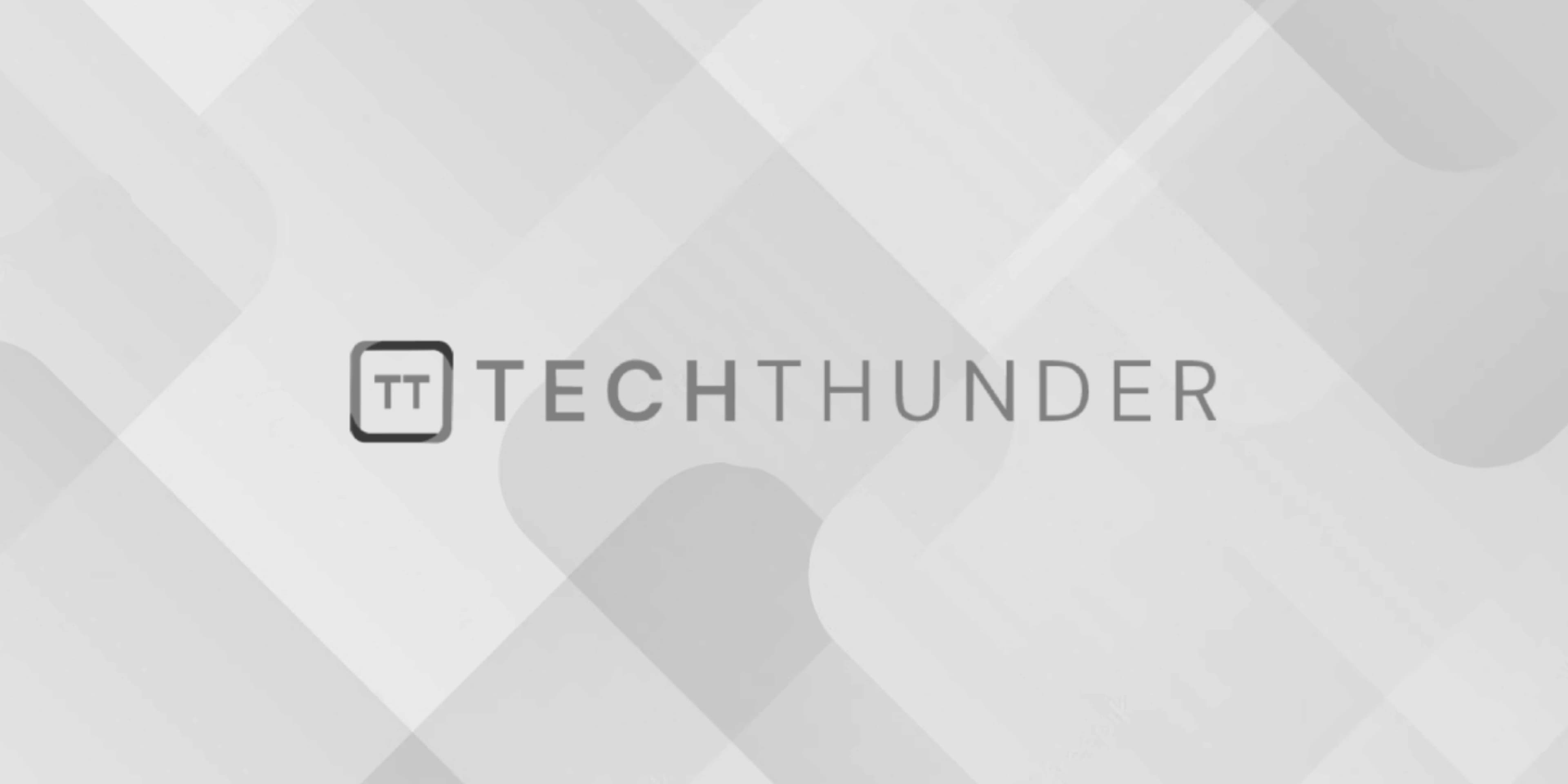
MySQL INSERT Record
To insert a record (a row of data) into a MySQL table, you can use the INSERT INTO
statement. Here’s the basic syntax for inserting a record into a MySQL table:
INSERT INTO table_name (column1, column2, column3, ...)
VALUES (value1, value2, value3, ...);
table_name
: The name of the table where you want to insert the record.column1, column2, column3, ...
: The names of the columns in the table where you want to insert data.value1, value2, value3, ...
: The corresponding values for the columns you specified. The values should match the data types and order of the columns.
Here’s an example of how to insert a record into a hypothetical “students” table:
INSERT INTO students (student_id, first_name, last_name, age)
VALUES (1, 'John', 'Doe', 20);
In this example:
- The
students
table has columns namedstudent_id
,first_name
,last_name
, andage
. - We’re inserting a new record with a student ID of 1, a first name of ‘John’, a last name of ‘Doe’, and an age of 20.
Make sure to provide values for all the columns that are not nullable (i.e., columns that do not have a NULL
constraint) and ensure that the data types of the values match the data types of the corresponding columns.
If you want to insert multiple records in a single INSERT
statement, you can use a syntax like this:
INSERT INTO students (student_id, first_name, last_name, age)
VALUES
(1, 'John', 'Doe', 20),
(2, 'Jane', 'Smith', 22),
(3, 'Alice', 'Johnson', 21);
This inserts three records into the “students” table in one statement.
After executing the INSERT
statement, the new records will be added to the specified table. You can use a similar approach to insert records into other tables in your database.