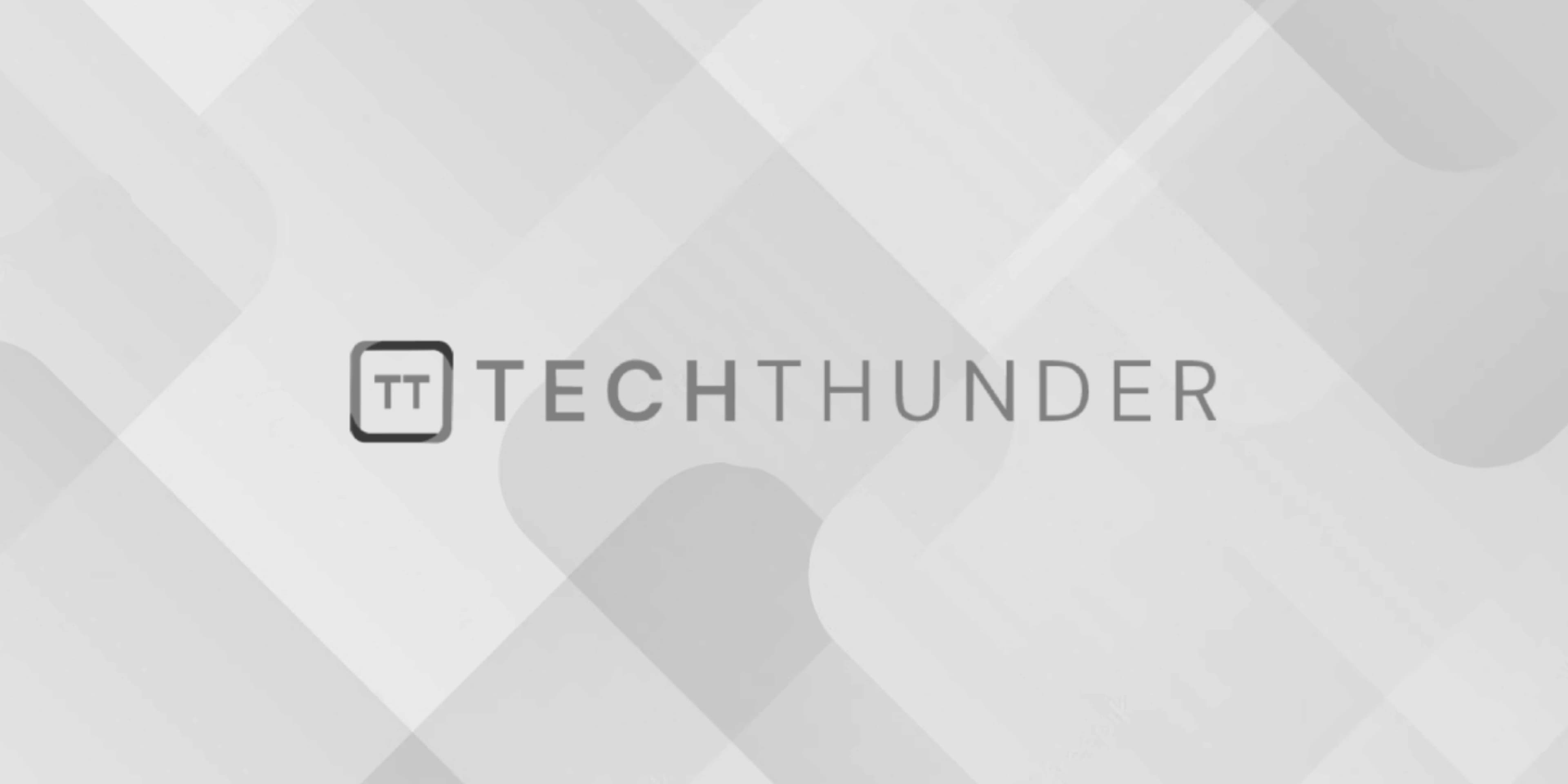
String replaceAll()
The Java replaceAll()
method is a part of the java.lang.String
class, and it is used to replace all occurrences of a specified substring (or regular expression) in the original string with a new string. It returns a new string with all replacements applied while leaving the original string unchanged. The basic syntax of the replaceAll()
method is as follows:
String replacedString = originalString.replaceAll(regex, replacement);
originalString
: The original string in which you want to perform replacements.regex
: A regular expression or a plain substring to be searched for and replaced.replacement
: The string that will replace the matched substring or regular expression.
Here’s a simple example demonstrating the use of replaceAll()
to replace all occurrences of a substring in a string:
public class StringReplaceAllExample {
public static void main(String[] args) {
String originalString = "The quick brown fox jumps over the lazy dog.";
String replacedString = originalString.replaceAll("fox", "cat");
System.out.println("Original String: " + originalString);
System.out.println("Replaced String: " + replacedString);
}
}
In this example, the replaceAll()
method is used to replace all occurrences of the substring “fox” with “cat” in the originalString
. The original string remains unchanged, and the modified string is stored in replacedString
.
Keep in mind that the first argument, regex
, can also be a regular expression pattern. For example, if you want to replace all digits in a string with “X”, you can use a regular expression like this:
String originalString = "123-456-7890";
String replacedString = originalString.replaceAll("\\d", "X");
The regular expression \\d
matches all digits, and they are replaced with “X”. The \\
is an escape character in Java, and it’s used to represent a single backslash in the regular expression pattern.