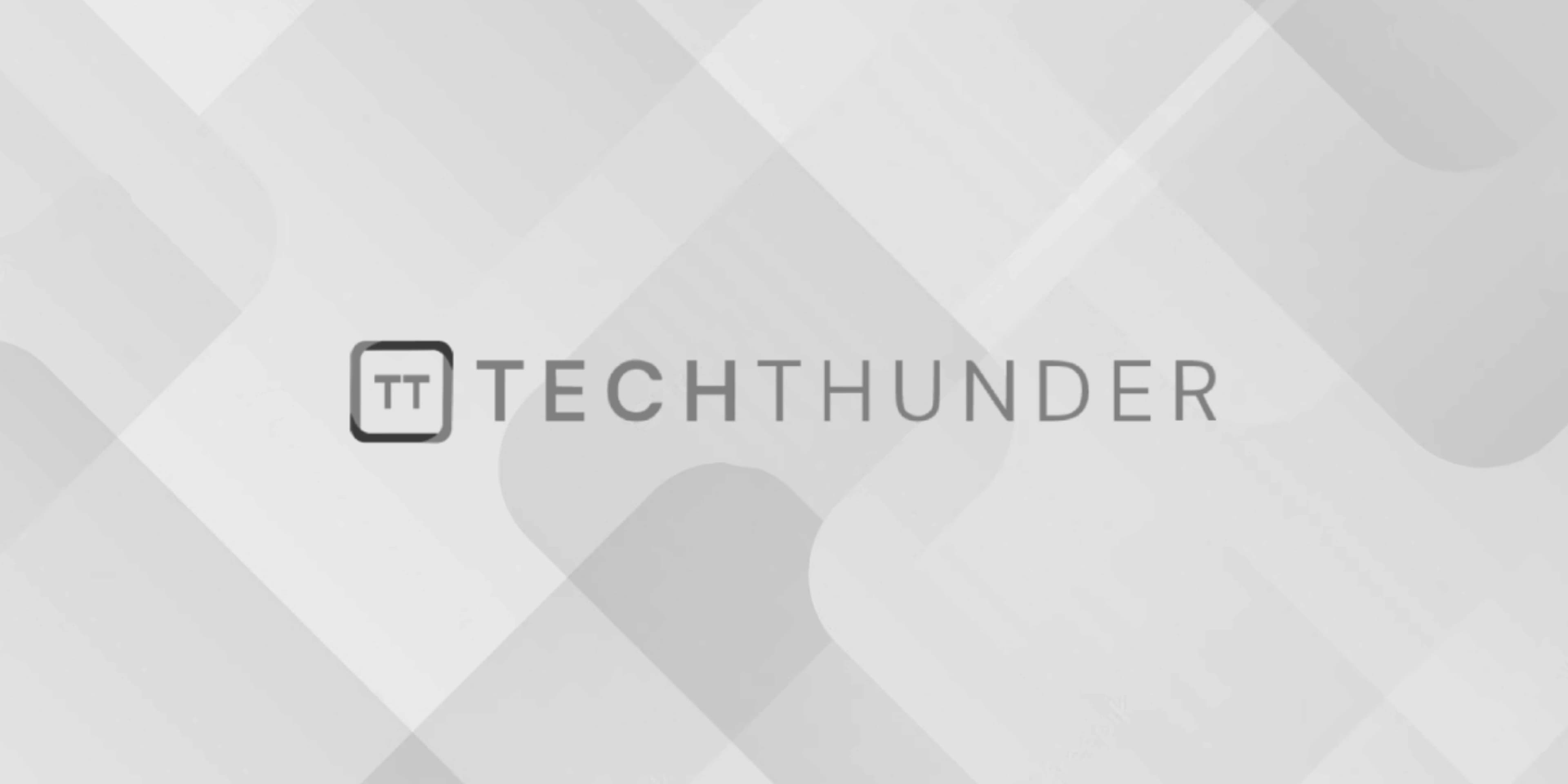
MySQL Inner Join
The INNER JOIN
in MySQL is a type of join operation that returns only the rows for which there is a match between the columns in the tables being joined. It combines rows from two or more tables based on a specified condition, and it excludes rows from both tables that do not have corresponding values in the other table.
Here’s the basic syntax for an INNER JOIN
:
SELECT columns
FROM table1
INNER JOIN table2 ON table1.column_name = table2.column_name;
Let’s break down the components of this syntax:
SELECT columns
: Specifies the columns you want to retrieve from the joined tables. You can select specific columns from both tables.FROM table1
: Indicates the first table you want to include in the join.INNER JOIN table2
: Specifies the second table you want to join with the first table.ON table1.column_name = table2.column_name
: This is the join condition. It defines which columns in each table are used to match rows from the two tables.
Here’s a practical example of using an INNER JOIN
. Suppose you have two tables, employees
and departments
, and you want to retrieve the names of employees and their corresponding department names based on a shared department_id
:
SELECT employees.employee_name, departments.department_name
FROM employees
INNER JOIN departments ON employees.department_id = departments.department_id;
In this example, the INNER JOIN
combines rows where the department_id
values match in both tables. This produces a result set that includes the names of employees and their corresponding department names, but only for employees who belong to a department.
An INNER JOIN
is useful when you want to retrieve data only when there is a match in both tables, ensuring that you get related information without including unrelated rows. It is one of the most common types of joins used in SQL queries.