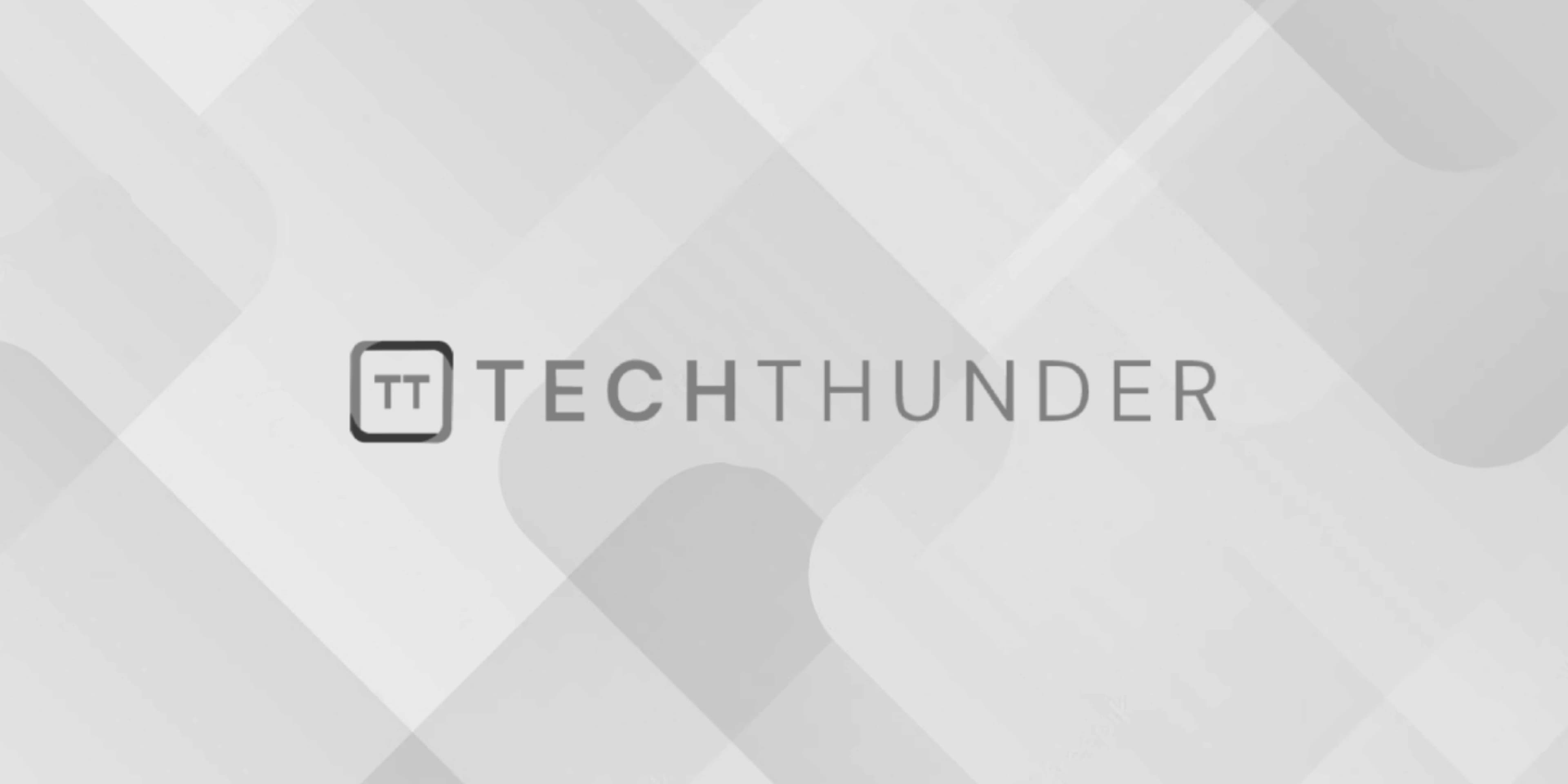
98 views
MySQL Signal Resignal
The MySQL SIGNAL
and RESIGNAL
statements are used within stored procedures, triggers, and functions to raise and re-raise custom error conditions. These statements are helpful for handling exceptions and providing more informative error messages to users or developers.
Here’s a brief explanation of each:
- SIGNAL Statement: The
SIGNAL
statement allows you to raise a custom error condition within a MySQL stored procedure, trigger, or function. You can specify an SQL state, a message text, and a specific error code. The basic syntax is as follows:
SIGNAL SQLSTATE 'sql_state_value' SET MESSAGE_TEXT = 'message_text', MYSQL_ERRNO = error_code;
sql_state_value
: A five-character SQL state code. This code is used to identify the error condition.message_text
: A custom error message that provides information about the error.error_code
: A specific error code to identify the error condition. Here’s an example of using theSIGNAL
statement within a stored procedure:
DELIMITER //
CREATE PROCEDURE DivideNumbers(a INT, b INT)
BEGIN
IF b = 0 THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Division by zero is not allowed', MYSQL_ERRNO = 1001;
ELSE
-- Perform the division
END IF;
END //
DELIMITER ;
- RESIGNAL Statement: The
RESIGNAL
statement allows you to re-raise an error condition that was previously caught or handled. This is useful if you catch an error and want to provide more specific information about the error or re-raise the error for higher-level handling. The basic syntax is as follows:
RESIGNAL SQLSTATE 'sql_state_value' SET MESSAGE_TEXT = 'new_message_text', MYSQL_ERRNO = new_error_code;
sql_state_value
: A five-character SQL state code.new_message_text
: A new error message to replace the original one.new_error_code
: A new error code to replace the original one. Here’s an example of using theRESIGNAL
statement within a stored procedure:
DELIMITER //
CREATE PROCEDURE HandleDivisionErrors(a INT, b INT)
BEGIN
DECLARE division_error CONDITION FOR SQLSTATE '45000';
-- Attempt the division
-- If an error occurs, catch it and provide more specific information
DECLARE CONTINUE HANDLER FOR division_error
BEGIN
RESIGNAL SQLSTATE '45001'
SET MESSAGE_TEXT = 'Division error: ' || MYSQL_ERRNO, MYSQL_ERRNO = 1002;
END;
-- Perform the division
-- If an error occurs, it will be caught and re-raised with new details
END //
DELIMITER ;
These statements are valuable for handling and communicating errors in your MySQL stored procedures, triggers, and functions, allowing you to provide more meaningful and informative error messages to users or developers.