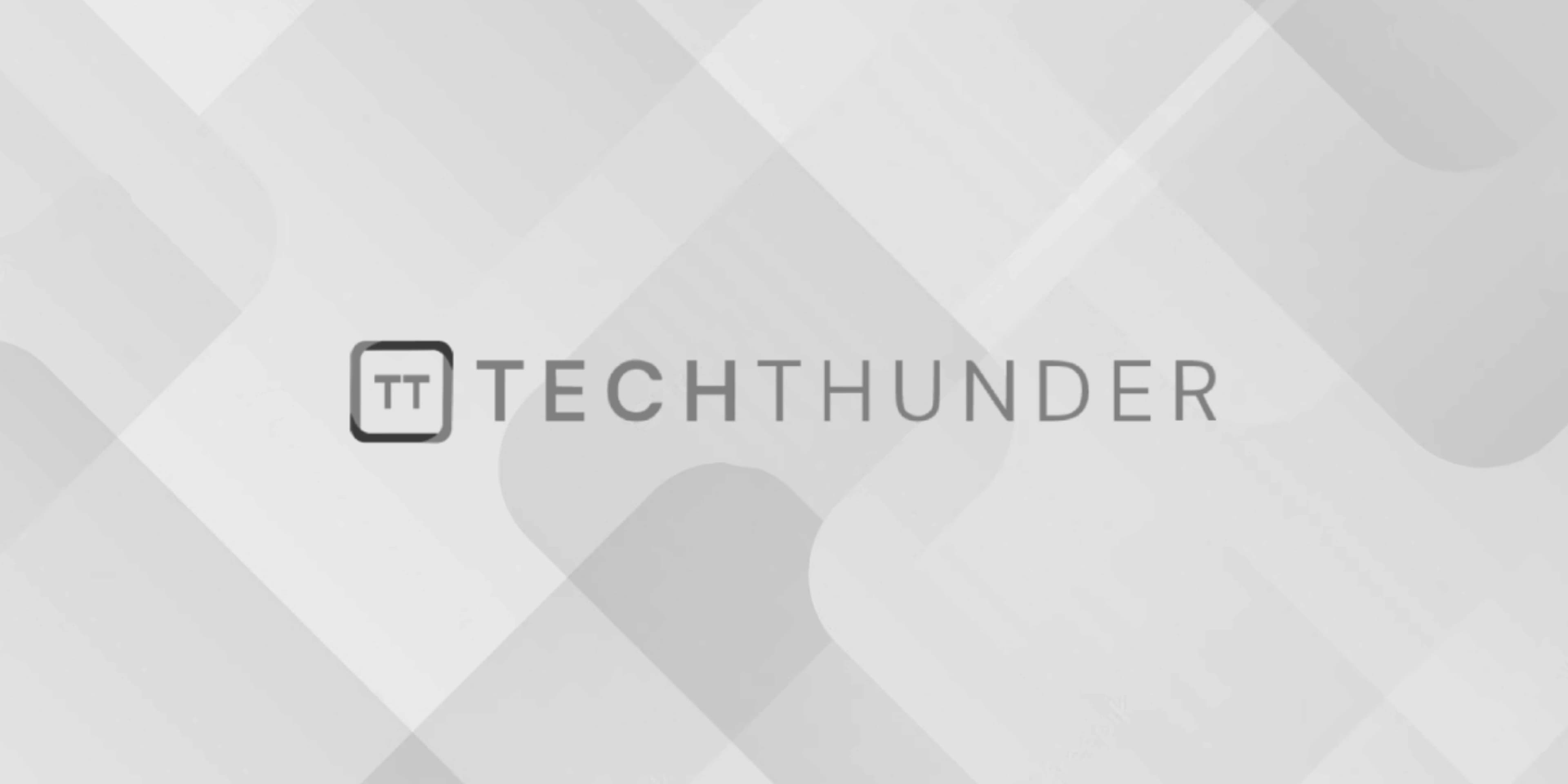
95 views
MySQL UPDATE Record
The MySQL can use the UPDATE
statement to modify existing records in a table. The UPDATE
statement allows you to change the values of one or more columns in existing rows based on a specified condition. Here’s the basic syntax of the UPDATE
statement:
UPDATE table_name
SET column1 = value1, column2 = value2, ...
WHERE condition;
Here’s a breakdown of each part of the UPDATE
statement:
table_name
: This is the name of the table in which you want to update records.SET column1 = value1, column2 = value2, ...
: In this part, you specify the columns you want to update and the new values you want to assign to them.WHERE condition
: This is an optional part that allows you to specify a condition to determine which records should be updated. If you omit theWHERE
clause, all rows in the table will be updated.
Here are some examples of how to use the UPDATE
statement in MySQL:
- Update the salary of an employee with an
employee_id
of 101 to set their new salary to 55000:
UPDATE employees
SET salary = 55000
WHERE employee_id = 101;
- Change the department of all employees with a
department
value of ‘Marketing’ to ‘Sales’:
UPDATE employees
SET department = 'Sales'
WHERE department = 'Marketing';
- Update the
status
of all orders in theorders
table to ‘Shipped’ where the order was placed before a certain date:
UPDATE orders
SET status = 'Shipped'
WHERE order_date < '2023-01-01';
- Increment the
quantity
of all products in theinventory
table by 10%:
UPDATE inventory
SET quantity = quantity * 1.10;
It’s important to use the WHERE
clause carefully to specify the records you want to update. If you omit the WHERE
clause, you’ll update all records in the table, which may not be what you intend.
Before running an UPDATE
statement, ensure that you have the necessary permissions and make sure you have a backup of your data in case any unintended changes occur.