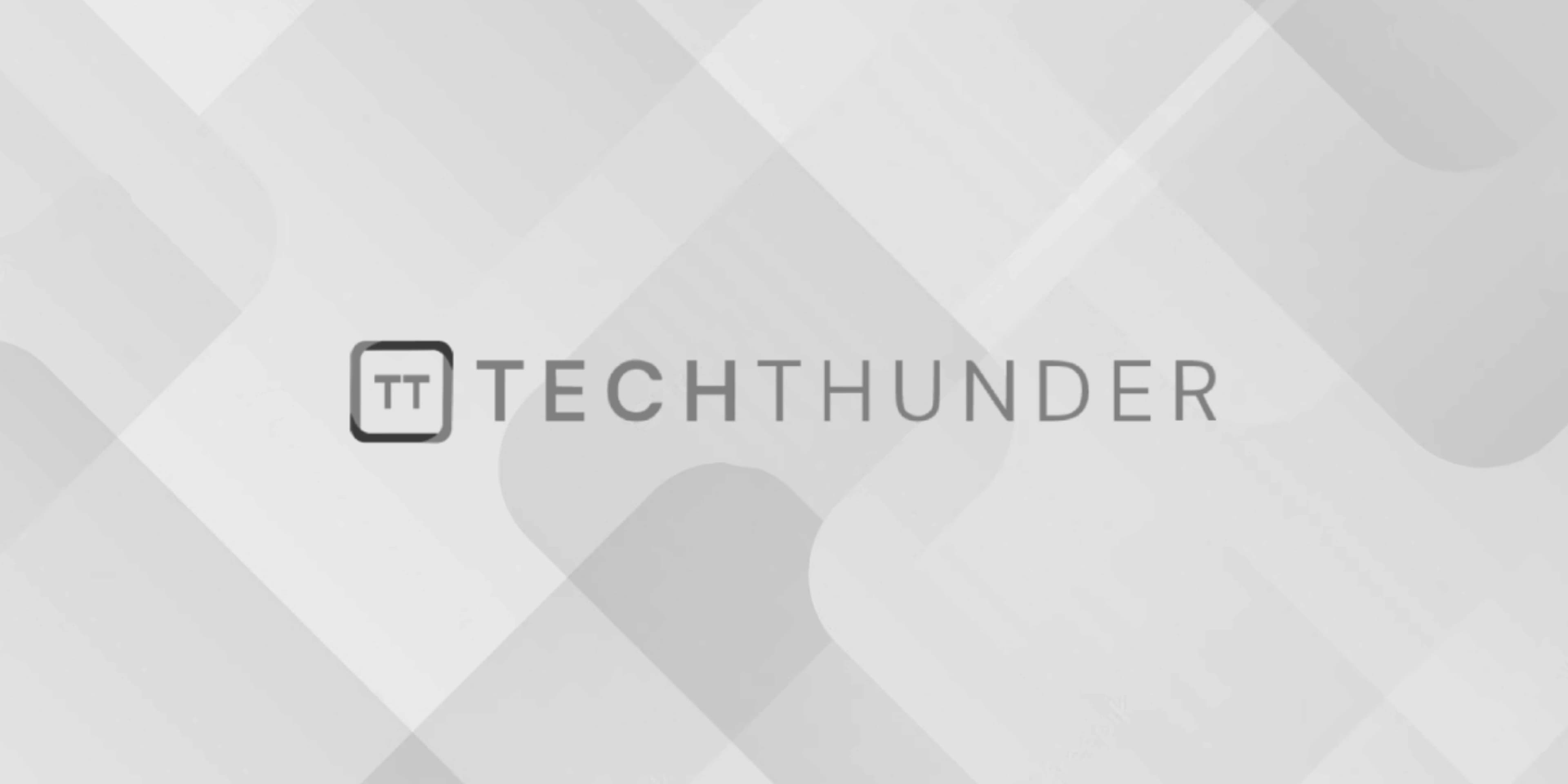
String toCharArray()
The toCharArray()
method in java is a part of the String
class and is used to convert a string into an array of characters. This method returns a new character array that contains the characters of the string in the same order as they appear in the string.
The basic syntax of the toCharArray()
method is as follows:
char[] toCharArray()
Here’s how you can use it:
String str = "Hello";
char[] charArray = str.toCharArray();
In this example, the toCharArray()
method is called on the string "Hello"
, and it returns a character array containing ['H', 'e', 'l', 'l', 'o']
.
You can use the resulting character array just like any other array in Java. For example, you can iterate over the characters or access specific characters using array indices:
for (char c : charArray) {
System.out.print(c + " "); // Output: H e l l o
}
char firstChar = charArray[0]; // 'H'
char secondChar = charArray[1]; // 'e'
Keep in mind that the toCharArray()
method creates a new character array, so it incurs a memory allocation cost. If you only need to iterate through the characters of a string without modifying them, you can also achieve this using the charAt()
method in combination with the string’s length:
String str = "Hello";
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
System.out.print(c + " "); // Output: H e l l o
}
Both approaches accomplish the same task of accessing the characters of a string, but toCharArray()
provides a convenient way to work with the characters as an array if you need to perform more complex operations on them.