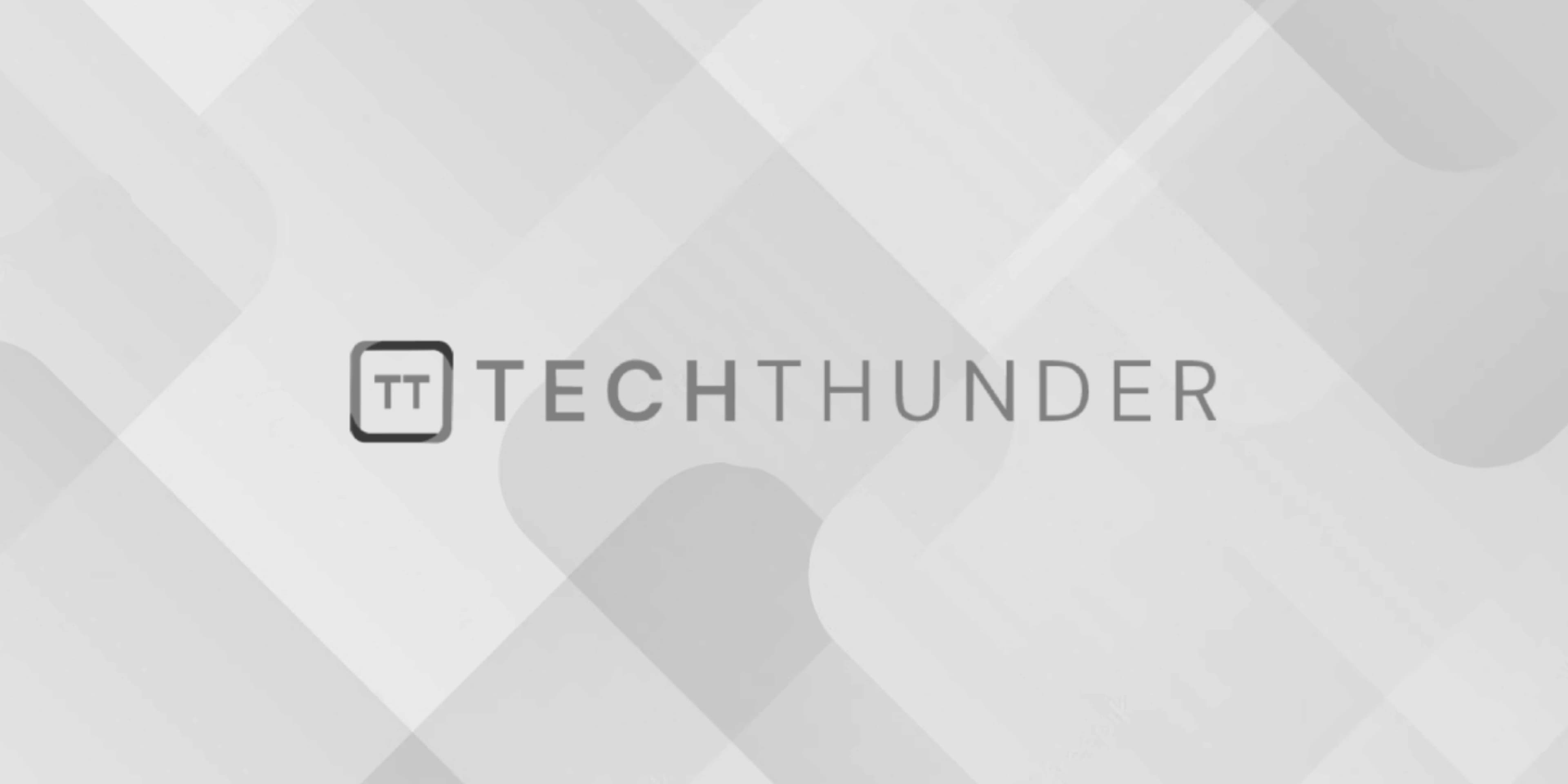
233 views
String trim()
The trim()
method in java is a part of the String
class and is used to remove leading and trailing whitespace characters from a string. It returns a new string with all whitespace characters removed from the beginning and end of the original string.
The basic syntax of the trim()
method is as follows:
String trim()
Here’s how you can use it:
String original = " Hello, World! ";
String trimmed = original.trim(); // "Hello, World!"
In this example, the trim()
method is called on the string " Hello, World! "
, and it returns a new string with the leading and trailing whitespace characters removed.
Keep in mind the following:
- The
trim()
method doesn’t modify the original string. Instead, it creates a new string with the trimmed content. - “Whitespace characters” in this context include space characters, tabs (
\t
), newline characters (\n
), carriage return characters (\r
), and other Unicode whitespace characters. - Only leading and trailing whitespace characters are removed. Any whitespace characters within the string are left unchanged.
- If the string consists only of whitespace characters, the result of
trim()
will be an empty string.
Here are a few more examples:
String str1 = " Leading and trailing spaces ";
String str2 = "\tTab characters\t";
String str3 = "\nNewline characters\n";
String str4 = "\rCarriage return characters\r";
String str5 = " \t \n Mixed whitespace \t\n ";
String trimmed1 = str1.trim(); // "Leading and trailing spaces"
String trimmed2 = str2.trim(); // "Tab characters"
String trimmed3 = str3.trim(); // "Newline characters"
String trimmed4 = str4.trim(); // "Carriage return characters"
String trimmed5 = str5.trim(); // "Mixed whitespace"
The trim()
method is often used when processing user input, such as removing extra spaces or ensuring that leading/trailing spaces do not affect the logic of your program.