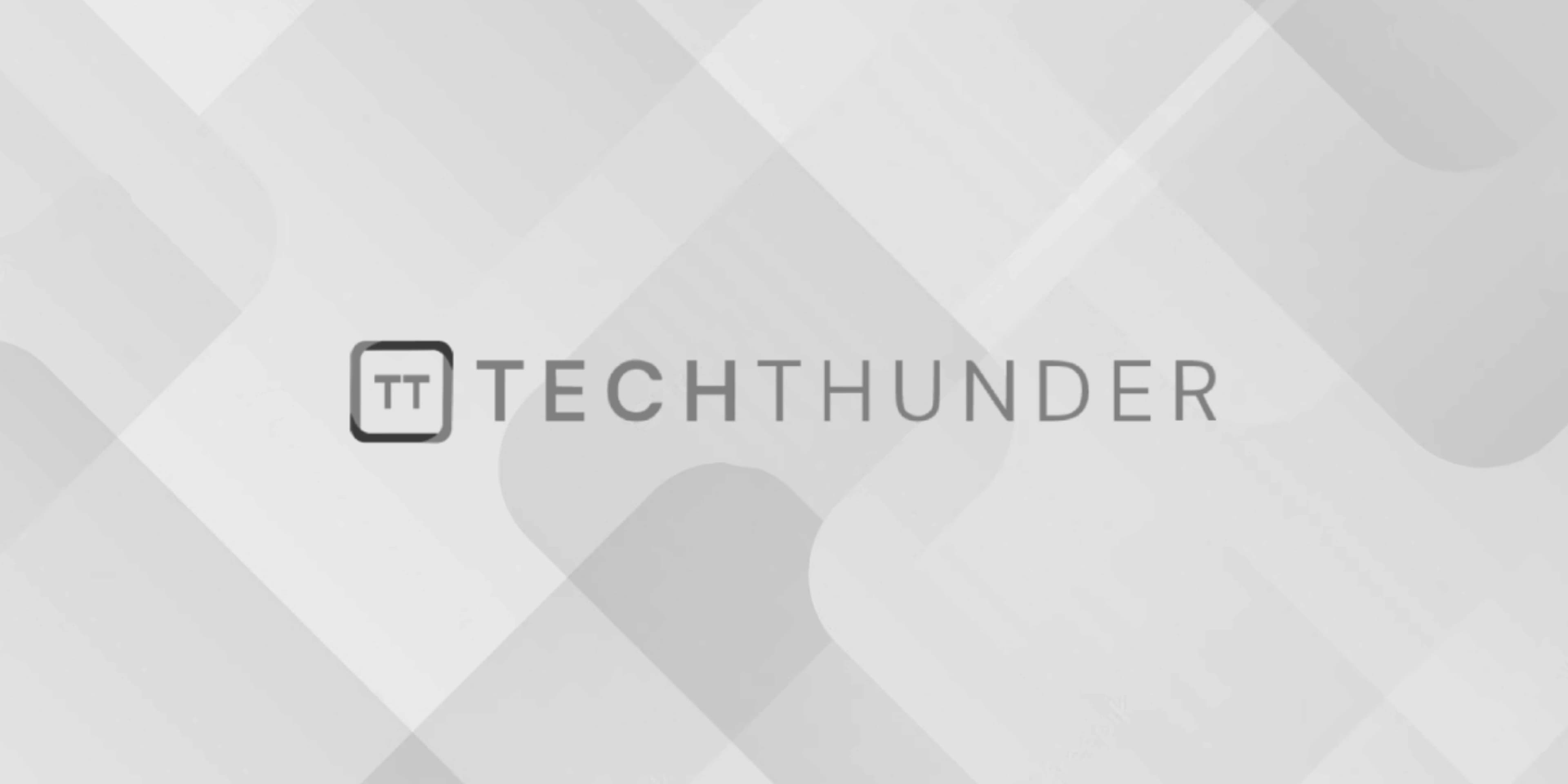
MySQL AFTER UPDATE Trigger
The AFTER UPDATE trigger in MySQL is a type of database trigger that automatically executes after an update operation is performed on a table. This type of trigger allows you to perform actions or log information after data has been updated in the table. Here’s how you can create an “AFTER UPDATE” trigger:
DELIMITER //
CREATE TRIGGER after_update_trigger
AFTER UPDATE ON your_table
FOR EACH ROW
BEGIN
-- Trigger logic here
-- You can reference OLD.column_name and NEW.column_name to access the previous and new values.
-- You can perform actions like logging, updating other tables, or sending notifications.
END;
//
DELIMITER ;
Let’s break down the components of the “AFTER UPDATE” trigger:
after_update_trigger
: This is the name of your trigger. You should choose a unique name for the trigger.AFTER UPDATE
: Specifies that the trigger should execute after an update operation on the specified table.your_table
: Replace this with the name of the table on which you want to create the trigger.FOR EACH ROW
: Indicates that the trigger will execute once for each row affected by the update.BEGIN
andEND
: These enclose the trigger logic, where you can include SQL statements to perform actions or logging after the update operation.
Inside the trigger logic, you can reference both OLD.column_name
and NEW.column_name
. The OLD.column_name
represents the previous value in the column before the update, and NEW.column_name
represents the new value after the update. You can use these values to perform various actions, such as logging the changes, updating other tables, or sending notifications.
Here’s an example of an “AFTER UPDATE” trigger that logs information about updated records into an audit table:
DELIMITER //
CREATE TRIGGER log_after_update
AFTER UPDATE ON orders
FOR EACH ROW
BEGIN
INSERT INTO order_log (order_id, action, action_time)
VALUES (NEW.order_id, 'UPDATE', NOW());
END;
//
DELIMITER ;
In this example, the trigger logs the order_id
and the action as ‘UPDATE’ along with the current timestamp into an order_log
table each time an order record is updated.
AFTER UPDATE triggers are useful for post-update actions, such as data auditing, history tracking, or triggering further events based on the updated data. Make sure that your trigger logic is efficient to avoid performance issues when using such triggers.