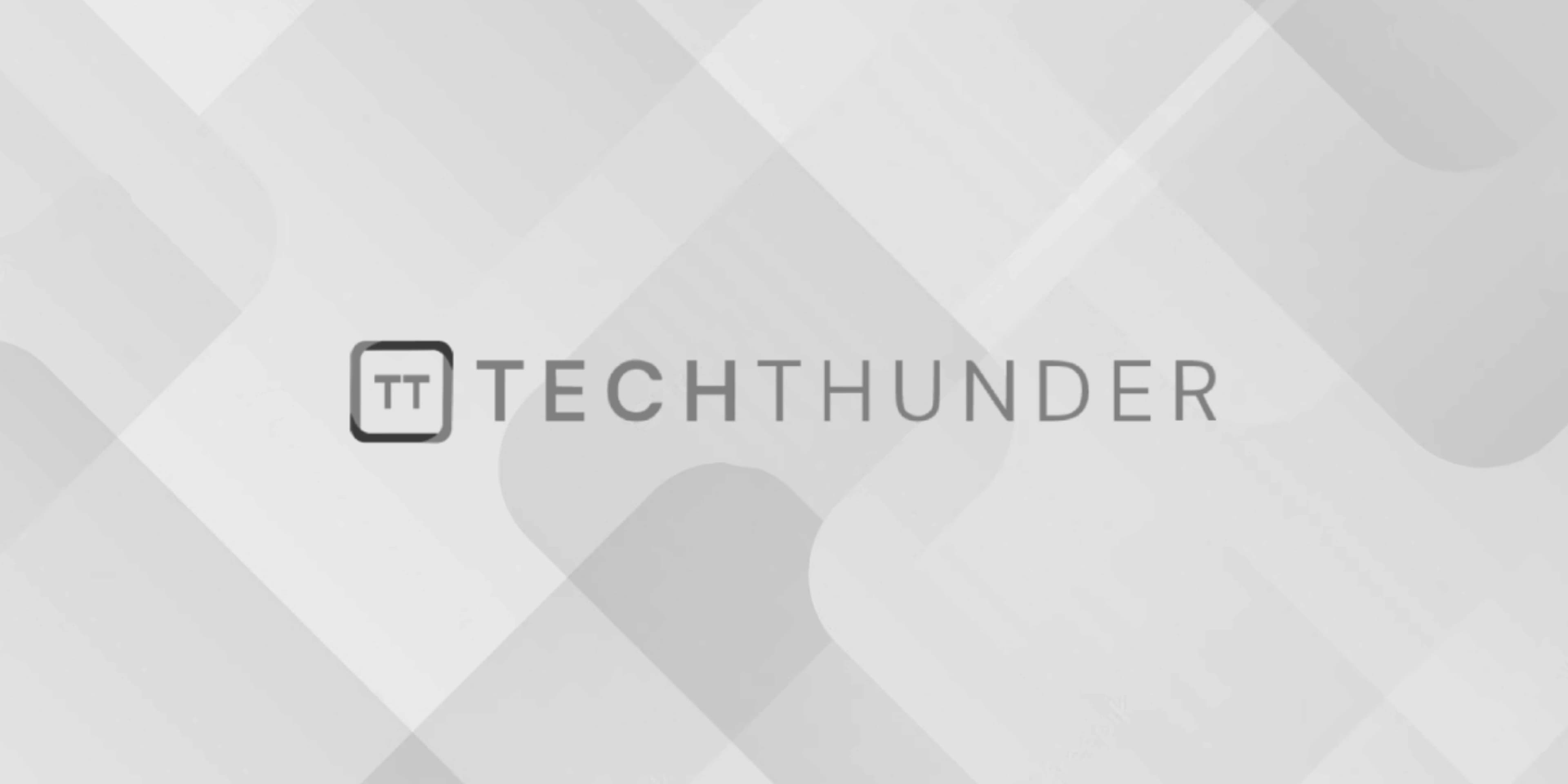
257 views
String Methods of String class
The String
class in Java provides a variety of methods for working with strings. Here’s an overview of some commonly used methods of the String
class:
- Length and Character Access:
int length()
: Returns the length (number of characters) of the string.char charAt(int index)
: Returns the character at the specified index.int codePointAt(int index)
: Returns the Unicode code point at the specified index.
- Substring:
String substring(int beginIndex)
: Returns a substring starting from thebeginIndex
to the end of the string.String substring(int beginIndex, int endIndex)
: Returns a substring frombeginIndex
(inclusive) toendIndex
(exclusive).
- Concatenation:
String concat(String str)
: Concatenates the specified string to the end of the invoking string.String + String
: You can also use the+
operator for string concatenation.
- Comparison:
boolean equals(Object obj)
: Compares the string with the specified object for equality.boolean equalsIgnoreCase(String anotherString)
: Compares the string to another string while ignoring case.int compareTo(String anotherString)
: Compares the string lexicographically to another string.boolean startsWith(String prefix)
: Checks if the string starts with a specified prefix.boolean endsWith(String suffix)
: Checks if the string ends with a specified suffix.int indexOf(String str)
: Returns the index of the first occurrence of a substring.int lastIndexOf(String str)
: Returns the index of the last occurrence of a substring.
- Search and Replace:
boolean contains(CharSequence sequence)
: Checks if a specified sequence of characters is present in the string.String replace(char oldChar, char newChar)
: Replaces all occurrences ofoldChar
withnewChar
.String replace(CharSequence target, CharSequence replacement)
: Replaces all occurrences oftarget
withreplacement
.String replaceAll(String regex, String replacement)
: Replaces all substrings that match the regular expression with the replacement string.
- Whitespace Trimming:
String trim()
: Removes leading and trailing whitespaces from the string.
- Case Conversion:
String toLowerCase()
: Converts the string to lowercase.String toUpperCase()
: Converts the string to uppercase.
- Checking for Empty or Null:
boolean isEmpty()
: Checks if the string is empty (has a length of 0).boolean isBlank()
: Checks if the string is empty or contains only white spaces.
- Splitting:
String[] split(String regex)
: Splits the string into an array of substrings using a regular expression as the delimiter.String[] split(String regex, int limit)
: Splits the string with a specified limit on the number of resulting substrings.
- Formatting:
String format(String format, Object... args)
: Returns a formatted string using the specified format string and arguments.
- String Representation:
String toString()
: Returns a string representation of the object.
- Encoding and Decoding:
byte[] getBytes()
: Converts the string to a byte array using the platform’s default character encoding.byte[] getBytes(String charsetName)
: Converts the string to a byte array using a specified character encoding.String(byte[] bytes)
: Constructs a new string by decoding a byte array using the platform’s default character encoding.String(byte[] bytes, String charsetName)
: Constructs a new string by decoding a byte array using a specified character encoding.
These are just some of the commonly used methods provided by the String
class in Java. Depending on your specific requirements, you may choose the appropriate methods to manipulate and work with strings in your applications.