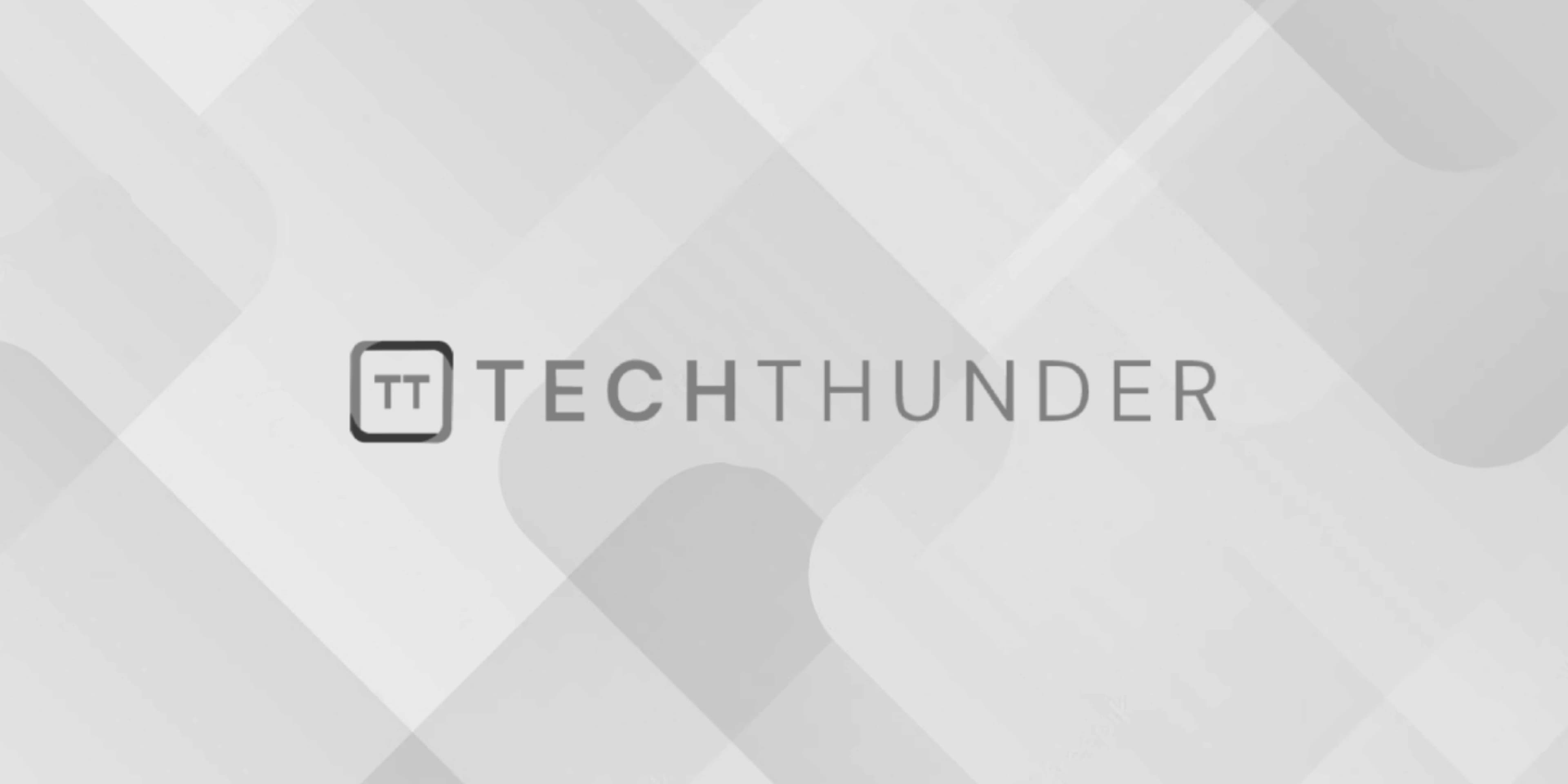
toString method
The Java toString()
method is a built-in method provided by the Object
class and is available for all objects. It is used to obtain a string representation of an object. The default implementation of toString()
in the Object
class returns a string containing the class name and the object’s hash code.
However, it is often a good practice to override the toString()
method in your own classes to provide a meaningful and human-readable representation of the object’s state. This is especially useful for debugging and logging purposes.
To override the toString()
method, follow these steps:
- Add the
toString()
method to your class, typically by providing your own implementation. - Inside the
toString()
method, construct a string that represents the object’s state or properties. - Return the constructed string.
Here’s an example of overriding the toString()
method in a custom class:
public class Person {
private String name;
private int age;
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public String toString() {
return "Person{name='" + name + "', age=" + age + '}';
}
}
In this example, the Person
class has overridden the toString()
method to provide a custom string representation of a Person
object. The overridden method returns a string containing the person’s name and age.
You can then use the toString()
method to obtain a string representation of a Person
object like this:
Person person = new Person("Alice", 30);
String personInfo = person.toString();
System.out.println(personInfo); // Output: Person{name='Alice', age=30}
It’s a good practice to provide a meaningful toString()
method for your custom classes because it makes debugging and logging easier, as you can quickly see the state of objects in a human-readable format.