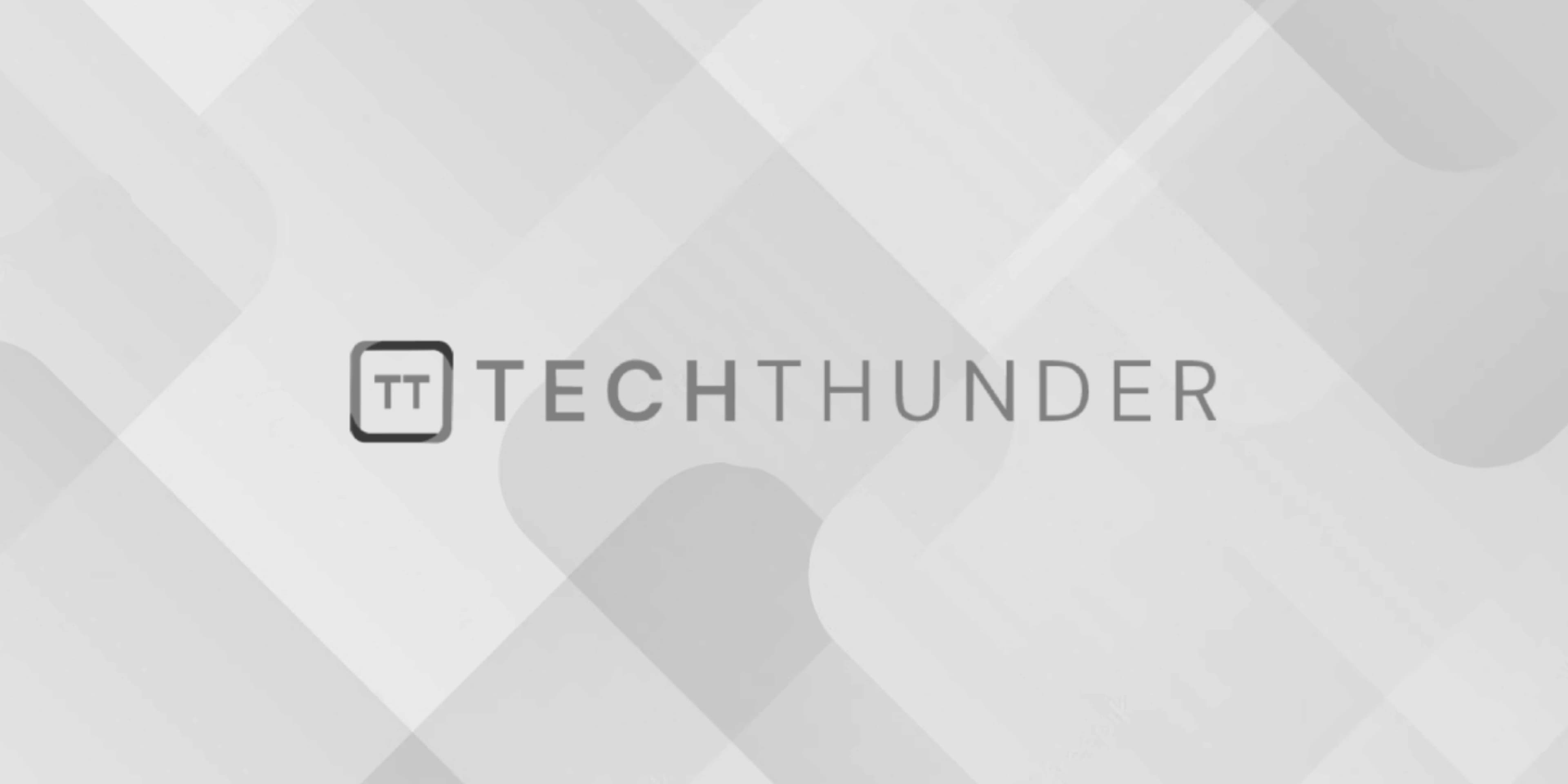
String toUpperCase()
The toUpperCase()
method in java is a part of the String
class and is used to convert all characters of a string to uppercase. This method returns a new string with all characters converted to uppercase while leaving any characters that are already uppercase unchanged.
The basic syntax of the toUpperCase()
method is as follows:
String toUpperCase()
Here’s how you can use it:
String original = "Hello World";
String uppercase = original.toUpperCase(); // "HELLO WORLD"
In this example, the toUpperCase()
method is called on the string "Hello World"
, and it returns a new string with all characters converted to uppercase.
Keep in mind the following:
- The
toUpperCase()
method doesn’t modify the original string. Instead, it creates a new string with the uppercase representation of the characters. - This method uses the default locale’s rules for converting characters to uppercase. If you need to perform case conversion according to a specific locale, you can use the overloaded version of this method that accepts a
Locale
argument:
String toUpperCase(Locale locale)
For example:
String original = "übermensch";
String uppercase = original.toUpperCase(Locale.GERMAN); // "ÜBERMENSCH"
- Some characters might have multiple uppercase equivalents in different locales. The conversion behavior might differ based on the rules of the locale.
Here are a few more examples:
String str1 = "Java";
String str2 = "UpPeRcAsE";
String str3 = "ThIs Is A MiXeD CaSe StRiNg";
String uppercase1 = str1.toUpperCase(); // "JAVA"
String uppercase2 = str2.toUpperCase(); // "UPPERCASE"
String uppercase3 = str3.toUpperCase(); // "THIS IS A MIXED CASE STRING"
The toUpperCase()
method is particularly useful when you need to perform case-insensitive string comparisons or when you want to ensure consistent case usage in your application, regardless of the input.