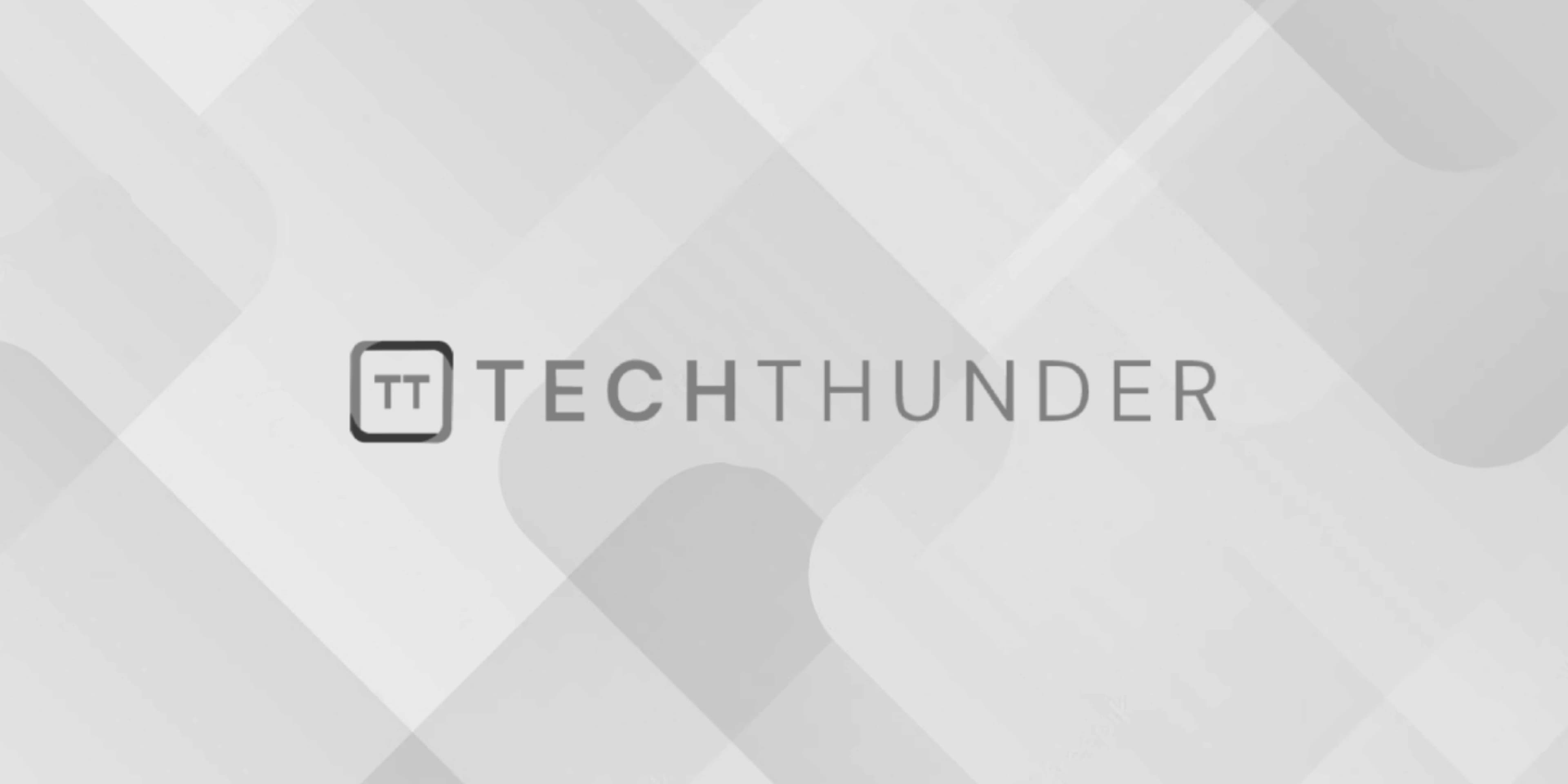
String getBytes()
The Java getBytes()
method of the String
class is used to encode a string into a sequence of bytes using the platform’s default character encoding. It returns a byte array that represents the characters in the string based on the default character encoding.
The basic syntax of the getBytes()
method is as follows:
byte[] byteArray = str.getBytes();
str
: The string that you want to convert into a byte array.
Here’s a simple example:
public class GetBytesExample {
public static void main(String[] args) {
String text = "Hello, World!";
byte[] byteArray = text.getBytes();
for (byte b : byteArray) {
System.out.print(b + " ");
}
}
}
In this example, the getBytes()
method is used to convert the string text
into a byte array. The resulting byteArray
contains the byte values of the characters in the string, based on the default character encoding (which is typically UTF-8 in modern Java implementations). The for
loop is used to print the byte values.
It’s important to note that the actual byte representation of a string can vary depending on the character encoding being used. If you need to specify a specific character encoding, you can provide it as an argument to the getBytes()
method:
byte[] byteArray = str.getBytes("UTF-16");
In this example, the string is converted to a byte array using the UTF-16 character encoding. You can replace “UTF-16” with the appropriate character encoding according to your requirements.
When working with character encodings, it’s crucial to ensure that you handle character encoding and decoding consistently to avoid unexpected issues with data integrity, especially when dealing with text data from different sources or systems.