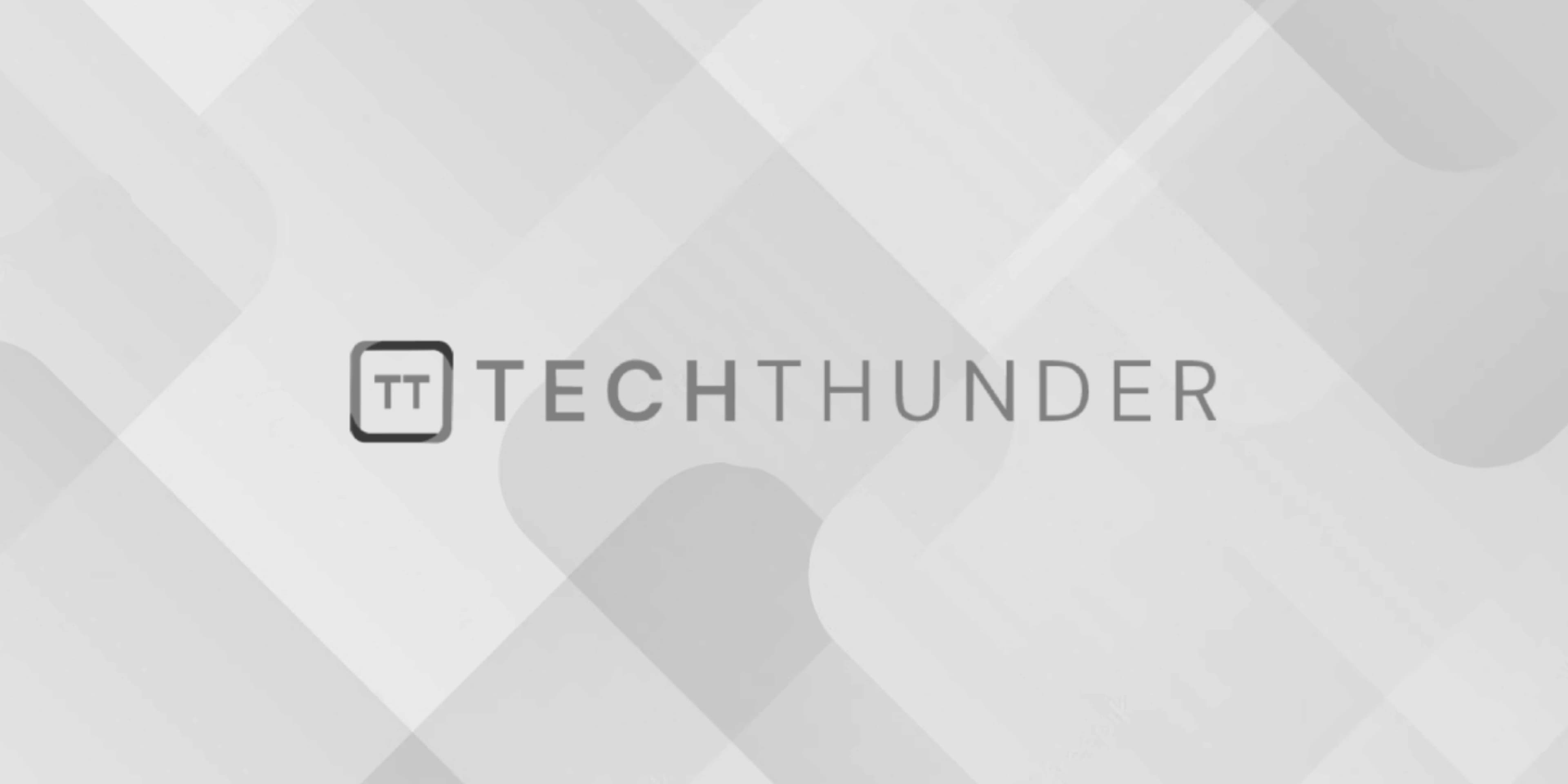
String toLowerCase()
The toLowerCase()
method is a part of the String
class and is used to convert all characters of a string to lowercase. This method returns a new string with all characters converted to lowercase while leaving any characters that are already lowercase unchanged.
The basic syntax of the toLowerCase()
method is as follows:
String toLowerCase()
Here’s how you can use it:
String original = "Hello World";
String lowercase = original.toLowerCase(); // "hello world"
In this example, the toLowerCase()
method is called on the string "Hello World"
, and it returns a new string with all characters converted to lowercase.
Keep in mind the following:
- The
toLowerCase()
method doesn’t modify the original string. Instead, it creates a new string with the lowercase representation of the characters. - This method uses the default locale’s rules for converting characters to lowercase. If you need to perform case conversion according to a specific locale, you can use the overloaded version of this method that accepts a
Locale
argument:
String toLowerCase(Locale locale)
For example:
String original = "Übermensch";
String lowercase = original.toLowerCase(Locale.GERMAN); // "übermensch"
- Some characters might have multiple lowercase equivalents in different locales. The conversion behavior might differ based on the rules of the locale.
Here are a few more examples:
String str1 = "Java";
String str2 = "LoWerCaSe";
String str3 = "ThIs Is A MixED CaSe StRiNg";
String lowercase1 = str1.toLowerCase(); // "java"
String lowercase2 = str2.toLowerCase(); // "lowercase"
String lowercase3 = str3.toLowerCase(); // "this is a mixed case string"
The toLowerCase()
method is particularly useful when you need to perform case-insensitive string comparisons or when you want to ensure consistent case usage in your application, regardless of the input.