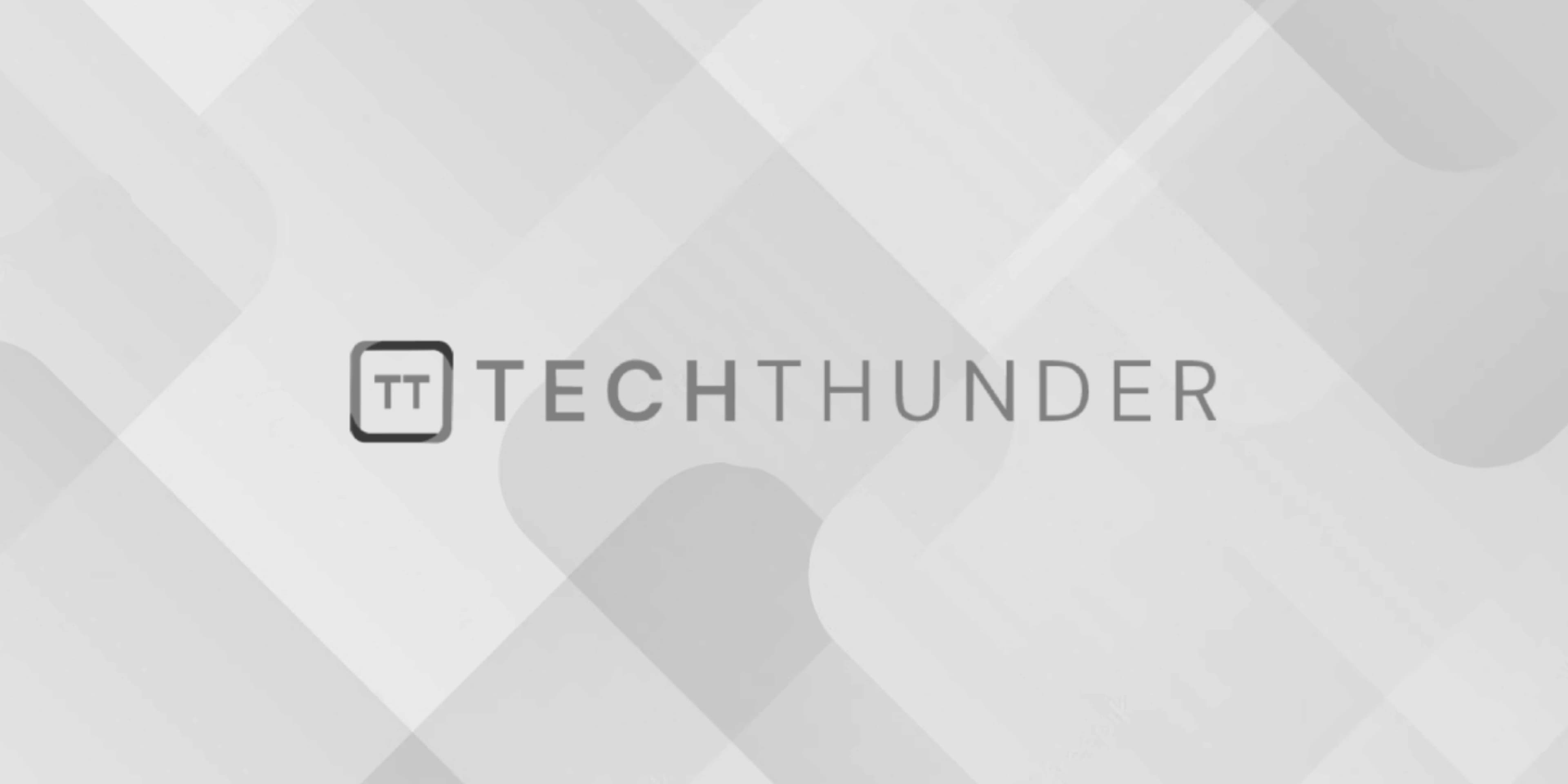
MySQL Sequence
The MySQL doesn’t have a built-in SEQUENCE object like some other database management systems (e.g., Oracle, PostgreSQL). However, you can create your own sequence-like functionality using MySQL tables and queries. Here’s how you can do it:
- Create a Sequence Table:
You can create a table to store the sequence’s current value. For example:
CREATE TABLE my_sequence (
name VARCHAR(50) PRIMARY KEY,
value INT
);
INSERT INTO my_sequence (name, value) VALUES ('my_sequence_name', 1);
In this example, we create a table my_sequence
with a name (for identification) and a value column.
- Increment the Sequence:
To increment the sequence, you can use anUPDATE
statement to increment the value in the table:
UPDATE my_sequence
SET value = value + 1
WHERE name = 'my_sequence_name';
You can execute this query to increment the sequence by one each time you need a new value.
- Retrieve the Current Sequence Value:
When you need to get the current value of the sequence, simply query the table:
SELECT value
FROM my_sequence
WHERE name = 'my_sequence_name';
This query will return the current value of the sequence without incrementing it.
- Reset or Initialize the Sequence:
If you want to reset or initialize the sequence to a specific value, you can use anUPDATE
statement:
UPDATE my_sequence
SET value = new_value
WHERE name = 'my_sequence_name';
Replace new_value
with the desired starting value.
By using a dedicated sequence table, you can simulate a sequence in MySQL. However, it’s worth noting that MySQL 8.0 introduced the AUTO_INCREMENT
column property, which simplifies sequence generation for primary key columns in tables. If your goal is to generate unique values for primary keys, you can utilize AUTO_INCREMENT
.