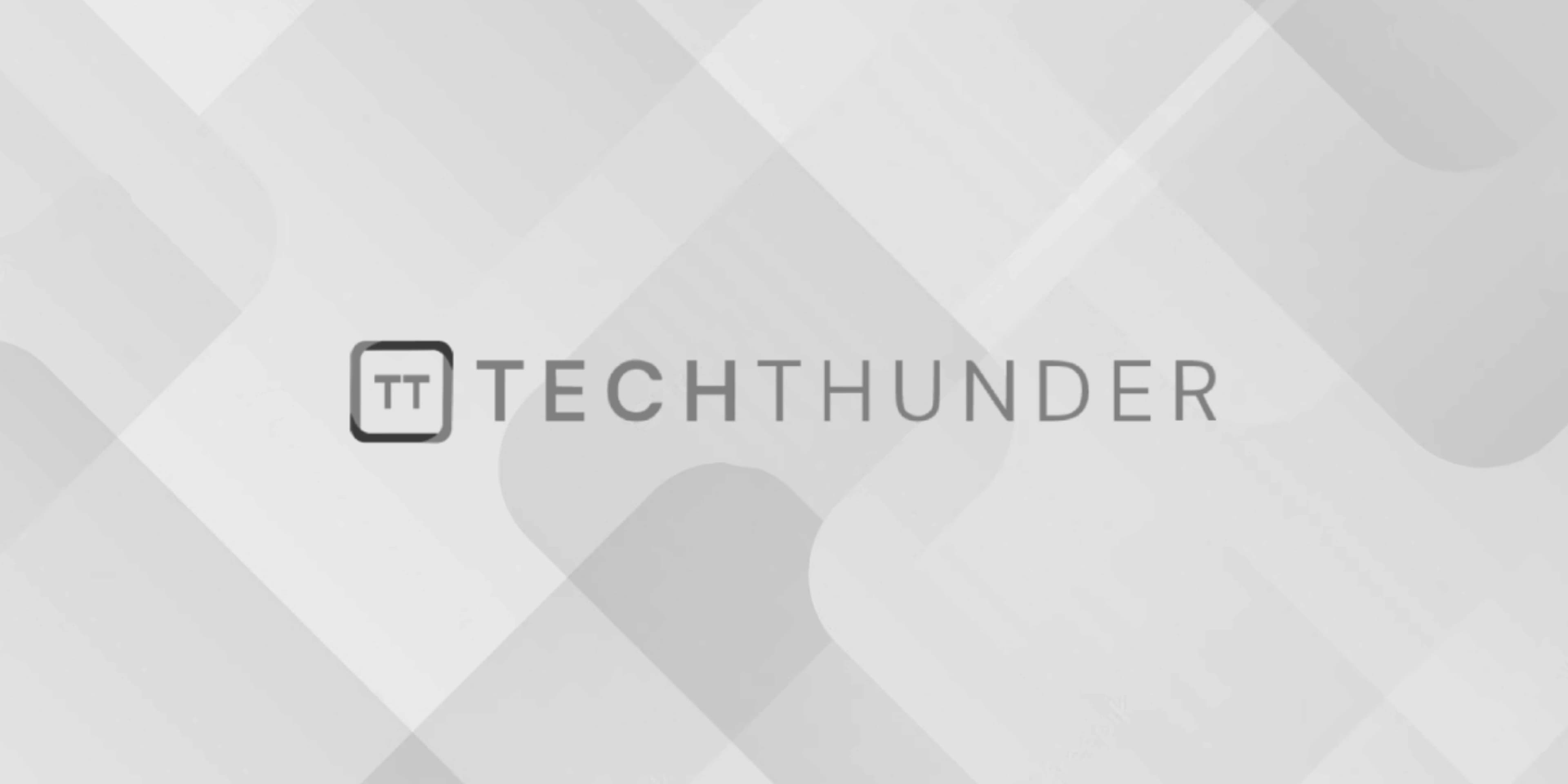
String concat()
The Java concat()
method is used to concatenate a specified string at the end of another string, creating a new string as a result. It returns a new string that is the combination of the original string (the one on which the concat()
method is called) and the specified string.
The basic syntax of the concat()
method is as follows:
String concatenatedString = originalString.concat(otherString);
originalString
: The original string to which you want to append the other string.otherString
: The string you want to concatenate with the original string.
Here’s a simple example:
public class ConcatExample {
public static void main(String[] args) {
String firstName = "John";
String lastName = "Doe";
String fullName = firstName.concat(" " + lastName);
System.out.println("Full Name: " + fullName);
}
}
In this example, the concat()
method is used to concatenate the first name and last name with a space in between, creating a full name. The fullName
string will contain the combined string, and the output will display “Full Name: John Doe.”
It’s important to note that the concat()
method creates a new string and does not modify the original string. Strings in Java are immutable, which means that once a string is created, its content cannot be changed. Therefore, any operation that appears to modify a string actually creates a new string with the desired changes.
In modern Java programming, using the +
operator or a StringBuilder
is often preferred for string concatenation because they provide more flexibility and better performance for complex string-building operations.