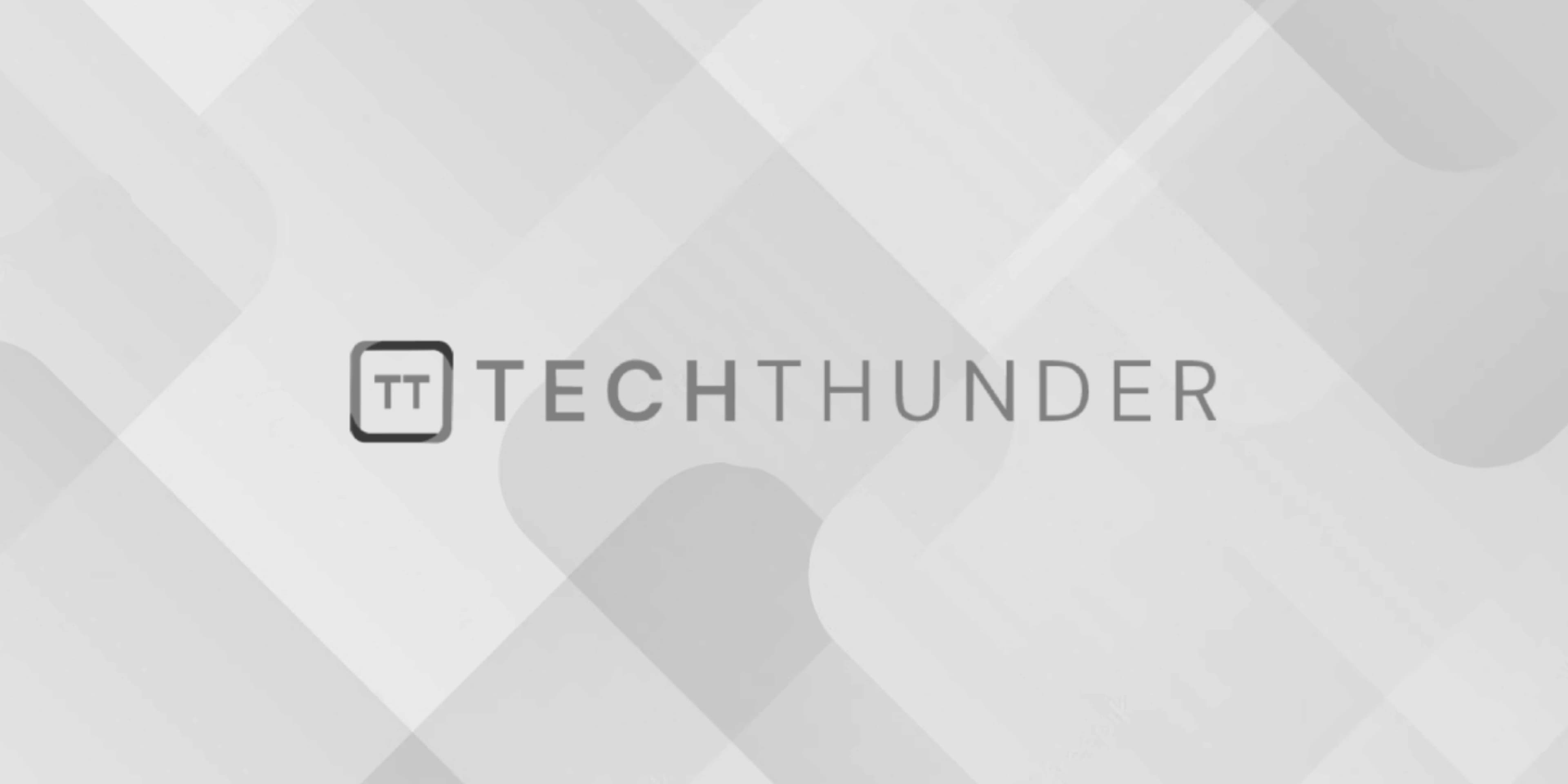
String charAt()
The Java charAt(int index)
method is used to retrieve the character located at a specific index within a string. The index
parameter is an integer that represents the position of the character you want to access, with the first character at index 0, the second character at index 1, and so on.
The basic syntax of the charAt()
method is as follows:
char charAtIndex = originalString.charAt(index);
originalString
: The original string from which you want to retrieve the character.index
: The position (index) of the character you want to access.
Here’s a simple example of using the charAt()
method:
public class CharAtExample {
public static void main(String[] args) {
String text = "Hello, World!";
char firstChar = text.charAt(0);
char fifthChar = text.charAt(4);
System.out.println("First character: " + firstChar);
System.out.println("Fifth character: " + fifthChar);
}
}
In this example, the charAt()
method is used to retrieve the first character of the text
string (which is ‘H’) and the fifth character (which is ‘,’), and the results are printed.
It’s important to note that the charAt()
method throws an IndexOutOfBoundsException
if you provide an index that is out of the valid range (i.e., negative index or an index greater than or equal to the length of the string). Therefore, it’s a good practice to ensure that the index is within the valid range before using the charAt()
method to avoid exceptions.
For example:
String text = "Hello, World!";
int index = 12; // Index exceeds the length of the string
if (index >= 0 && index < text.length()) {
char charAtIndex = text.charAt(index);
System.out.println("Character at index " + index + ": " + charAtIndex);
} else {
System.out.println("Invalid index.");
}
To check whether the index is within the valid range before calling charAt()
. This helps prevent an exception from being thrown.