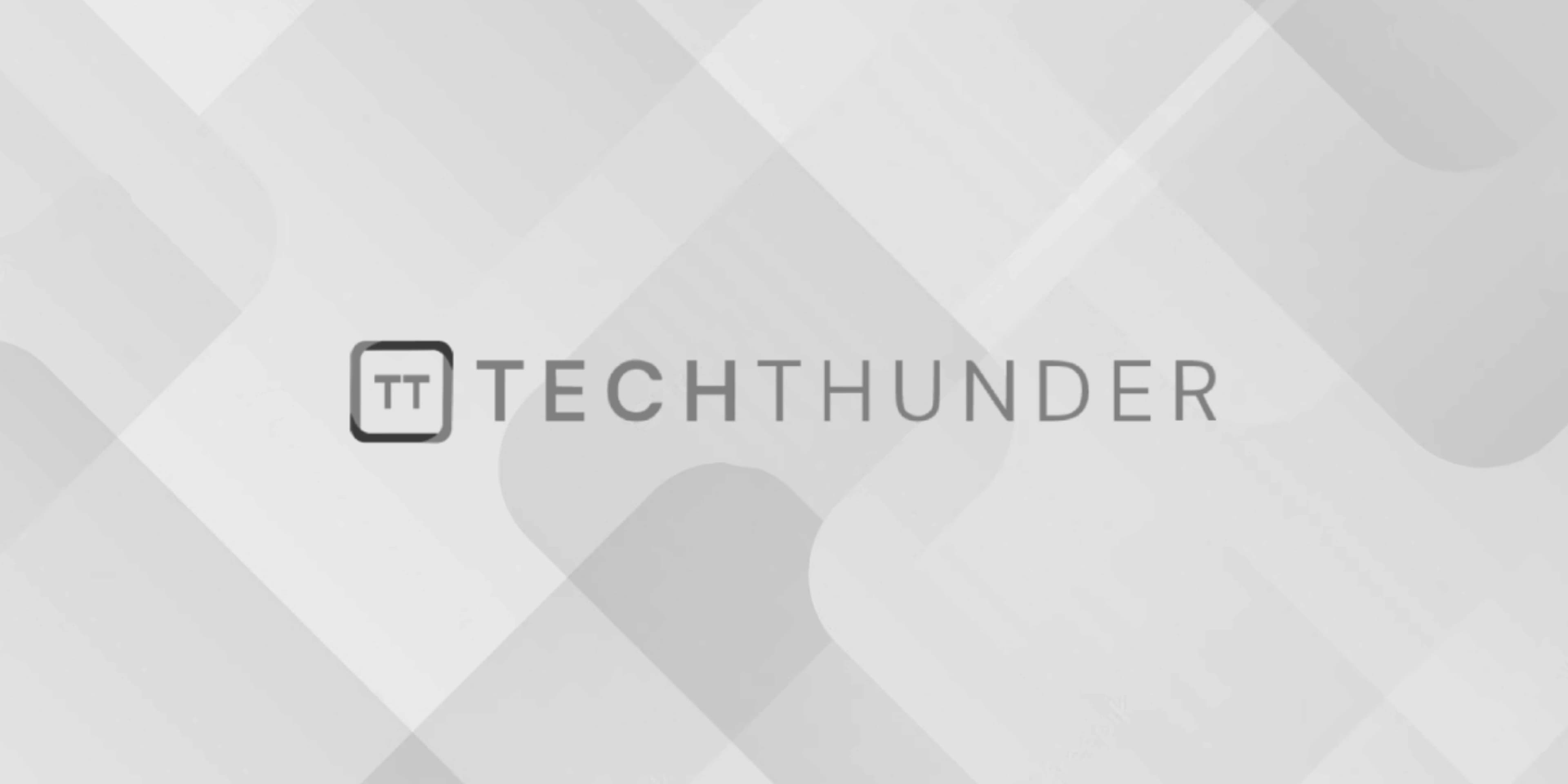
MySQL After Insert Trigger
The AFTER INSERT trigger in MySQL is a type of database trigger that automatically executes after a new row is inserted into a table. It allows you to perform actions or log information after data has been added to a table. Here’s how you can create an “AFTER INSERT” trigger:
DELIMITER //
CREATE TRIGGER after_insert_trigger
AFTER INSERT ON your_table
FOR EACH ROW
BEGIN
-- Trigger logic here
-- You can reference NEW.column_name to access the newly inserted values.
-- You can perform actions like logging, updating other tables, or sending notifications.
END;
//
DELIMITER ;
Let’s break down the components of the “AFTER INSERT” trigger:
after_insert_trigger
: This is the name of your trigger. You should choose a unique name.AFTER INSERT
: Specifies that the trigger should execute after an insert operation on the specified table.your_table
: Replace this with the name of the table on which you want to create the trigger.FOR EACH ROW
: Indicates that the trigger will execute once for each row that is inserted.BEGIN
andEND
: These enclose the trigger logic, where you can include SQL statements that perform actions or logging after the insert operation.
Inside the trigger logic, you can reference the NEW.column_name
to access the values that were just inserted into the table. You can perform various actions, such as logging the data, updating other tables, or sending notifications.
Here’s an example of an “AFTER INSERT” trigger that logs information about newly inserted records into an audit table:
DELIMITER //
CREATE TRIGGER log_after_insert
AFTER INSERT ON orders
FOR EACH ROW
BEGIN
INSERT INTO order_log (order_id, action, action_time)
VALUES (NEW.order_id, 'INSERT', NOW());
END;
//
DELIMITER ;
In this example, the trigger logs the order_id
and the action as ‘INSERT’ along with the current timestamp into an order_log
table each time a new order is inserted.
The AFTER INSERT triggers are useful for post-insert actions, such as data auditing, history tracking, or triggering further events based on the newly inserted data. As with any trigger, make sure that your trigger logic is efficient to avoid performance issues.