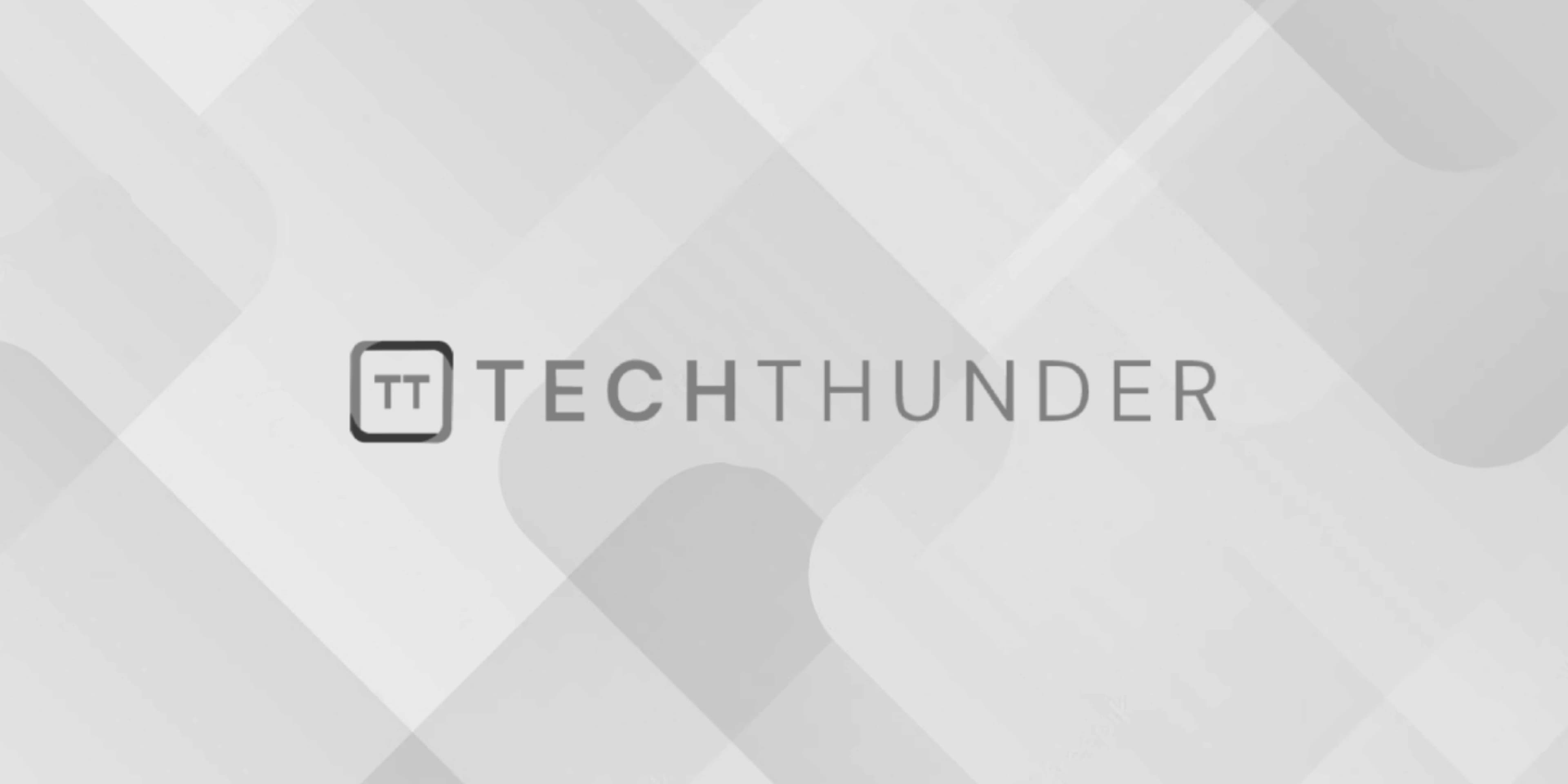
151 views
String length()
The length()
method in java is a part of the String
class and is used to retrieve the number of characters in a string. It returns an integer value representing the length of the string.
The basic syntax of the length()
method is as follows:
int length()
Here’s how you can use it:
String str = "Hello, world!";
int len = str.length(); // 13
In this example, the length()
method is called on the string "Hello, world!"
, and it returns the value 13, which is the number of characters in the string.
Key points to note:
- The
length()
method returns the number of characters in the string, including letters, digits, spaces, punctuation, and any other Unicode characters. - The method doesn’t require parentheses because it’s a method, not a field or a property.
- The length of the string is 0 for an empty string (
""
).
Here are a few more examples:
String empty = "";
int emptyLength = empty.length(); // 0
String digits = "12345";
int digitsLength = digits.length(); // 5
String stringWithSpaces = " Spaces ";
int stringWithSpacesLength = stringWithSpaces.length(); // 15
In the above examples, emptyLength
is 0 because the empty string has no characters. digitsLength
is 5 because the string “12345” contains five digits. stringWithSpacesLength
is 15 because the string ” Spaces ” has 15 characters, including the spaces.