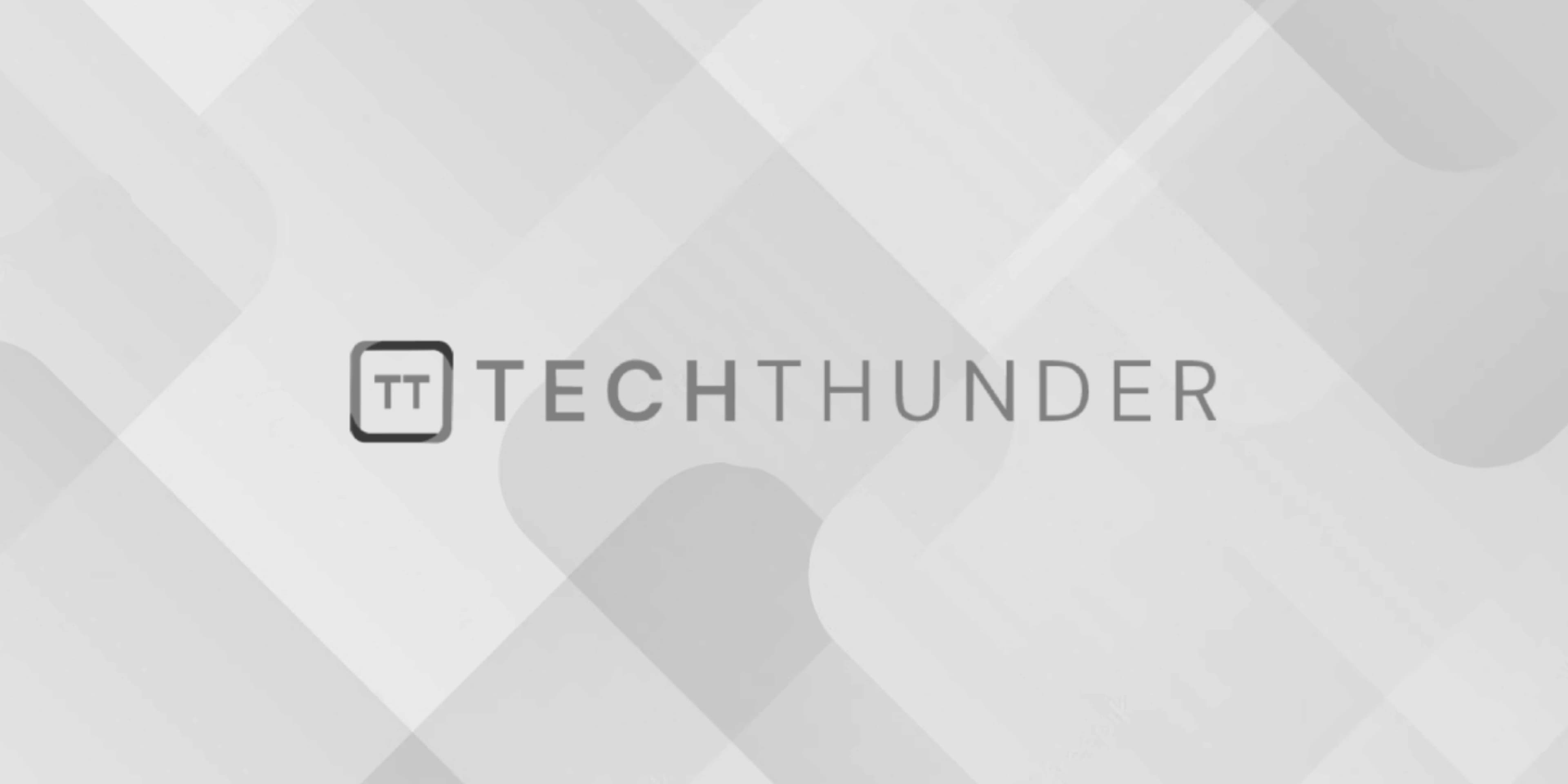
118 views
String vs StringBuffer
The Java has both String
and StringBuffer
are used to work with strings, but they have different characteristics and are suitable for different use cases. The primary distinction between them is their mutability and thread-safety:
- String:
- Immutable:
String
objects are immutable, which means that their content cannot be changed after they are created. Any operation that appears to modify aString
actually creates a newString
object. - Thread-Safe: Since
String
objects are immutable, they are inherently thread-safe. Multiple threads can read and shareString
objects without synchronization concerns. - Use Cases:
String
is suitable for situations where the string’s content remains constant and you don’t need to modify it. This is the preferred choice for representing constants and ensuring data integrity. - Performance: Due to their immutability, creating new
String
objects when modifying a string can be less efficient for large-scale string manipulation.
- StringBuffer:
- Mutable:
StringBuffer
objects are mutable, which means you can modify the characters in the sequence after creating the object. This allows for efficient string manipulation, such as appending, inserting, and deleting characters. - Not Thread-Safe:
StringBuffer
is not inherently thread-safe. If multiple threads access aStringBuffer
concurrently, external synchronization is required to avoid data corruption. - Use Cases:
StringBuffer
is a good choice when you need to perform dynamic and thread-safe string manipulations, especially in multi-threaded applications where synchronization is managed. - Performance:
StringBuffer
can be more efficient thanString
for string manipulations because it avoids creating new objects every time you modify the content.
- StringBuilder:
- Mutable and Not Thread-Safe:
StringBuilder
is similar toStringBuffer
in terms of mutability but is not thread-safe. It’s the preferred choice for single-threaded applications where you need efficient string manipulations. - Use Cases: If you don’t require thread safety,
StringBuilder
is a better choice thanStringBuffer
due to its better performance in single-threaded scenarios.
Here’s a summary of when to use each class:
- Use
String
when you need an immutable string or when thread-safety is not a concern. - Use
StringBuffer
when you need a thread-safe, mutable string, and you are working in a multi-threaded environment. - Use
StringBuilder
when you need a mutable string and you are working in a single-threaded environment.StringBuilder
is more efficient thanStringBuffer
in single-threaded scenarios due to its lack of synchronization.
To choosing the appropriate class depends on the specific requirements of your application. For most single-threaded scenarios, StringBuilder
is often the preferred choice for efficient string manipulations, while StringBuffer
is used in multi-threaded scenarios. String
is primarily used for immutable string representations.