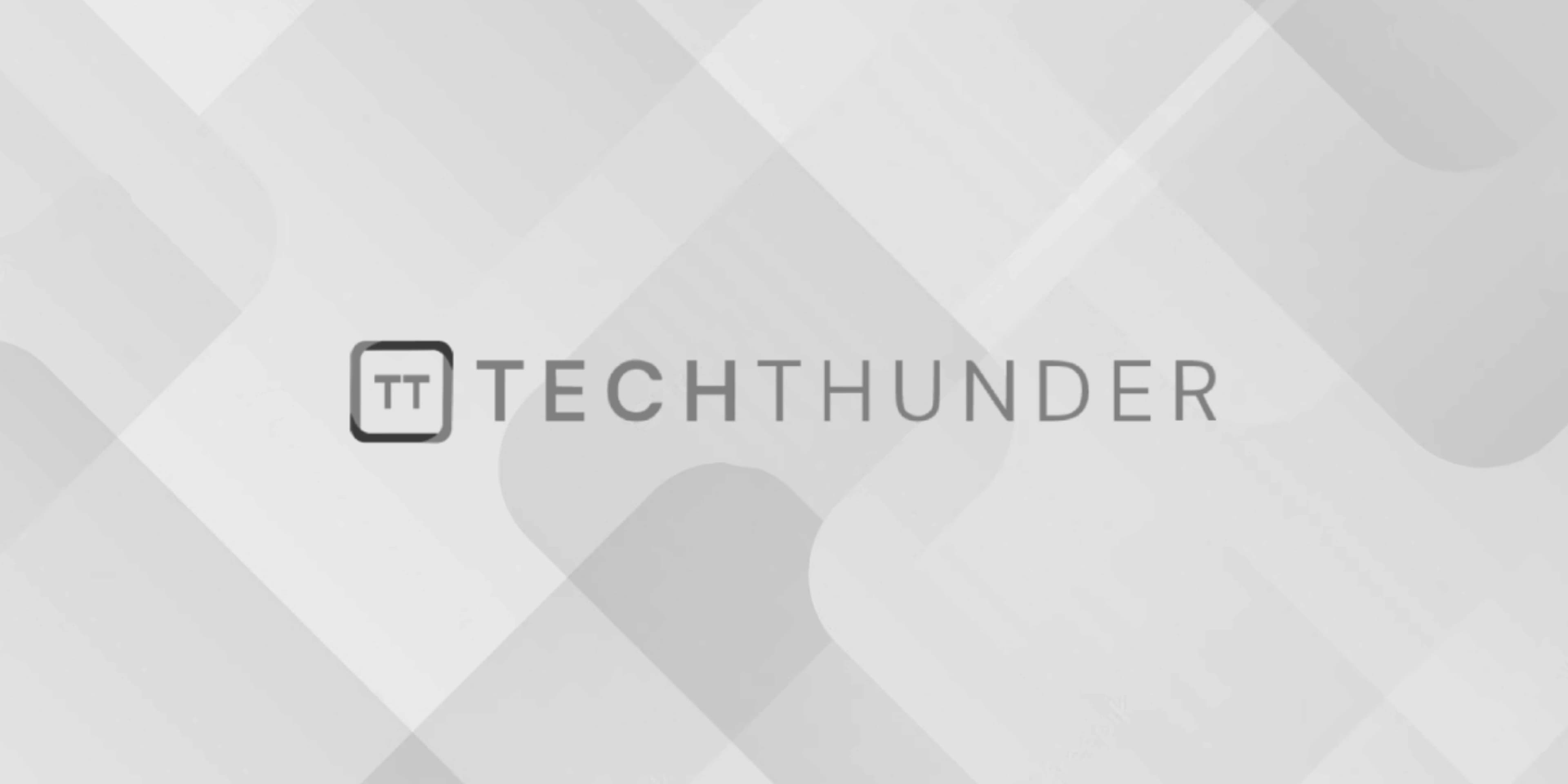
String join()
The String.join()
method in java is a static method introduced in Java 8 as part of the java.lang.String
class. It’s used to join together multiple strings, often with a delimiter in between them, to form a single string. This is particularly useful when you want to concatenate a list of strings into a single, formatted string.
The basic syntax of the String.join()
method is as follows:
static String join(CharSequence delimiter, CharSequence... elements)
Here’s how you can use it:
String[] words = {"Hello", "world", "from", "Java"};
String joinedString = String.join(" ", words); // "Hello world from Java"
In this example, the String.join()
method is used to join the strings in the words
array with a space delimiter between them, resulting in the string “Hello world from Java”.
Key points to note:
- The
delimiter
parameter is the character sequence that will be inserted between the elements being joined. - The
elements
parameter is a varargs parameter that represents the strings you want to join. - The method returns a new string that’s the result of joining the elements with the specified delimiter.
You can use String.join()
to join any type of CharSequence
objects, not just strings. For example:
List<String> list = Arrays.asList("apple", "banana", "orange");
String joinedFruits = String.join(", ", list); // "apple, banana, orange"
In this example, a list of strings is joined with a comma and space delimiter to create the string “apple, banana, orange”.
Remember that while String.join()
is convenient for simple cases, for more complex string concatenations or formatting, you might want to consider using the StringBuilder
class or other string formatting mechanisms.