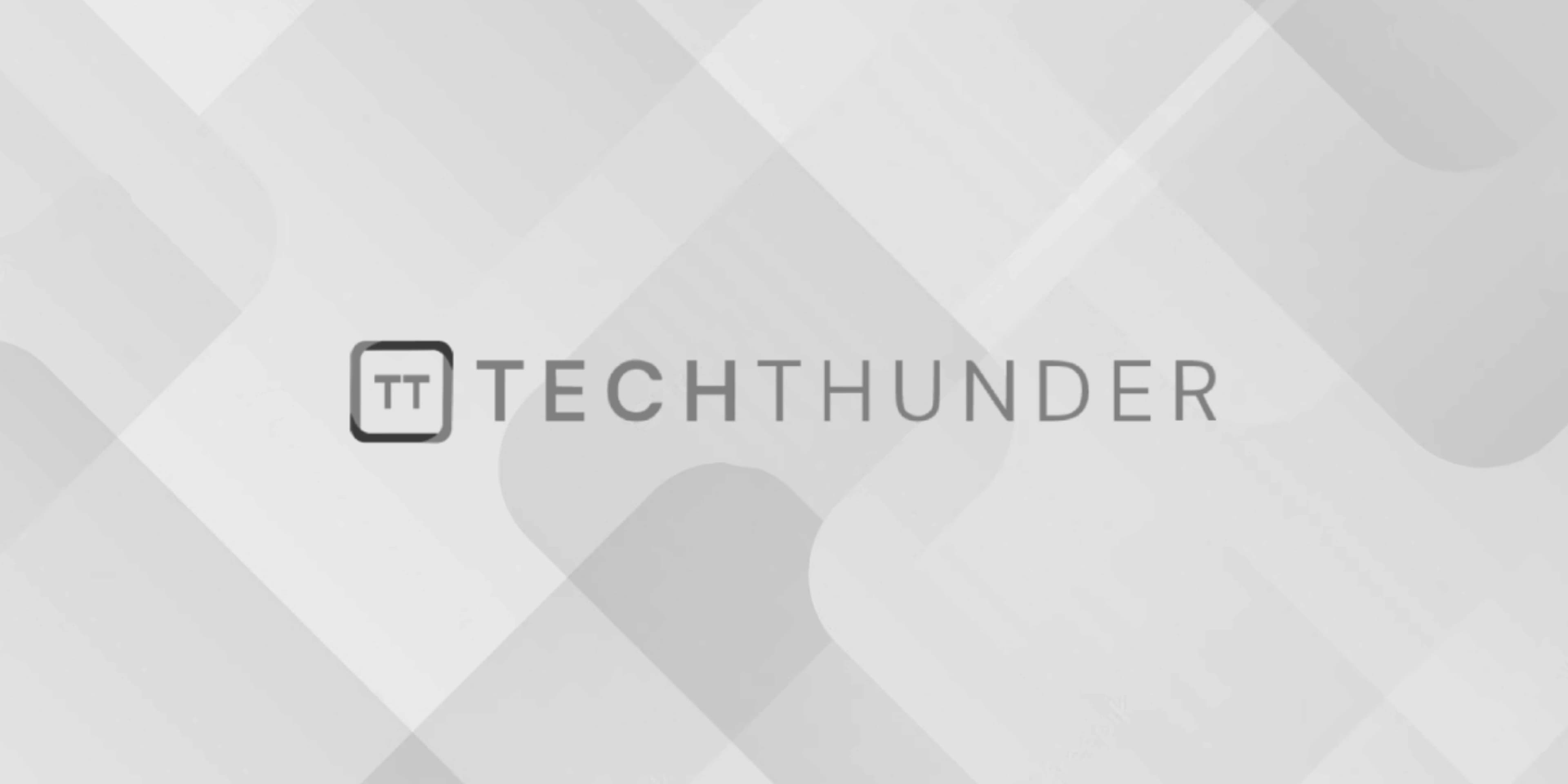
Regular Expressions
Regular expressions, often referred to as regex or regexp, are a powerful tool for pattern matching and text manipulation. They provide a concise and flexible way to search, match, and manipulate text based on specific patterns. Regular expressions are widely used in programming, text processing, and data validation tasks.
Here are some key concepts and components of regular expressions:
- Character Matching:
- Literal Characters: Regular expressions can match literal characters. For example, the regular expression
apple
matches the word “apple” in text.
- Metacharacters:
- Metacharacters have special meanings in regular expressions. Some common metacharacters include:
.
(dot): Matches any single character except a newline.*
(asterisk): Matches zero or more occurrences of the preceding character or group.+
(plus): Matches one or more occurrences of the preceding character or group.?
(question mark): Matches zero or one occurrence of the preceding character or group.[]
(square brackets): Defines a character class, matching any character within the brackets.()
(parentheses): Groups characters or expressions together.
- Character Classes:
- Character classes allow you to match any character from a specific set. For example,
[aeiou]
matches any vowel. - Ranges can be defined, such as
[0-9]
to match any digit.
- Anchors:
- Anchors define the position in the text where a match should occur.
^
(caret): Matches the start of a line.$
(dollar sign): Matches the end of a line.
- Quantifiers:
- Quantifiers specify the number of times a character or group should be matched.
*
(asterisk): Matches zero or more occurrences.+
(plus): Matches one or more occurrences.{n}
: Matches exactlyn
occurrences.{n,}
: Matches at leastn
occurrences.{n,m}
: Matches betweenn
andm
occurrences.
- Modifiers:
- Modifiers change the behavior of a regular expression.
i
: Case-insensitive matching.g
: Global matching (matches all occurrences in the text).
- Escape Character:
\
(backslash): Escapes a metacharacter to match it as a literal character. For example,\.
matches a period.
Regular expressions are used in various programming languages, text editors, and tools. Here’s an example of using regular expressions in Python:
import re
text = "The quick brown fox jumps over the lazy dog."
pattern = r"\b\w{3}\b" # Matches three-letter words.
matches = re.findall(pattern, text, re.IGNORECASE)
print(matches)
In this Python code, the re
module is used to find and print three-letter words in the text.
Regular expressions are a powerful tool for text processing, but they can also be complex and challenging to work with. Learning and mastering regular expressions can greatly enhance your ability to manipulate and process text efficiently. Many online resources and tools are available for learning and testing regular expressions.