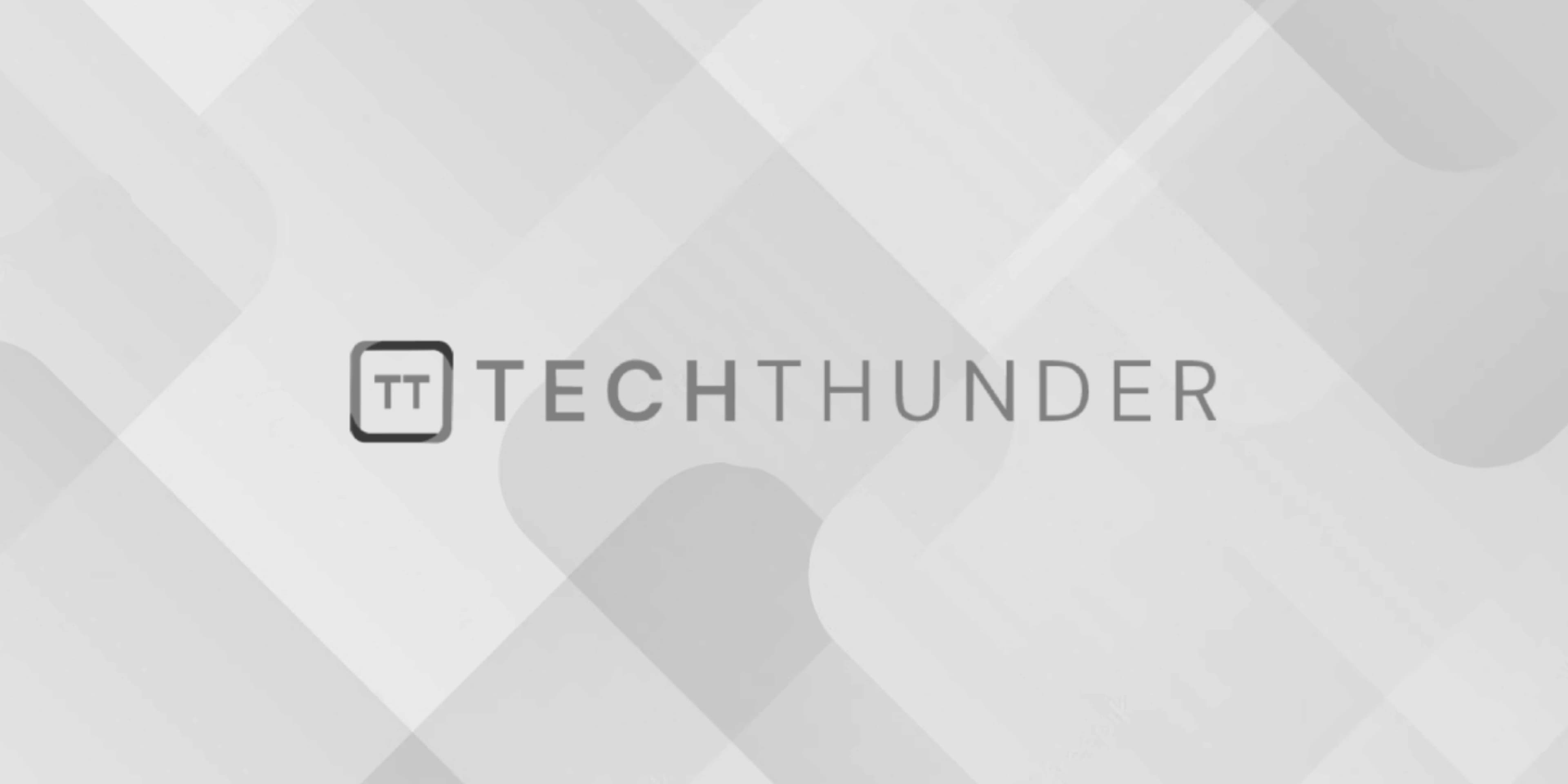
176 views
StringBuilder class
The Java StringBuilder
class is used to create and manipulate mutable sequences of characters, just like the StringBuffer
class. Both StringBuilder
and StringBuffer
are designed for the same purpose, but they have different characteristics:
StringBuilder
is not thread-safe, meaning it is not synchronized for multi-threaded operations. This lack of synchronization makesStringBuilder
more efficient in single-threaded applications.
Here are some key features and methods of the StringBuilder
class:
- Mutable Character Sequence: A
StringBuilder
represents a mutable sequence of characters. You can add, modify, or remove characters from the sequence. - Not Thread-Safe: Unlike
StringBuffer
,StringBuilder
is not thread-safe. This means that if multiple threads access aStringBuilder
concurrently, external synchronization is required to avoid issues. - Performance Considerations:
StringBuilder
is typically preferred in single-threaded scenarios due to its improved performance compared toStringBuffer
. However, if you require thread safety, you should useStringBuffer
. - Constructors:
StringBuilder()
: Creates an emptyStringBuilder
with a default initial capacity.StringBuilder(int capacity)
: Creates an emptyStringBuilder
with the specified initial capacity.StringBuilder(String str)
: Creates aStringBuilder
initialized with the contents of the specified string.
- Modifying Operations:
append(data)
: Appends various data types to the end of the sequence.insert(index, data)
: Inserts data at the specified position.setCharAt(index, ch)
: Sets the character at the specified index.delete(start, end)
: Deletes characters from the start index (inclusive) to the end index (exclusive).deleteCharAt(index)
: Deletes the character at the specified index.replace(start, end, str)
: Replaces the characters in the specified range with the contents of the provided string.
- Capacity Management:
capacity()
: Returns the current capacity of theStringBuilder
.ensureCapacity(minimumCapacity)
: Ensures that theStringBuilder
has at least the specified capacity.trimToSize()
: Reduces the capacity of theStringBuilder
to its current length.
- String Conversion:
toString()
: Converts theStringBuilder
to aString
.
- Other Operations:
length()
: Returns the current length (number of characters) in theStringBuilder
.charAt(index)
: Returns the character at the specified index.subSequence(start, end)
: Returns a subsequence from the start index (inclusive) to the end index (exclusive).
Here’s an example of using StringBuilder
:
public class StringBuilderExample {
public static void main(String[] args) {
StringBuilder sb = new StringBuilder("Hello, ");
sb.append("World!");
System.out.println(sb); // Output: Hello, World!
sb.insert(7, "Java ");
System.out.println(sb); // Output: Hello, Java World!
sb.replace(7, 11, "C++");
System.out.println(sb); // Output: Hello, C++ World!
sb.delete(7, 11);
System.out.println(sb); // Output: Hello, World!
}
}
The StringBuilder
is used to build and modify a mutable sequence of characters. You can see how the append
, insert
, replace
, and delete
methods are used to manipulate the content.