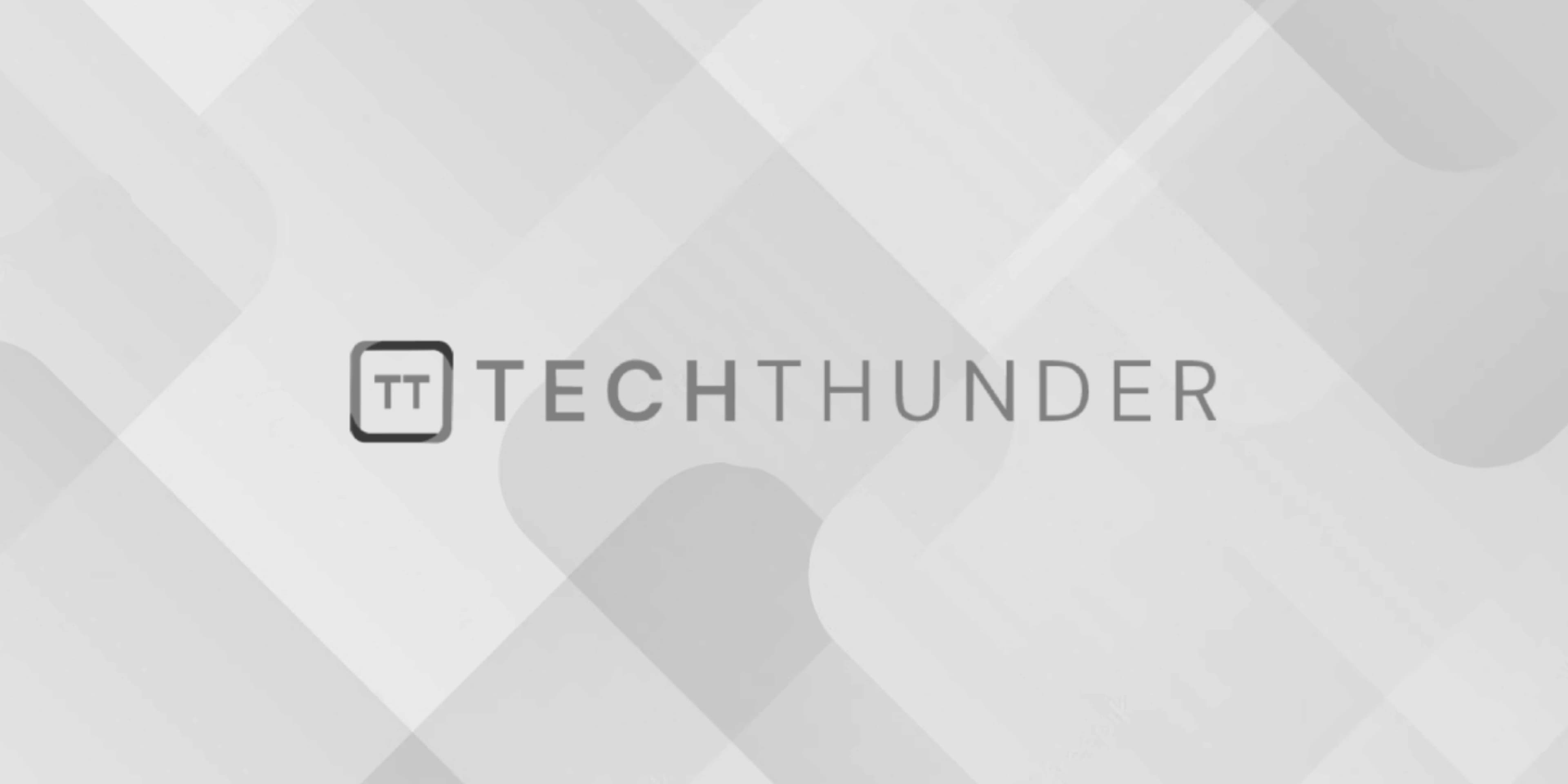
MySQL BEFORE UPDATE Trigger
The BEFORE UPDATE trigger in MySQL is a type of database trigger that is automatically executed before an update operation is performed on a table. This type of trigger allows you to validate or modify data before it is updated in the table. Here’s how you can create a “BEFORE UPDATE” trigger:
DELIMITER //
CREATE TRIGGER before_update_trigger
BEFORE UPDATE ON your_table
FOR EACH ROW
BEGIN
-- Trigger logic here
-- You can reference OLD.column_name and NEW.column_name to access the existing and new values.
-- You can perform validations or data modifications.
END;
//
DELIMITER ;
Let’s break down the components of the “BEFORE UPDATE” trigger:
before_update_trigger
: This is the name of your trigger. You should choose a unique name for the trigger.BEFORE UPDATE
: Specifies that the trigger should execute before an update operation on the specified table.your_table
: Replace this with the name of the table on which you want to create the trigger.FOR EACH ROW
: Indicates that the trigger will execute once for each row affected by the update.BEGIN
andEND
: These enclose the trigger logic, where you can include SQL statements to perform validations or modifications before the update operation.
Inside the trigger logic, you can reference both OLD.column_name
and NEW.column_name
. The OLD.column_name
represents the existing value in the column before the update, and NEW.column_name
represents the new value being inserted. You can use these values to perform validations or modify data as needed.
Here’s an example of a “BEFORE UPDATE” trigger that prevents the update of a product’s price to a negative value:
DELIMITER //
CREATE TRIGGER prevent_negative_price_update
BEFORE UPDATE ON products
FOR EACH ROW
BEGIN
IF NEW.price < 0 THEN
SIGNAL SQLSTATE '45000'
SET MESSAGE_TEXT = 'Price cannot be updated to a negative value';
END IF;
END;
//
DELIMITER ;
In this example, the trigger checks whether the new price being updated is negative and, if so, raises an error using the SIGNAL
statement to prevent the update.
The BEFORE UPDATE triggers are useful for enforcing data validation rules or performing data transformations before the data is updated in the table. Be cautious when using triggers and ensure that your trigger logic is efficient to avoid potential performance issues.