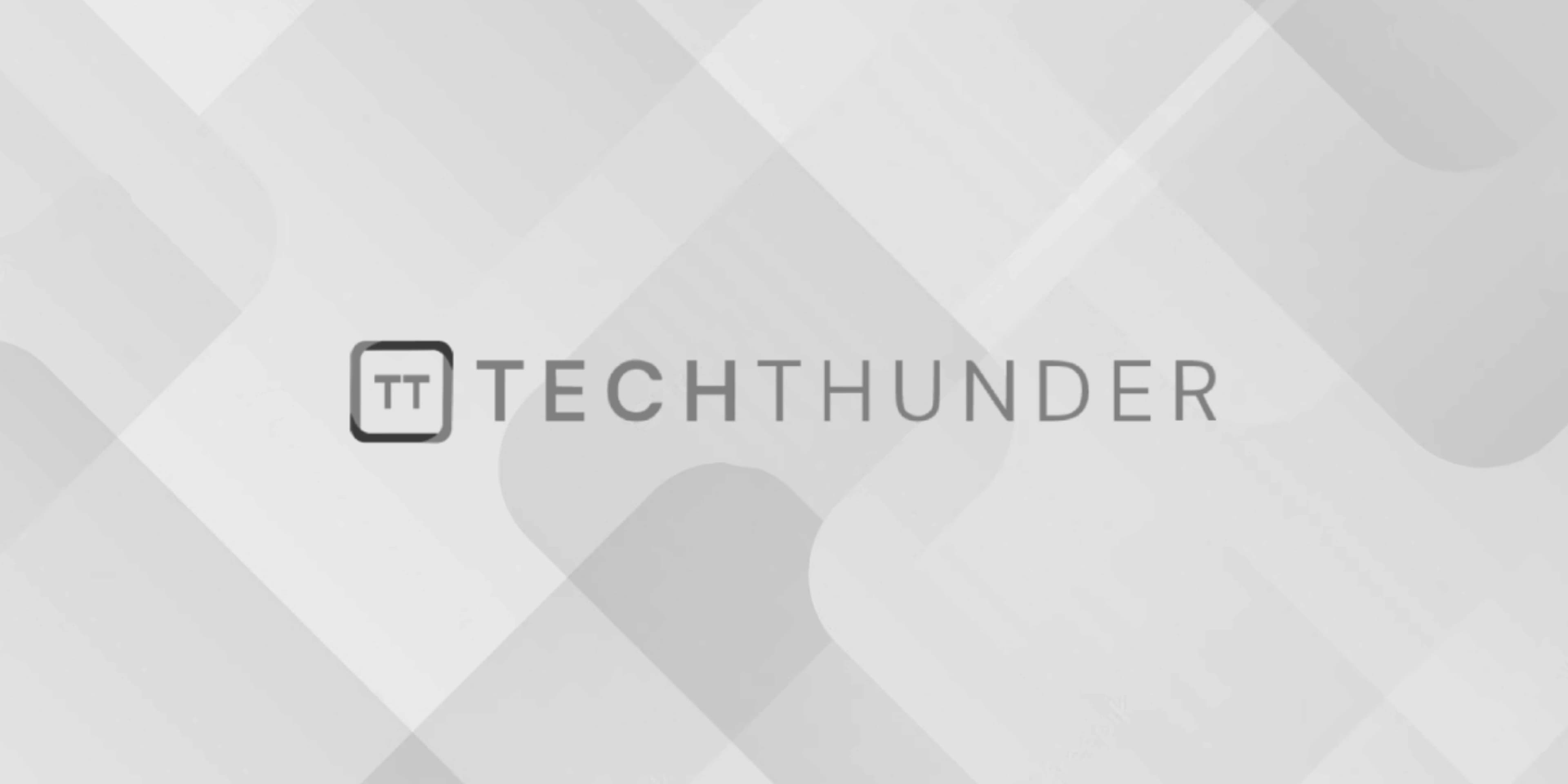
126 views
MySQL JSON
MySQL has built-in support for working with JSON data, allowing you to store, manipulate, and query JSON documents in your database. This feature was introduced in MySQL 5.7 and has been expanded in subsequent versions. Here are some key aspects of working with JSON in MySQL:
- JSON Data Type: MySQL introduces a JSON data type that can be used to store JSON documents. JSON values can be inserted into JSON columns using standard SQL syntax. Example of creating a table with a JSON column:
CREATE TABLE products (
id INT PRIMARY KEY,
name VARCHAR(255),
details JSON
);
- Inserting JSON Data: You can insert JSON data into a JSON column using the
JSON
keyword or by providing a JSON string.
INSERT INTO products (id, name, details)
VALUES (1, 'Product A', JSON_OBJECT('price', 10.99, 'description', 'A nice product'));
- Querying JSON Data: MySQL provides a range of JSON functions for querying and manipulating JSON data. For example, you can use
JSON_EXTRACT()
to extract values from JSON documents.
SELECT name, JSON_EXTRACT(details, '$.price') AS price
FROM products;
- JSON Path Expressions: You can use JSON path expressions in functions like
JSON_EXTRACT()
to navigate the structure of JSON documents. The$
symbol represents the root object.
JSON_EXTRACT(details, '$.description')
- JSON Aggregation Functions: MySQL supports JSON aggregation functions like
JSON_ARRAYAGG()
andJSON_OBJECTAGG()
for aggregating JSON data.
SELECT category, JSON_ARRAYAGG(details) AS product_details
FROM products
GROUP BY category;
- Indexing JSON Data: MySQL allows you to create indexes on JSON columns to improve query performance. You can use functional indexes to index specific JSON expressions.
CREATE INDEX idx_price ON products((details->'$.price'));
- Validating JSON Data: You can use the
IS JSON
condition to check if a string is valid JSON before inserting it into a JSON column.
INSERT INTO products (id, name, details)
VALUES (2, 'Product B', JSON_OBJECT('price', 12.99, 'description', 'Another product', 'image', 'invalid_json'));
-- This insert will fail because 'image' is not valid JSON.
- Modifying JSON Data: MySQL provides functions to add, modify, and delete values within JSON documents, such as
JSON_SET()
,JSON_INSERT()
, andJSON_REMOVE()
.
UPDATE products
SET details = JSON_SET(details, '$.price', 9.99, '$.discount', true)
WHERE id = 1;
- JSON Path Language Support: MySQL supports a subset of the JSON path language standard (JSONPath).
Working with JSON in MySQL is beneficial for scenarios where you need to store and query semi-structured data, such as configuration settings, product details, or user profiles. JSON support allows you to work with data in a flexible and dynamic way. It’s important to check the version of MySQL you are using, as JSON features and functions may vary between versions.